Table of Contents
There are two ways to use interceptor with Spring MVC.
Spring MVC tutorial:
- Spring MVC hello world example
- Spring MVC Hibernate MySQL example
- Spring MVC interceptor example
- Spring MVC angularjs example
- Spring MVC @RequestMapping example
- Spring Component,Service, Repository and Controller example
- Spring MVC @ModelAttribute annotation example
- Spring MVC @RestController annotation example
- Spring MultiActionController Example
- Spring MVC ModelMapSpring MVC file upload example
- Spring restful web service example
- Spring restful web service json example
- Spring Restful web services CRUD example
- Spring security hello world example
- Spring security custom login form example
HandlerInterceptor Interface :
We can implement HandlerInterceptor interface to use interceptor. It has three methods which we need to implement.
HandlerInterceptorAdapter class:
Issue with HandlerInterceptor is that you need to implement all the methods but if you extend to [abstract class](https://java2blog.com/abstract-class-java/ “abstract class”) called HandlerInterceptorAdapter, you can implement only those method which you want and other methods will have default implementation.
Lets implement custom interceptor example using HandlerInterceptorAdapter class.
Spring MVC interceptor example:
I am using Spring MVC hello world example here with small changes to it.
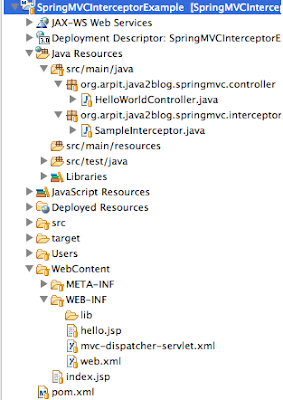
Adding Spring MVC dependency
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringMVCHelloWorldExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringMVCHelloWorldExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> </dependencies> <build> <finalName>SpringMVCHelloWorldExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <jdk.version>1.7</jdk.version> </properties> </project> |
Create ControllerÂ
Create a package named “org.arpit.java2blog.springmvc.controller”
create a controller class named “HelloWorldController.java”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
package org.arpit.java2blog.springmvc.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.servlet.ModelAndView; @Controller public class HelloWorldController { @RequestMapping("/helloworld") public ModelAndView hello() { return new ModelAndView("hello"); } } |
Create interceptorÂ
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
package org.arpit.java2blog.springmvc.interceptor; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.springframework.web.servlet.ModelAndView; import org.springframework.web.servlet.handler.HandlerInterceptorAdapter; public class SampleInterceptor extends HandlerInterceptorAdapter { @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { request.setAttribute("blogName", "java2blog"); return true; } @Override public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView) throws Exception { String blogName = (String) request.getAttribute("blogName"); // We are adding some modelAndView objects here and will use it in view jsp. modelAndView.addObject("blogName",blogName); modelAndView.addObject("authorName", "Arpit"); } @Override public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) throws Exception { String blogName = (String) request.getAttribute("blogName"); String authorName = (String) request.getAttribute("authorName"); System.out.println("Request URL::" + request.getRequestURL().toString()); System.out.println("Blog name : " + blogName); System.out.println("Author Name : " + authorName); } } |
Here I am setting request attribute(blogName) in preHandle method and we are getting it in postHandle method. We are also adding two objects to modelAndView which we will use in jsp.
Create view
Modify index.jsp as below
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>HelloWorld</title> </head> <body> <a href="helloworld.html">Click here to read hello message </a> </body> </html> |
Create hello.jsp in /WEB-INF/ folder
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Hello</title> </head> <body> This is written by ${authorName} on ${blogName} </body> </html> |
Configuring spring mvc application
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>Archetype Created Web Application</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>springmvc-dispatcher</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springmvc-dispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> |
create xml file named “springmvc-dispatcher-servlet.xml” in /WEB-INF folder as below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<beans:beans xmlns="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <context:component-scan base-package="org.arpit.java2blog.springmvc.controller" /> <beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <beans:property name="prefix" value="/WEB-INF/"/> <beans:property name="suffix" value=".jsp" /> </beans:bean> <interceptors> <interceptor> <mapping path="/helloworld.html" /> <beans:bean class="org.arpit.java2blog.springmvc.interceptor.SampleInterceptor"></beans:bean> </interceptor> </interceptors> </beans:beans> |
create xml file named “springmvc-dispatcher-servlet.xml” in /WEB-INF folder as below.
1 2 3 4 5 6 7 |
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> |
It ‘s time to do maven build.
