Table of Contents
We have already seen integration of Spring Rest with hibernate in previous tutorial.
Spring MVC Tutorial
- Spring MVC hello world example
- Spring MVC Hibernate MySQL example
- Spring MVC Spring Data example
- Spring MVC Interceptor example
- Spring MVC angularjs example
- Spring MVC @RequestMapping example
- Spring Component,Service, Repository and Controller example
- Spring MVC @ModelAttribute annotation example
- Spring MVC @RestController annotation example
- Spring MultiActionController Example
- Spring MVC ModelMap
- Spring MVC file upload example
- Spring MVC Exceptional handling using @ControllerAdvice
- Spring MVC Exceptional handling using @ExceptionalHandler
- Spring restful web service example
- Spring restful web service json example
- Spring Restful web services CRUD example
- Spring security hello world example
- Spring security custom login form example
Here are steps to create a project with Spring MVC , hibernate and mySQL crud example.
Github Source code:
Maven dependencies
2) We are using Spring 4 and Hibernate 4 for this application. It should work with hibernate 3 also but we need to do small changes in hibernate configuration.
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringMVCHibernateCRUDExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringMVCHibernateCRUDExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> <!-- Hibernate --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>${hibernate.version}</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${hibernate.version}</version> </dependency> <!-- Apache Commons DBCP --> <dependency> <groupId>commons-dbcp</groupId> <artifactId>commons-dbcp</artifactId> <version>1.4</version> </dependency> <!-- Spring ORM --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>${spring.version}</version> </dependency> <!-- AspectJ --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>${org.aspectj-version}</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.6</version> </dependency> <!-- https://mvnrepository.com/artifact/jstl/jstl --> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> </dependencies> <build> <finalName>SpringMVCHibernateCRUDExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <configuration> <failOnMissingWebXml>false</failOnMissingWebXml> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <security.version>4.0.3.RELEASE</security.version> <jdk.version>1.7</jdk.version> <hibernate.version>4.3.5.Final</hibernate.version> <org.aspectj-version>1.7.4</org.aspectj-version> </properties> </project> |
Spring application configuration:
3)Â Change web.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>Archetype Created Web Application</display-name> <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/applicationcontext.xml</param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <servlet> <servlet-name>spring</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>spring</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> |
4)Â create a xml file named spring-servlet.xml in /WEB-INF/ folder.
Please change context:component-scan if you want to use different package for spring to search for controller.Please refer to spring mvc hello world example for more understanding.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
<?xml version="1.0" encoding="UTF-8"?> <beans:beans xmlns="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd"> <annotation-driven /> <resources mapping="/resources/**" location="/resources/" /> <beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <beans:property name="prefix" value="/WEB-INF/views/" /> <beans:property name="suffix" value=".jsp" /> </beans:bean> <beans:bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close"> <beans:property name="driverClassName" value="com.mysql.jdbc.Driver" /> <beans:property name="url" value="jdbc:mysql://localhost:3306/CountryData" /> <beans:property name="username" value="root" /> <beans:property name="password" value="" /> </beans:bean> <!-- Hibernate 4 SessionFactory Bean definition --> <beans:bean id="hibernate4AnnotatedSessionFactory" class="org.springframework.orm.hibernate4.LocalSessionFactoryBean"> <beans:property name="dataSource" ref="dataSource" /> <beans:property name="annotatedClasses"> <beans:list> <beans:value>org.arpit.java2blog.model.Country</beans:value> </beans:list> </beans:property> <beans:property name="hibernateProperties"> <beans:props> <beans:prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect </beans:prop> <beans:prop key="hibernate.show_sql">true</beans:prop> </beans:props> </beans:property> </beans:bean> <context:component-scan base-package="org.arpit.java2blog" /> <tx:annotation-driven transaction-manager="transactionManager" /> <beans:bean id="transactionManager" class="org.springframework.orm.hibernate4.HibernateTransactionManager"> <beans:property name="sessionFactory" ref="hibernate4AnnotatedSessionFactory" /> </beans:bean> </beans:beans> |
In Spring-servlet.xml, we have done hibernate configuration.
dataSource bean is used to specify java data source. We need to provide driver, URL , Username and Password.
transactionManager bean is used to configure hibernate transaction manager. hibernate4AnnotatedSessionFactory bean is used to configure FactoryBean that creates a Hibernate SessionFactory. This is the common way to set up a shared Hibernate SessionFactory in a Spring application context, so you can use this SessionFactory to inject in Hibernate data access objects.
