Table of Contents
If you want to practice data structure and algorithm programs, you can go through 100+ java coding interview questions.
1. Introduction
This article provides a detailed exploration of the Level Order traversal technique in binary trees, focusing on its implementation in Java.
2. What is Level Order Traversal?
Level Order traversal involves visiting all nodes at same level before moving to next level. This means traversing binary tree level by level.
Level order traversal of below binary tree will be:
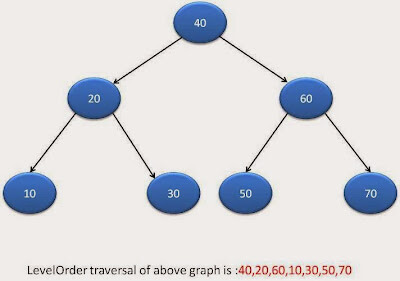
We will use Queue for Level Order traversal. This algorithm is very similar to Breadth first search of graph.
3. Process of Level Order Traversal
- Create empty
queue
and pustroot
node to it. - Do the following when
queue
is not emptyPop
a node fromqueue
and print itPush left child
of popped node toqueue
if not nullPush right child
of popped node toqueue
if not null
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
// prints in level order public static void levelOrderTraversal(TreeNode startNode) { Queue<TreeNode> queue=new LinkedList<TreeNode>(); queue.add(startNode); while(!queue.isEmpty()) { TreeNode tempNode=queue.poll(); System.out.printf("%d ",tempNode.data); if(tempNode.left!=null) queue.add(tempNode.left); if(tempNode.right!=null) queue.add(tempNode.right); } } |
4. Complete Java Program
Lets say our binary tree is :
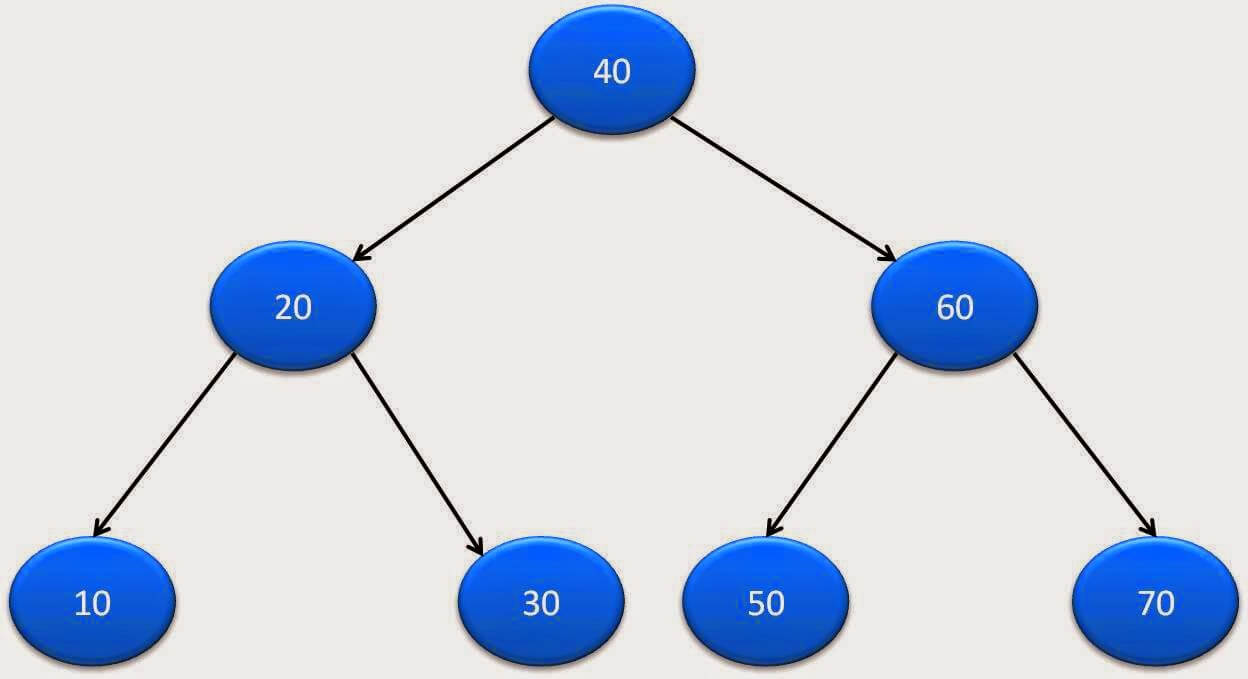
So Level Order traversal will work as below:
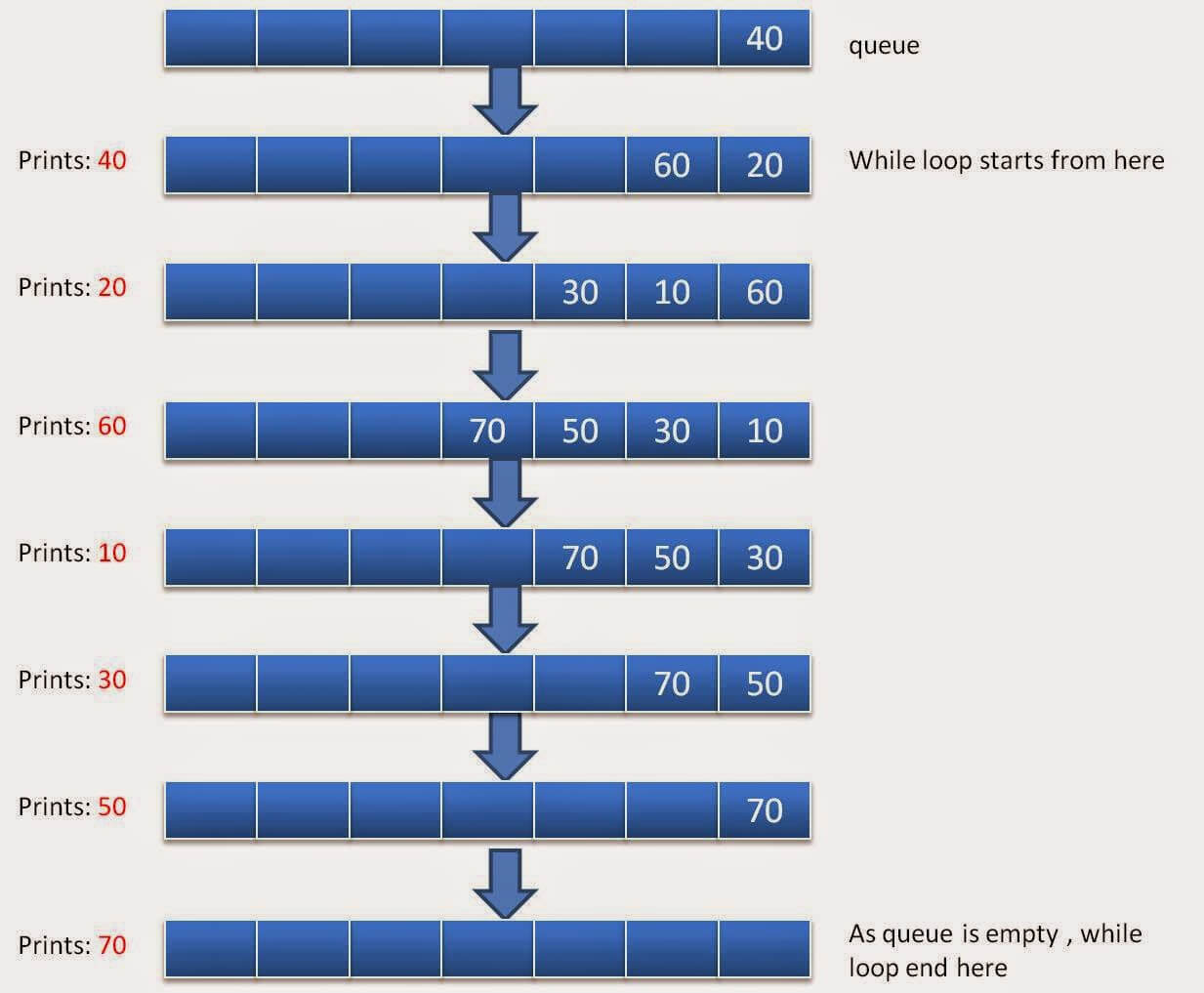
Here is java program for level order traversal:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
import java.util.Queue; import java.util.LinkedList; public class BinaryTreeLevelOrder { public static class TreeNode { int data; TreeNode left; TreeNode right; TreeNode(int data) { this.data=data; } } // prints in level order public static void levelOrderTraversal(TreeNode startNode) { Queue<TreeNode> queue=new LinkedList<TreeNode>(); queue.add(startNode); while(!queue.isEmpty()) { TreeNode tempNode=queue.poll(); System.out.printf("%d ",tempNode.data); if(tempNode.left!=null) queue.add(tempNode.left); if(tempNode.right!