Table of Contents
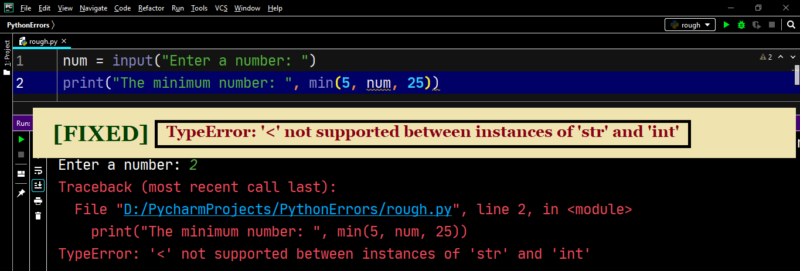
Introduction
Problem: How to fix – TypeError: '<' not supported between instances of 'str' and 'int'
in Python?
Example:
1 2 3 4 5 6 |
num = input("Enter a number: ") print('The minimum number is: ', min(1, 2, 3)) print("The minimum number: ", min(5, num, 25)) |
Output:
Enter a number: 20
The minimum number is: 1
Traceback (most recent call last):
File “D:/PycharmProjects/PythonErrors/rough.py”, line 3, in
print(“The minimum number: “, min(5, num, 25))
TypeError: ‘<‘ not supported between instances of ‘str’ and ‘int’
Explanation:
- In the above example, you can see that when three
integers
were passed to themin
function, then the program gave us the output by fetching the minimum number, i.e.,1
. - But, when we passed a
string
value to themin
function along with two otherintegers
, then it resulted in aType Error: '<' not supported between instances of 'str' and 'int'
.
Hence the TypeError
occurred when we compared two variables whose datatypes are completely different (a string and integer in this case.)
Before proceeding further, let us have a quick look at some of the key concepts that will help you to identify and avoid TypeErrors
in the future.
💡 Python TypeError
TypeError
is one of the most common standard Python exceptions that occur in Python. Python throws aTypeError
when an operation is carried out on an incorrect/unsupported object type.- For example, using the < (less than) operator on an integer and a string value will throw a TypeError.
- This is because integers and strings are two different types of data types and cannot be compared with the help of operators used for comparisons such as <, >, >=, <=, ===, !==.
📖 Read more here: What is TypeError in Python?
📍 The following example will be used to demonstrate the solutions to our mission- critical question:- How to fix TypeError: ‘<‘ not supported between instances of ‘str’ and ‘int’?
Example
The following table lists the tenure and the corresponding Fixed Deposit rates of a certain bank.
Tenure | FD Interest Rates |
6 months to 364 days | 5.25% |
18 months 1 day to 1 year 364 days | 6.00% |
1 year 1 day to 18 months | 5.75% |
2 years to 2 years 364 days | 6.00% |
3 years to 4 years 364 days | 5.65% |
5 years to 10 years | 5.50% |
In the following code, when the user selects a certain tenure the corresponding FD rates shall be displayed. Also, if tenure is greater than 1 year 1 day to 18 months, then customer is a “Prime Customer” else “Normal Customer”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
print(""" Tenure FD 1. 6 months to 364 days 5.25% 2. 18 months 1 day to 1 year 364 days 6.00% 3. 1 year 1 day to 18 months 5.75% 4. 2 years to 2 years 364 days 6.00% 5. 3 years to 4 years 364 days 5.65% 6. 5 years to 10 years 5.50% """) option = input("Select the Tenure (1 - 6): ") if option < 4: print("Normal Customer") if option == 1: print("Rate = ", 5.25) if option == 2: print("Rate = ", 6.00) if option == 3: print("Rate = ", 5.75) else: print("Prime Customer") if option == 4: print("Rate = ", 6.00) if option == 5: print("Rate = ", 5.65) if option == 6: print("Rate = ", 5.50) |
Output:
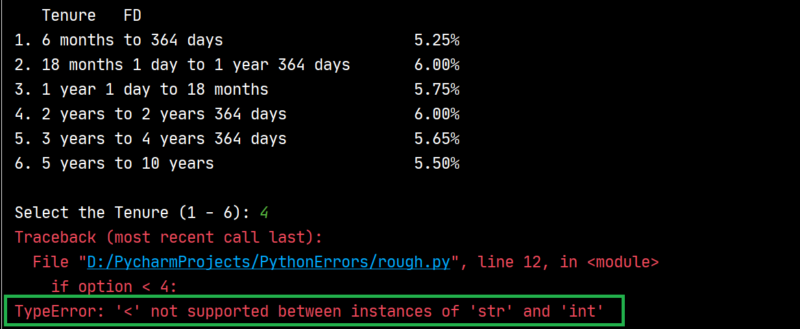
The above error occurred because if option < 4
is an unsupported operation. Let’s solve this issue using the following methods.