Create a applicationcontext.xml in WEB-INF folder, this file is used for bean configuration as we are using spring-servlet.xml for bean configuration , we will keep this file empty.
1 2 3 |
<!--?xml version="1.0" encoding="UTF-8"?--> |
Create bean class
4)Â Create a bean name “Country.java” in org.arpit.java2blog.bean.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
package org.arpit.java2blog.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; /* * This is our model class and it corresponds to Country table in database */ @Entity @Table(name="COUNTRY") public class Country{ @Id @Column(name="id") @GeneratedValue(strategy=GenerationType.IDENTITY) int id; @Column(name="countryName") String countryName; @Column(name="population") long population; public Country() { super(); } public Country(int i, String countryName,long population) { super(); this.id = i; this.countryName = countryName; this.population=population; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } public long getPopulation() { return population; } public void setPopulation(long population) { this.population = population; } } |
@Entity is used for making a persistent pojo class.For this java class,you will have corresponding table in database. @Column is used to map annotated attribute to corresponding column in table. So Create Country table in mysql database with following code:
1 2 3 4 5 6 7 8 9 |
CREATE TABLE COUNTRY ( id int PRIMARY KEY NOT NULL AUTO_INCREMENT, countryName varchar(100) NOT NULL, population int NOT NULL ) ; |
Create Controller
5) Create a controller named “CountryController.java” in package org.arpit.java2blog.controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
package org.arpit.java2blog.controller; import java.util.List; import org.arpit.java2blog.model.Country; import org.arpit.java2blog.service.CountryService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller public class CountryController { @Autowired CountryService countryService; @RequestMapping(value = "/getAllCountries", method = RequestMethod.GET, headers = "Accept=application/json") public String getCountries(Model model) { List listOfCountries = countryService.getAllCountries(); model.addAttribute("country", new Country()); model.addAttribute("listOfCountries", listOfCountries); return "countryDetails"; } @RequestMapping(value = "/getCountry/{id}", method = RequestMethod.GET, headers = "Accept=application/json") public Country getCountryById(@PathVariable int id) { return countryService.getCountry(id); } @RequestMapping(value = "/addCountry", method = RequestMethod.POST, headers = "Accept=application/json") public String addCountry(@ModelAttribute("country") Country country) { if(country.getId()==0) { countryService.addCountry(country); } else { countryService.updateCountry(country); } return "redirect:/getAllCountries"; } @RequestMapping(value = "/updateCountry/{id}", method = RequestMethod.GET, headers = "Accept=application/json") public String updateCountry(@PathVariable("id") int id,Model model) { model.addAttribute("country", this.countryService.getCountry(id)); model.addAttribute("listOfCountries", this.countryService.getAllCountries()); return "countryDetails"; } @RequestMapping(value = "/deleteCountry/{id}", method = RequestMethod.GET, headers = "Accept=application/json") public String deleteCountry(@PathVariable("id") int id) { countryService.deleteCountry(id); return "redirect:/getAllCountries"; } } |
Create DAO class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
package org.arpit.java2blog.dao; import java.util.List; import org.arpit.java2blog.model.Country; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Repository; @Repository public class CountryDAO { @Autowired private SessionFactory sessionFactory; public void setSessionFactory(SessionFactory sf) { this.sessionFactory = sf; } public List getAllCountries() { Session session = this.sessionFactory.getCurrentSession(); List countryList = session.createQuery("from Country").list(); return countryList; } public Country getCountry(int id) { Session session = this.sessionFactory.getCurrentSession(); Country country = (Country) session.load(Country.class, new Integer(id)); return country; } public Country addCountry(Country country) { Session session = this.sessionFactory.getCurrentSession(); session.persist(country); return country; } public void updateCountry(Country country) { Session session = this.sessionFactory.getCurrentSession(); session.update(country); } public void deleteCountry(int id) { Session session = this.sessionFactory.getCurrentSession(); Country p = (Country) session.load(Country.class, new Integer(id)); if (null != p) { session.delete(p); } } } |
@Repository is specialised component annotation which is used to create bean at DAO layer. We have use Autowired annotation to inject hibernate SessionFactory into CountryDAO class. We have already configured hibernate SessionFactory object in Spring-Servlet.xml file.