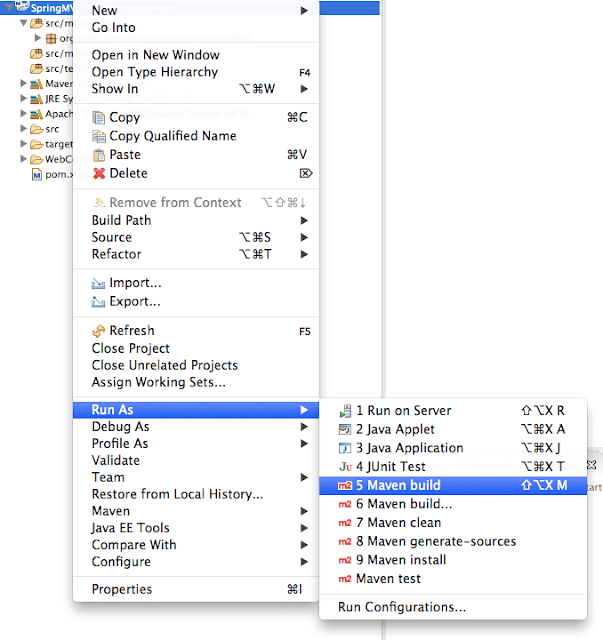
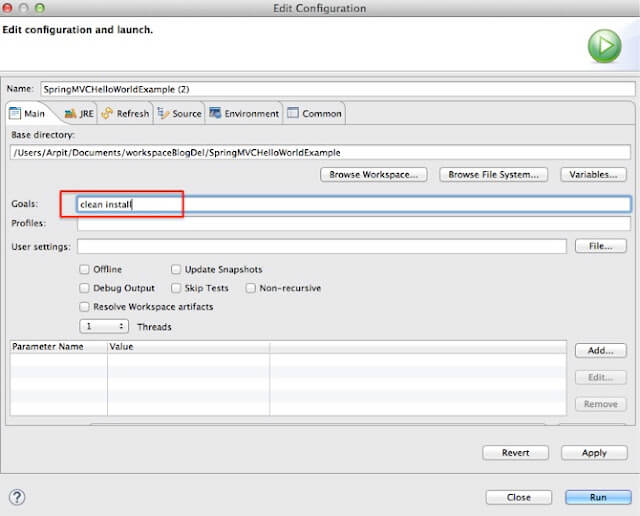
Run the application
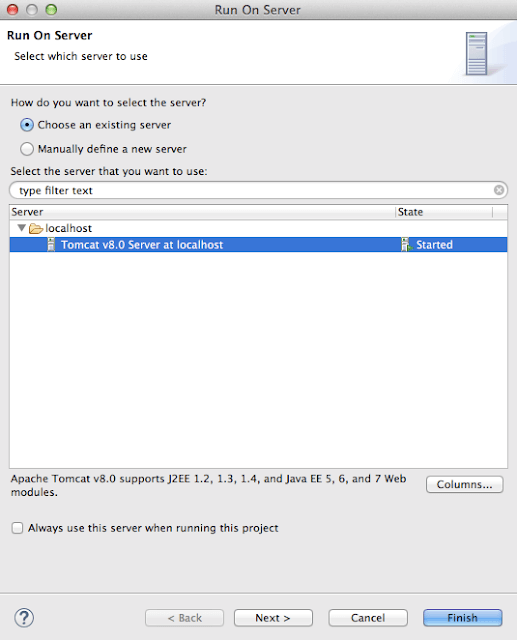
 You will see below screen:
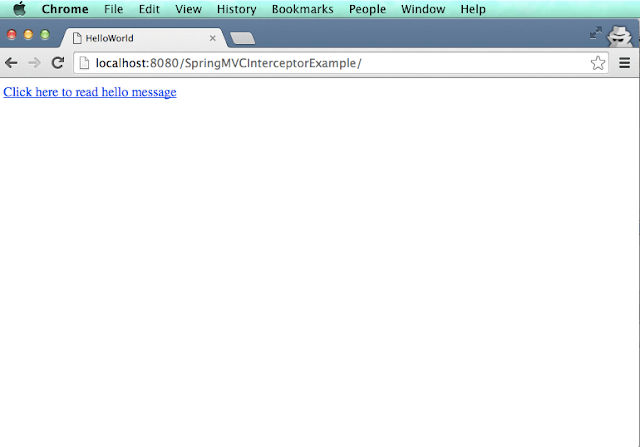
When you click on above link, you will get below screen
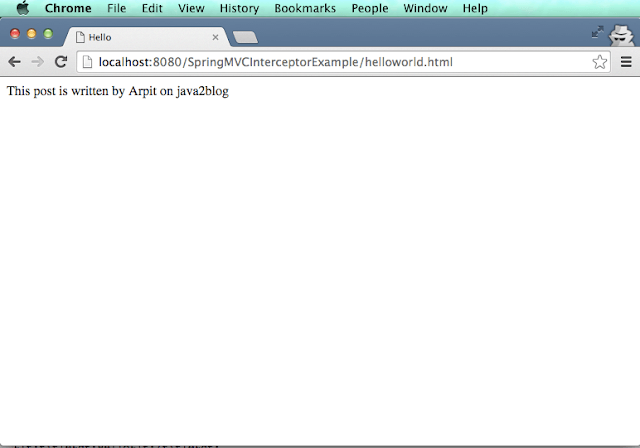
We are done with Spring MVC interceptor example. If you are still facing any issue, please comment.
click to begin20KB .zip