📌 Method 1: Using int()
Instead of accepting the user input as a string, you can ensure that the user input is an integer with the help of int()
method.
Solution:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
print(""" 1. 6 months to 364 days 2. 18 months 1 day to 1 year 364 days 3. 1 year 1 day to 18 months 4. 2 years to 2 years 364 days 5. 3 years to 4 years 364 days 6. 5 years to 10 years """) option = int(input("Select the Tenure (1 - 6): ")) if option < 4: print("Normal Customer") if option == 1: print("Rate = 5.25%") if option == 2: print("Rate = 6.00%") if option == 3: print("Rate = 5.75%") else: print("Prime Customer") if option == 4: print("Rate = 6.00%") if option == 5: print("Rate = 5.65%") if option == 6: print("Rate = 5.50%") |
Output:
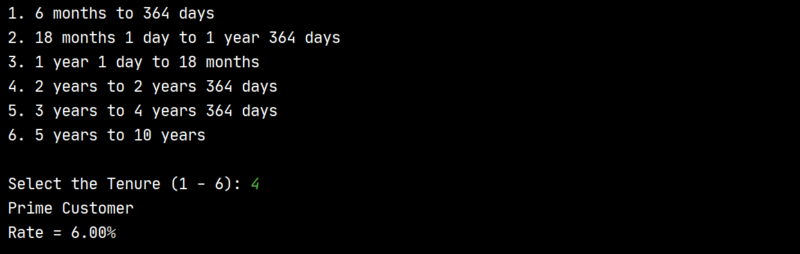
Explanation: int(value)
function converts the user-input to an integer value. Thus now you can compare two values of the same data type using the <
operator.
📌 Method 2: Using a Custom Function
Instead of manually typecasting the string value to an integer you can also define a function to convert it to a string.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
def convert(x, cast): x = cast(x) return x print(""" 1. 6 months to 364 days 2. 18 months 1 day to 1 year 364 days 3. 1 year 1 day to 18 months 4. 2 years to 2 years 364 days 5. 3 years to 4 years 364 days 6. 5 years to 10 years """) def fd(option): if int(option) < 4: print("Normal Customer") if option == 1: print("Rate = 5.25%") if option == 2: print("Rate = 6.00%") if option == 3: print("Rate = 5.75%") else: print("Prime Customer") if option == 4: print("Rate = 6.00%") if option == 5: print("Rate = 5.65%") if option == 6: print("Rate = 5.50%") choice = input("Select the Tenure (1 - 6): ") x = convert(choice, int) fd(x) |
Output:
2. 18 months 1 day to 1 year 364 days
3. 1 year 1 day to 18 months
4. 2 years to 2 years 364 days
5. 3 years to 4 years 364 days
6. 5 years to 10 years
Select the Tenure (1 – 6): 5
Prime Customer
Rate = 5.65%
🐰 Bonus Read: Python Typecasting
Type Casting is the process of converting a literal of one type to another type. Typecasting is also known as explicit type conversion. Python inbuilt functions like int()
, float()
and str()
are used for typecasting.
For example:
int()
function can accept afloat
orstring
literal as an input and return a value ofint
type.
1 2 3 4 5 6 7 8 9 10 11 12 |
li = ['50', '50.25', 100] my_int = int(li[0]) # typecasting str to int my_float = float(li[1]) # typecasting str to float my_str = str(li[2]) # typecasting float to str print("""Original Typecasted {0} {1} {2} {3} {4} {5} """.format(type(li[0]), type(my_int), type(li[1]), type(my_float), type(li[2]), type(my_str))) |
Output:
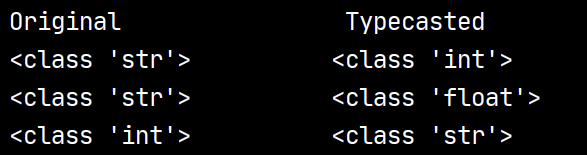
Conclusion
Hence, in this article, you learned what is Type Error: '<' not supported between instances of 'str' and 'int'
and the possible causes and solutions of the error.
In general, if you are getting a TypeError
in your code, take a moment to verify that the data you are operating on is actually of the type you would expect it to be.
You can rectify this error by manually converting the datatype so that the comparison is performed between values of the same datatypes.
Recommended Tutorials:
- [Solved] TypeError: Can only Concatenate str (not “int”) to str
- [Solved] TypeError: not all arguments converted during string formatting
- [Solved] TypeError: Can’t Multiply Sequence by non-int of Type ‘float’ In Python?
Please subscribe and stay tuned for more tutorials. Happy learning! 📚