Create Service class
It is service level class. It will call DAO layer class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
package org.arpit.java2blog.service; import java.util.List; import org.arpit.java2blog.dao.CountryDAO; import org.arpit.java2blog.model.Country; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; @Service("countryService") public class CountryService { @Autowired CountryDAO countryDao; @Transactional public List getAllCountries() { return countryDao.getAllCountries(); } @Transactional public Country getCountry(int id) { return countryDao.getCountry(id); } @Transactional public void addCountry(Country country) { countryDao.addCountry(country); } @Transactional public void updateCountry(Country country) { countryDao.updateCountry(country); } @Transactional public void deleteCountry(int id) { countryDao.deleteCountry(id); } } |
Create view
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <%@ taglib uri="http://www.springframework.org/tags" prefix="spring" %> <%@ taglib uri="http://www.springframework.org/tags/form" prefix="form" %> <html> <head> <style> .blue-button{ background: #25A6E1; filter: progid: DXImageTransform.Microsoft.gradient( startColorstr='#25A6E1',endColorstr='#188BC0',GradientType=0); padding:3px 5px; color:#fff; font-family:'Helvetica Neue',sans-serif; font-size:12px; border-radius:2px; -moz-border-radius:2px; -webkit-border-radius:4px; border:1px solid #1A87B9 } table { font-family: "Helvetica Neue", Helvetica, sans-serif; width: 50%; } th { background: SteelBlue; color: white; } td,th{ border: 1px solid gray; width: 25%; text-align: left; padding: 5px 10px; } </style> </head> <body> <form:form method="post" modelAttribute="country" action="/SpringMVCHibernateCRUDExample/addCountry"> <table> <tr> <th