In this post, we will see how to enable or disable Wifi in android programmatically.
It is very simple to enable or disable wifi from android code.
For enabling WiFi:
For disabling Wifi:
So you can enable or disable WiFi using WifiManger class.
You need to add extra permissions in AndroidManifest.xml to enable or disable Wifi.
It is very simple to enable or disable wifi from android code.
For enabling WiFi:
1 2 3 4 |
WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(true); |
1 2 3 4 |
WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(false); |
You need to add extra permissions in AndroidManifest.xml to enable or disable Wifi.
Source code:
Step 1 :
Create an android application project named “EnableDisableWiFiApp”.
Step 2:
Add following permissions in AndoridManifest.xml.
1 2 3 4 |
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/> <uses-permission android:name="android.permission.CHANGE_WIFI_STATE"/> |
Go to app -> src -> main -> AndroidManifest.xml and add above permissions. AndroidManifest.xml will look like below xml.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.java2blog.enabledisablewifiapp"> <uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/> <uses-permission android:name="android.permission.CHANGE_WIFI_STATE"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> |
Step 3 :
Change res ->layout -> activity_main.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_margin="16dp" android:gravity="center" android:orientation="vertical"> <ToggleButton android:id="@+id/toggleButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:checked="false" /> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="16dp" /> </LinearLayout> |
Step 4:
Change src/main/packageName/MainActivity.java as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
package com.java2blog.enabledisablewifiapp; import android.content.Context; import android.net.wifi.WifiManager; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.CompoundButton; import android.widget.TextView; import android.widget.ToggleButton; import com.java2blog.enabledisablewifiapp.R; public class MainActivity extends AppCompatActivity { ToggleButton toggleButton; TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Getting toggle button and textView from activity_main toggleButton = (ToggleButton) findViewById(R.id.toggleButton); textView = (TextView) findViewById(R.id.textView); // Put listener on toggle button toggleButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton compoundButton, boolean checked) { if (checked) { textView.setText("WiFi is ON"); WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(true); } else { textView.setText("WiFi is OFF"); WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(false); } } }); // For initial setting if (toggleButton.isChecked()) { textView.setText("WiFi is ON"); WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(true); } else { textView.setText("WiFi is OFF"); WifiManager wifi = (WifiManager) getSystemService(Context.WIFI_SERVICE); wifi.setWifiEnabled(false); } } } |
Step 5:
Run the app
When you run the app, you will get below screen:
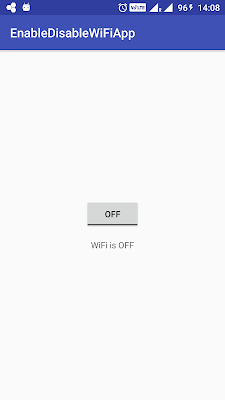
When you click on toggle button, you will get below screen:
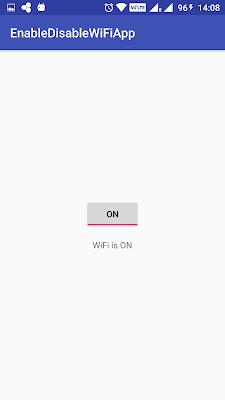
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.