Table of Contents
Problem Formulation: How to sort a given string alphabetically in Python?
Example:
- Input String: PYTHON
- Output String: HNOPTY
Thus, in this article, you will learn about numerous ways to sort and arrange a string alphabetically in Python, as shown in the above example.
✨ Method 1: Using sorted() + join() Methods
📍 sorted()
is a function in Python that sorts all the elements of an iterable in a specific order and returns a sorted list of the specified iterable. You can sort the items in ascending or descending order.
Syntax:
sorted(iterable, key=key, reverse=reverse)
✏️ Note: You cannot sort an iterable that contains both – numerical and string values.
📍 join()
is a method in Python that takes all the items in an iterable and then combines them in one string. You must specify a string as a separator.
Syntax:
string.join(iterable)
Now, let’s use the sorted()
and join()
methods to sort the given string alphabetically.
1 2 3 4 5 6 7 8 9 |
text = 'PYTHON' # printing both strings print("Original String-", text) # sorting the string alphabetically print("Sorted String-", ''.join(sorted(text))) |
Output:
Original String- PYTHON
Sorted String- HNOPTY
Explanation:
sorted(text)
helped us to sort the given characters of the string in alphabetical order.join()
helped us to combine the sorted characters into one string.
The above example sorts the string in ascending order. You can also sort it in descending order using the following syntax:
print(“Sorted String-“, ”.join(sorted(text, reverse=True)))
reverse
is a Boolean attribute of thesorted()
method that generates a sorted output in descending order when it is set toTrue
.
☠️ Caution: Always remember to use the join()
method after sorting the strings. Forgetting to do so will result in an output of a sorted list instead of a string, as shown below.
1 2 3 4 5 6 |
text = 'PYTHON' print("Original String-", text) print("String 1-", sorted(text)) |
Output:
Original String- PYTHON
String 1- [‘H’, ‘N’, ‘O’, ‘P’, ‘T’, ‘Y’]
✏️ You can also use the set()
method to only display unique letters in the sorted string. In Python, sets are an unordered collection of the unique elements. Thus, it eliminates all the duplicate values.
Example:
1 2 3 4 5 6 |
text = 'consequences' print("Original String: ", text) print("Sorted String: ", ''.join(sorted(set(text)))) |
Output:
Original String: consequences
Sorted String: cenoqsu
💡 How will you sort a string that contains both – uppercase and lowercase characters?
Solution: You can use to a lambda function within the sorted method to achieve this task.
1 2 3 4 5 |
text = 'MiSsIsSiPpI' print("".join(sorted(text, key=lambda a: a.lower()))) |
Output:
iIiIMPpSssS
✨ Method 2: Convert The String to List And Sort
In Python, strings are immutable, and lists are mutable.
Hence, we can sort the string by converting it into a list first. We will then use a sorting algorithm (insertion sort or bubble sort) to sort the converted list. Finally, we will convert the sorted list to a string and print the output.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
# Take user input or enter the value of strings manually inside the code my_str = input("Enter a string: ") # Creating an empty list lst = list() # Calculating the length of the string length = len(my_str) # Converting the string to list for x in my_str: lst.append(x) # Sorting the list using bubble sort for i in range(length - 1): for j in range(length - i - 1): if lst[j] > lst[j + 1]: lst[j], lst[j + 1] = lst[j + 1], lst[j] # Converting the sorted list into string sorted_str = "" for m in lst: sorted_str = sorted_str + m # Printing the Sorted string print("The sorted string is: ", sorted_str) |
Output:
Enter a string: PYTHON
The sorted string is: HNOPTY
✨ Method 3: Using The reduce() Method
reduce()
is function of thefunctools
module in Python.- It takes a function and a list as an argument.
- When it is called with a
lambda
function and an iterable, it performs a repetitive operation over the pairs of the iterable.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
# Importing reduce() function from functools import reduce # Take user input or enter the value of strings manually inside the code text = input("Enter a String: ") text2 = "InputString" print("String 2: ", text2) # Sorting the strings alphabetically sorted_str = reduce(lambda a, b: a + b, sorted(text.lower())) sorted_str2 = reduce(lambda a, b: a + b, sorted(text2.lower())) # Printing the Sorted strings print("Sorted String 1: ", sorted_str) print("Sorted String 2: ", sorted_str2) |
Output:
Enter a String: Python
String 2: InputString
Sorted String 1: hnopty
Sorted String 2: giinnprsttu
Explanation:
- To use the
reduce
method, you have to import it from thefunctools
module. sorted(text.lower())
–> converts all the characters to lowercase and then sorts them alphabetically in a list.- the reduce function then accepts the lambda function and the list containing sorted characters of the string as inputs. It then applies the join function on the sorted list with the help of
+
operator.
✨ Method 4: Using The accumulate() Function
✏️ accumulate()
is a function of the itertools
module in Python. It takes two arguments as an input, i.e., an iterable and a function that will be implemented at each iteration.
#Note: If no function parameter is mentioned, then by default accumulate
will perform an addition operation.
Syntax:
itertools.accumulate(iterable[, [func])
You can use the accumulate function to sort the given string as shown in the following example:
1 2 3 4 5 6 7 |
# import the accumulate function from itertools module from itertools import accumulate text = 'PYTHON' # generate sorted string using accumulate print(tuple(accumulate(sorted(text)))[-1]) |
Output:
HNOPTY
Explanation:
- To be able to use the
accumulate
function, you have to import it from theitertools
module. sorted(text)
will sort the string in alphabetical order.- Now let’s visualize what happens in each iteration within the accumulate function when you store the result of
accumulate(sorted(text))
in a list:
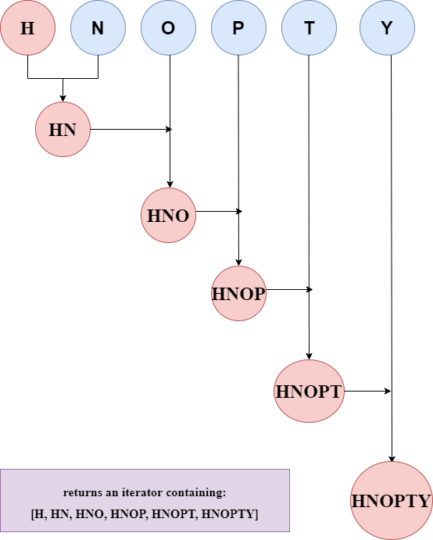
- Finally, select the last index of the list to print the output –>
li[-1]
Read here: Python String Compare
Conclusion
I hope this article managed to guide you through all the methods that can be used to sort a string alphabetically in Python. Please stay tuned and subscribe for more tutorials.
Happy learning! 📚
Author: Shubham Sayon
Co-author: Rashi Agarwal