colspan="2">Add Country</th> </tr> <tr> <form:hidden path="id" /> <td><form:label path="countryName">Country Name:</form:label></td> <td><form:input path="countryName" size="30" maxlength="30"></form:input></td> </tr> <tr> <td><form:label path="population">Population:</form:label></td> <td><form:input path="population" size="30" maxlength="30"></form:input></td> </tr> <tr> <td colspan="2"><input type="submit" class="blue-button" /></td> </tr> </table> </form:form> </br> <h3>Country List</h3> <c:if test="${!empty listOfCountries}"> <table class="tg"> <tr> <th width="80">Id</th> <th width="120">Country Name</th> <th width="120">Population</th> <th width="60">Edit</th> <th width="60">Delete</th> </tr> <c:forEach items="${listOfCountries}" var="country"> <tr> <td>{country.id}</td> <td>${country.countryName}</td> <td>${country.population}</td> <td><a href="<c:url value='/updateCountry/${country.id}' />" >Edit</a></td> <td><a href="<c:url value='/deleteCountry/${country.id}' />" >Delete</a></td> </tr> </c:forEach> </table> </c:if> </body> </html> |
Right click on project -> Run as -> Maven build
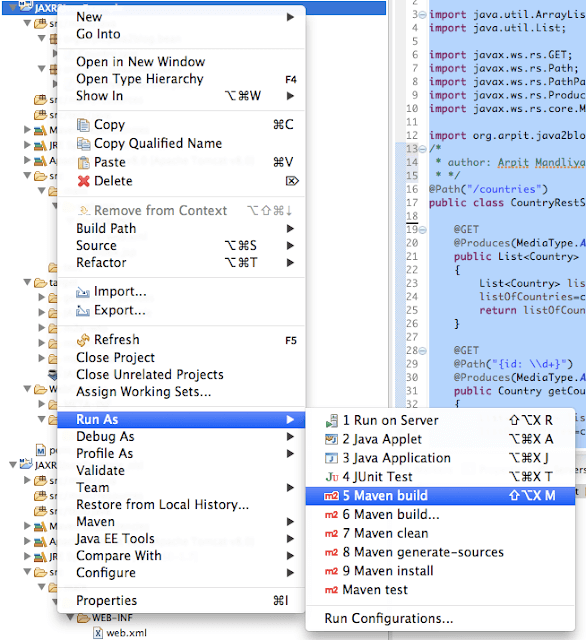
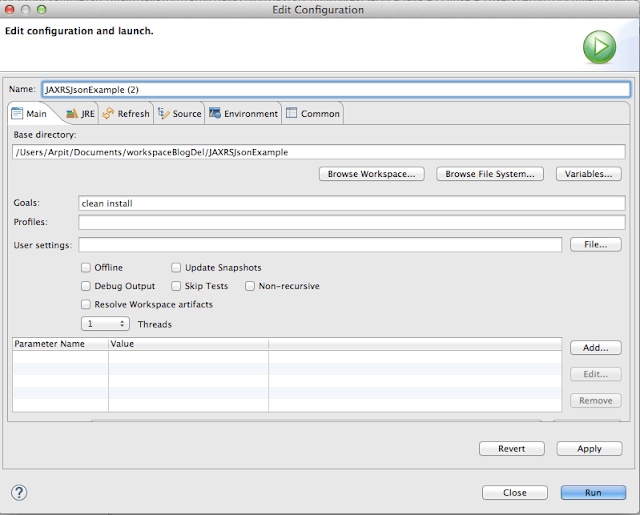
Run the application
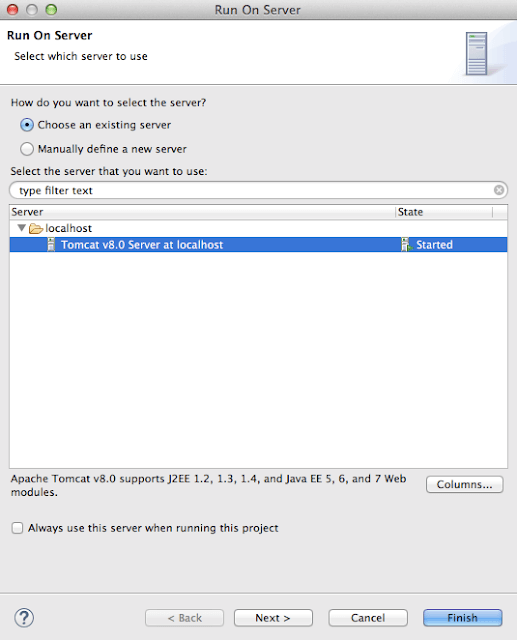
http://localhost:8080/SpringMVCHibernateCRUDExample/getAllCountries
You will get below screen:
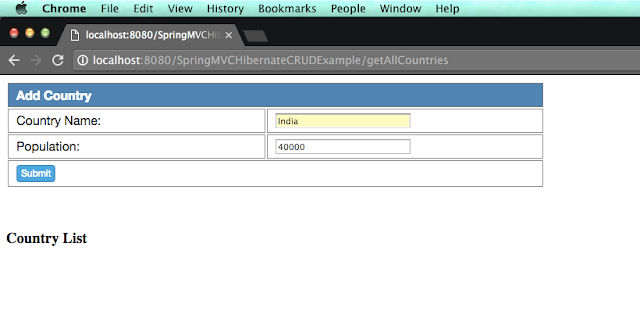
As you can see, we did not add any country to the list, so it is empty.
Lets add Country named India to country list and click submit.
Similarly we will add China , Bhutan and Nepal respectively and you will see below screen.
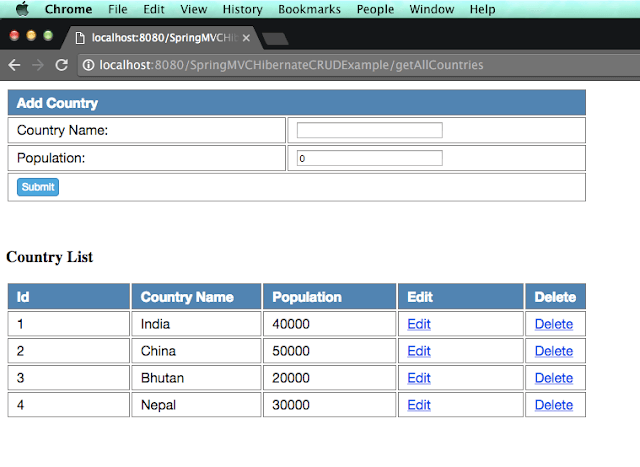
Lets edit population of Bhutan to 15000. Click on edit button corresponds to Bhutan.
