Table of Contents
- ❖ What is “TypeError” in Python?
- ❖ What does TypeError: can only concatenate str (not “int”) to str mean ?
- ◈ Solution 1: Use Same Data Types
- ◈ Solution 2: Typecasting The Data Types to Concatenate/Add Similar Types
- ◈ Solution 3: Using The format() Function
- ◈ Solution 4: Using The join() and str() Function Together
- ◈ Solution 5: Use Function
- Conclusion
Summary: To eliminate the TypeError - can only concatenate str (not "int") to str
typecast all the values into a single type.
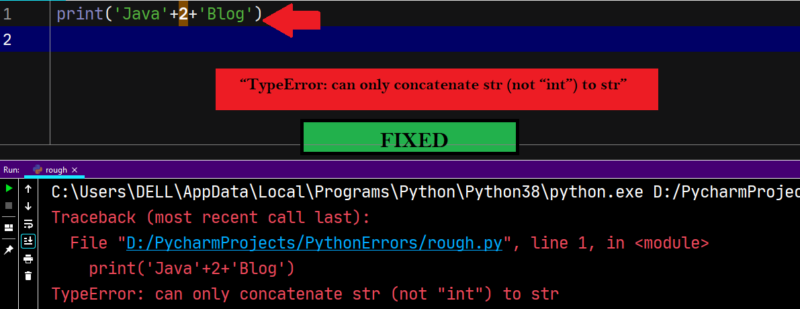
Problem Formulation: How to fix Typeerror: can only concatenate str (not "int") to str
in Python?
Example:
1 2 3 4 |
print('10'+5) # expected output: 15 print('Java' + '2' + 'Blog') # expected output: Java2Blog |
Output:
Traceback (most recent call last):
File “main.py”, line 1, in
print(’10’+5) # expected output: 15
TypeError: can only concatenate str (not “int”) to str
❖ What is “TypeError” in Python?
In Python, a TypeError occurs when you perform an incorrect function call or use an incorrect operator type. For instance, let’s see what happens when we include two inconsistent types:
Example:
1 2 3 4 |
var = 30 print(var[1]) |
Output:
Traceback (most recent call last):
File “D:/PycharmProjects/PythonErrors/rough.py”, line 2, in
print(var[1])
TypeError: ‘int’ object is not subscriptable
Python throws a TypeError
because int
type objects are not accessible by using the index. To access an element using its index- you must create a list, tuple, or string type objects.
❖ What does TypeError: can only concatenate str (not “int”) to str mean ?
To understand this Typeerror
, let’s consider the following example:
1 2 3 4 5 6 7 |
print(3 + 5) # adding two integers print('john' + 'monty') # concatenating two strings print('green ' * 3) # multiplying an int and a string print('Java' + 2 + 'Blog') # Concatenating two strings and an integer |
Output:
8
johnmonty
green green green
Traceback (most recent call last):
File “main.py”, line 4, in
print(‘Java’ + 2 + ‘Blog’) # Concatenating two strings and an integer
TypeError: can only concatenate str (not “int”) to str
The first three lines of code work fine because the concatenation between the same types and multiplication between an integer and a string is allowed in Python.
✨ As Python is a dynamic programming language, the first three lines of code are executed, and then Python throws TypeError: can only concatenate str (not "int") to str
because concatenating a string and a numeric value (int) is not allowed in Python.
Now that we know the reason behind the occurrence of this error, let’s dive into the solutions:
◈ Solution 1: Use Same Data Types
Instead of concatenating an integer and a string, you can locate the line within your code causing the error. Then rectify it to ensure that you concatenate two values of the same type, i.e., both the values are strings.
You can easily identify and eliminate the TypeError
by utilizing an IDE that checks for errors. If you don’t have any idea where the bug is and you are not utilizing the IDE, it is beneficial to download one as it makes it simpler to discover the bug in the code.

The Solution:
1 2 3 4 |
print(10 + 5) # expected output: 15 print('Java' + '2' + 'Blog') # expected output: Java2Blog |
Output:
15
Java2Blog
In any case, this method is incapable of more complex codes since you do it all manually! 😉
◈ Solution 2: Typecasting The Data Types to Concatenate/Add Similar Types
Discover in which line of code TypeError
showed up and then typecast the values to represent the same type.
- When you want to add two numbers, you have to typecast both values to ‘
int
‘. - When you want to concatenate two texts, typecast them to ‘
str
‘.
1 2 3 4 |
print(int('10') + 5) # expected output: 15 print('Java' + str(2) + 'Blog') # expected output: Java2Blog |
Output:
15
Java2Blog
⚠️ Caution: This method isn’t ideal when you have a ton of data that you need to combine because it is a cumbersome task to manually typecast every variable one by one.
◈ Solution 3: Using The format() Function
❖ The format()
function is used in Python for String Concatenation. This function joins various elements inside a string through positional formatting.
Example:
1 2 3 4 5 6 |
a = "John" b = 13 print("{} {}".format(a, b)) |
Output:
John13
The braces (“{}”) are utilized here to fix the string position. The variable ‘a’ is stored in the first brace, and the second variable ‘b’ gets stored in the second curly brace. The format()
function combines the strings stored in both variables “a” and “b” and displays the concatenated string.
◈ Solution 4: Using The join() and str() Function Together
The join()
method is the most adaptable method of concatenating strings in Python. If you have numerous strings and you need to combine them, use the join()
function. The most interesting thing about join()
is that you can concatenate strings utilizing a separator. Hence, it also works at iterators like tuples, strings, lists, and dictionaries.
To combine integers into a single string, apply the str()
function to every element in the list and then combine them with the join() as shown below.
1 2 3 4 5 |
a = [0, 1, 2] x = '-'.join ((str(n) for n in a)) print(x) |
Output:
0-1-2
◈ Solution 5: Use Function
Another approach to resolve our problem is to create a convert function that converts a variable from one type to another.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
def convert(a, b, type_): a = type_(a) b = type_(b) return a, b x, y = convert('Python', 3.18, str) print('First: Converting To String') print(type(x)) print(type(y)) print("Output: ", x + " " + y) a, b = convert('10', 5, int) print('\n Second: Converting To Integer') print(type(a)) print(type(b)) print("Output: ", a + b) |
Output:
First: Converting To String
<class ‘str>’
<class ‘str’>
Output: Python 3.18
Second: Converting To Integer
<class ‘int’>
<class ‘int’>
Output: 15
If there are more than two variables that you need to convert using a list as shown below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
def convert(list1, type_): for x in range(len(list1)): list1[x] = type_(list1[x]) a = 20 b = '25' c = '43' list2 = [a, b, c] print('\n String values:') convert(list2, str) print([type(number) for number in list2]) print(list2) print('\n Integer values:') convert(list2, int) print([type(number) for number in list2]) print(list2) print(list2[0] + list2[1]) |
Output:
String values:
[, , ]
[’20’, ’25’, ’43’]
Integer values:
[, , ]
[20, 25, 43]
45
Conclusion
I hope you found this article helpful. Please stay tuned and subscribe for more interesting articles! 📚
This article was submitted by Rashi Agarwal and edited by Shubham Sayon.