=null) queue.add(tempNode.right); } } public static void main(String[] args) { // Creating a binary tree TreeNode rootNode=createBinaryTree(); System.out.println("Level Order traversal of binary tree will be:"); levelOrderTraversal(rootNode); } public static TreeNode createBinaryTree() { TreeNode rootNode =new TreeNode(40); TreeNode node20=new TreeNode(20); TreeNode node10=new TreeNode(10); TreeNode node30=new TreeNode(30); TreeNode node60=new TreeNode(60); TreeNode node50=new TreeNode(50); TreeNode node70=new TreeNode(70); rootNode.left=node20; rootNode.right=node60; node20.left=node10; node20.right=node30; node60.left=node50; node60.right=node70; return rootNode; } } |
Run above program and you will get following output:
40 20 60 10 30 50 70
5. Complexity of Algorithm
The time complexity of Level Order traversal is o(n) where n is number of nodes in binary tree. This is because each node is visited exactly once during level order traversal.
The space complexity of Level Order traversal is o(w) where w is maximum width in binary tree. This is because maximum number of nodes in the queue is proportional to the width of binary tree at any point in time.
6. Conclusion
In this article, we covered about Binary tree Level Order traversal and its implementation. We have done traversal using similar to breadth first search. We also discussed about time and space complexity for the traversal.
Java Binary tree tutorial
- Binary tree in java
- Binary tree preorder traversal
- Binary tree postorder traversal
- Binary tree inorder traversal
- Binary tree level order traversal
- Binary tree spiral order traversal
- Binary tree reverse level order traversal
- Binary tree boundary traversal
- Print leaf nodes of binary tree
- Count leaf nodes in binary tree
- get maximum element in binary tree
- Print all paths from root to leaf in binary tree
- Print vertical sum of binary tree in java
- Get level of node in binary tree in java
- Lowest common ancestor(LCA) in binary tree in java
Please go through java interview programs for more such programs.
You have a typo after explain how Level Order traversal works on “Lets create java program for PreOrder traversal:” it is not PreOrder, anyway everything is well explained and is easy to see that is a typo.
Really good explanation
Good