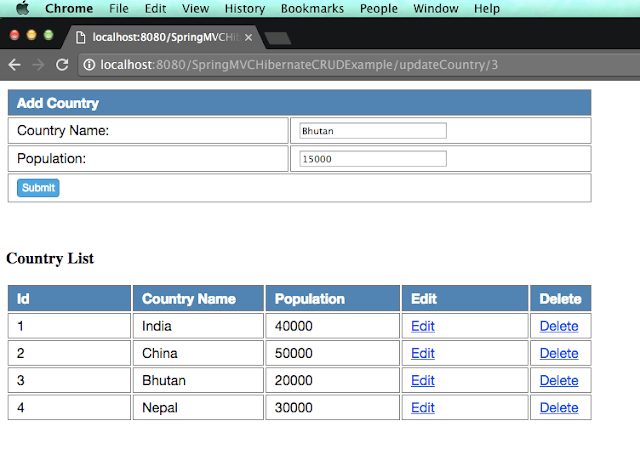
When you click on submit, you will get below screen.
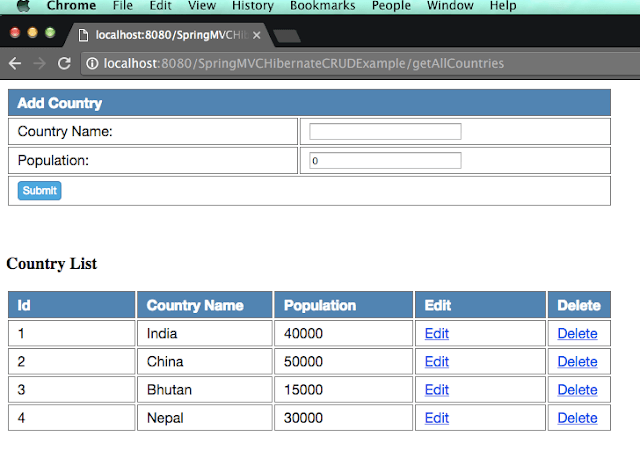
Lets delete country China from above list, click on delete button corresponds to 2nd row.
You will see below screen.
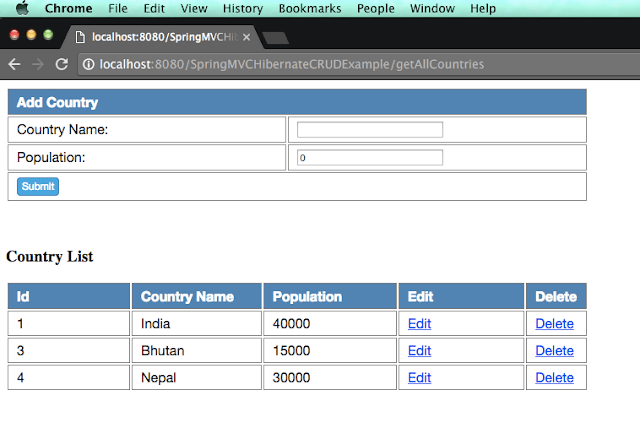
As you can see, china got deleted from above list.
Project structure:
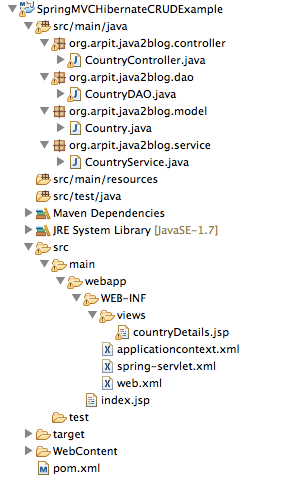
We are done with Spring MVC hibernate MySQL CRUD example. If you are still facing any issue, please comment.
EXCELLENT tutorial! This is the best yet of any I've seen. Just wanted to take a minute to say thanks for putting this together, it was extremely helpful!
Hi Friends
It is very usefull doucment please folow all the steps you get a solution
Thanks to Who has added post
hi arpit , i like u tutos. Can u explain how updateCountry method on @Controller work? when link the url for edit a country.. to get an exception.
Nice tutorial…………. working projected…..
Awesome tutorial….. I never write comments.. but this was one perfect…
Thank you so much ,awesome tutorial
The only working tutorial on the internet for hibernate
The best code I’ve seen so far.
Awesome tutorial…Only working blog, keep rocking…
Excellent way of Explaining all the tutorials.Thans
best example keep it up !!
its working properly !