Table of Contents
In this post, we are going to see how to load html String in a WebView in Android
Android WebView is a android UI widget which is used to open any web url or load html data. It is used to show web page in android activity. In simple words, Android WebView is a View that displays web pages.
Example :
Lets say You are creating an android app and you have some user agreement or some web pages hosted online, you can just render it using Android WebView.
There are two methods which we generally use to open web url or load html data.
Android WebView is a android UI widget which is used to open any web url or load html data. It is used to show web page in android activity. In simple words, Android WebView is a View that displays web pages.
Example :
Lets say You are creating an android app and you have some user agreement or some web pages hosted online, you can just render it using Android WebView.
There are two methods which we generally use to open web url or load html data.
- loadUrl
- loadData
I have already demonstrated about loadUrl in previous post. I am going to demonstrate about loadData in this post.
Source code:
Step 1 :Creating Project
Create an android application project named “WebViewLoadDataExampleApp”.
Step 2 : Creating Layout
Change res ->layout -> activity_main.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.java2blog.webviewexampleapp.MainActivity"> <WebView android:id="@+id/webView" android:layout_width="fill_parent" android:layout_height="fill_parent" /> </RelativeLayout> |
Step 3 : Creating MainActivity
Change src/main/packageName/MainActivity.java as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
package com.java2blog.webviewloaddataexampleapp; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.webkit.WebView; import com.java2blog.webviewloaddataexampleapp.R; public class MainActivity extends AppCompatActivity { private WebView webView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); webView = (WebView) findViewById(R.id.webView); String htmlData= "n" + "n" + "n" + "HelloWorldn" + "n" + "n" + " |
Hello World from Java2blog!!
1 2 3 4 |
n" + " |
Android WebView load html data example
1 2 3 4 5 6 7 8 9 |
n"+ "n" + ""; webView.loadData(htmlData, "text/html", "UTF-8"); } } |
We are getting WebView reference from layout file and then using WebView’s loadData method to load the html String in Android WebView.
Step 4 : Running the app
When you run the app, you will get below screen:
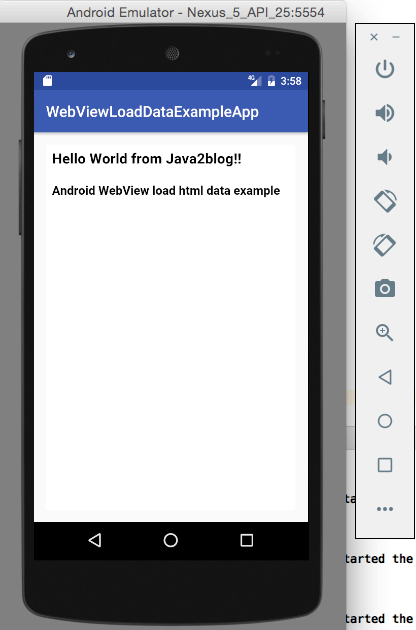
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.