Table of Contents
In this post, we are going to see about Android Spinner Dropdown example.
Android Spinner is a UI widget which have dropdown. Once you click on dropdown , you can select one option among various options. I am going to put static data in android spinner and select one of value from them.
Source code:
Step 1 :Creating Project
Create an android application project named “SpinnerDropdownExampleApp”.
Step 2 : Creating Layout
Change res ->layout -> activity_main.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.java2blog.spinnerdropdownexampleapp.MainActivity"> <TextView android:id="@+id/textview" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Select country:" /> <Spinner android:id="@+id/spinner" android:layout_below="@+id/textview" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </RelativeLayout> |
Step 3 : Creating MainActivity
Change src/main/packageName/MainActivity.java as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
package com.java2blog.spinnerdropdownexampleapp; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.Spinner; import android.widget.Toast; import android.widget.AdapterView.OnItemSelectedListener; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity implements OnItemSelectedListener { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // get Spinner reference Spinner spinner = (Spinner) findViewById(R.id.spinner); // Spinner click listener spinner.setOnItemSelectedListener(this); // Spinner Drop down elements List countries = new ArrayList(); countries.add("India"); countries.add("Nepal"); countries.add("China"); countries.add("Bhutan"); // Creating array adapter for spinner ArrayAdapter dataAdapter = new ArrayAdapter(this, android.R.layout.simple_spinner_item, countries); // Drop down style will be listview with radio button dataAdapter.setDropDownViewResource(android.R.layout.select_dialog_singlechoice); // attaching data adapter to spinner spinner.setAdapter(dataAdapter); } @Override public void onItemSelected(AdapterView parent, View view, int position, long id) { // getting selected item String item = parent.getItemAtPosition(position).toString(); // Showing selected spinner item in toast Toast.makeText(parent.getContext(), "Selected Country: " + item, Toast.LENGTH_LONG).show(); } public void onNothingSelected(AdapterView arg0) { } } |
We have created ArrayAdapter and attached it with Spinner in similar way we have done for Simple Android ListView.
If you notice, we have also implemented OnItemSelectedListener for listening to item selection in drop down.
If you notice, we have also implemented OnItemSelectedListener for listening to item selection in drop down.
Step 4 : Running the app
When you run the app, you will get below screen:
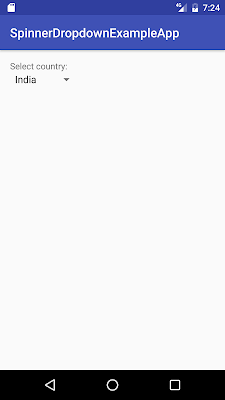
When you click on dropdown and select china, you will get below screen.
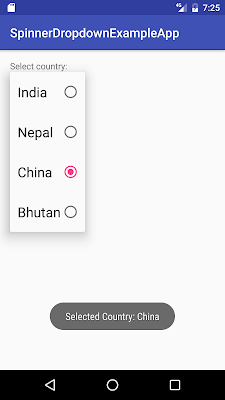
After selecting china, you will get below screen.
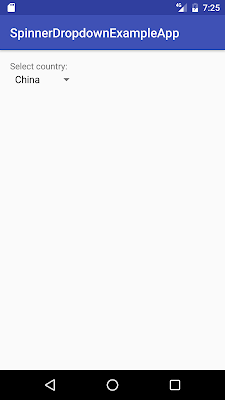
We are done with Android Spinner Dropdown Example. Please comment if you are facing any issue while implementing it.
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.