Table of Contents
◈ Problem Formulation
Aim:
In this article we will discuss: TypeError: 'Module' Object Is Not Callable
in Python and the methods to overcome such errors in your program.
Example:
Consider that you have created the following module with the name Palindrome.py
:
1 2 3 4 |
def palindrome(val): return val == val[::-1] |
Now, you want to use this module in another program and check if an entered value is Palindrome or not :
1 2 3 4 5 6 7 8 9 10 11 12 |
import Palindrome text = input("Enter the value: ") ans = Palindrome(text) if ans: print("Palindrome!") else: print("Not Palindrome!") |
Let’s execute this code and check the output:
1 2 3 4 5 6 7 |
Enter the value: 21 Traceback (most recent call last): File "D:/PycharmProjects/pythonProject1/Reverse.py", line 4, in <module> ans = Palindrome(text) TypeError: 'module' object is not callable |
Wait!!! 😵 Why did we get this error? It brings us to a number of questions:
- What is a
TypeError
? - What does
TypeError: module object is not callable
mean? - Why did we encounter this error in our program?
- How to fix
TypeError: module object is not callable
?
Let us dive into each question in details and find out the correct way of calling a certain module and its attributes.
◈ What is TypeError in Python?
A TypeError
is raised when a certain operation is applied to an object of an incorrect type. For example, if you try to add a string object and an integer object using the +
operator, then you will encounter a TypeError
because the +
operation is not allowed between the two objects that are f different types.
Example:
1 2 3 |
print('Java'+2+'Blog') |
Output:
1 2 3 |
TypeError: can only concatenate str (not "int") to str |
☞ There can be numerous reasons that lead to the occurrence of TypeError
. Some of these reasons are:
- Trying to perform an unsupported operation between two types of objects.
- Trying to call a non-callable caller.
- Trying to iterate over a non-iterative identifier.
Now that we have a clear idea about TypeErrors
, let us understand what does TypeError: module object is not callable
mean?
◈ What Does TypeError: module object is not callable
Mean?
TypeError: 'module' object is not callable
is generally raised when you are trying to access a module as a function within your program. This happens when you are confused between a module name and a class name/function name within that module. For example:
1 2 3 4 5 |
import socket s = socket(socket.AF_INET, socket.SOCK_STREAM) print(s) |
Output:
1 2 3 4 5 6 |
Traceback (most recent call last): File "D:/PycharmProjects/pythonProject1/example.py", line 2, in <module> s = socket(socket.AF_INET, socket.SOCK_STREAM) TypeError: 'module' object is not callable |
In the above example, we have imported the module socket; however, within the program, we are trying to use it as a function that ultimately leads to TypeError: 'module' object is not callable
. The user got confused because the module name and the class name are the same.
◈ Therefore we now have an idea about the reason behind the occurrence of TypeError: 'module' object is not callable.
So, why did we encounter this error in our example?
The reason is already known to us from the above explanation. If you have a look at the example, you will notice that the name of the module is Palindrome
while it has a function with the name Palindrome
as well. So, that’s what created the confusion!
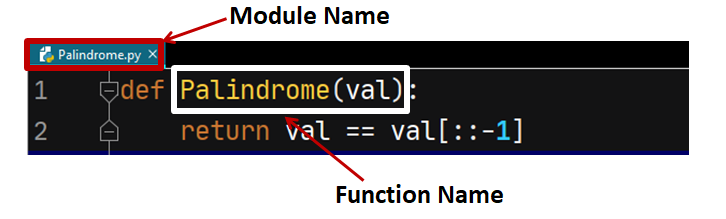
That brings us to the all important question. How do we fix the error?
◈ Fix: TypeError: ‘module’ object is not callable
There are couple of ways you can use to fix this error.
✨ Method 1: Change The “import” Statement
Instead of importing the module you can import the function or attribute within the module to avoid the TypeError
. Let’s have a look at the solution to our example scenario in the program given below.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
# import the method within the module from Palindrome import Palindrome text = input("Enter the value: ") ans = Palindrome(text) if ans: print("Palindrome!") else: print("Not Palindrome!") |
Output:
1 2 3 4 |
Enter the value: refer Palindrome! |
As Python now knows that you are referring to the Palindrome function within the Palindrome Module, hence the program runs without any error.
☞ Solution to the socket
program mentioned above:
1 2 3 4 5 6 |
from socket import * s = socket(AF_INET, SOCK_STREAM) print(s) |
Output:
1 2 3 |
<socket.socket fd=564, family=AddressFamily.AF_INET, type=SocketKind.SOCK_STREAM, proto=0> |
✨ Method 2: Use ModuleName.ClassName
Another workaround to our problem is to use ModuleName.ClassName
or ModuleName.FunctionName
instead of just using the module name which causes confusion and error.
Let’s have a look at the solution to our example scenario in the program given below.
1 2 3 4 5 6 7 8 9 10 11 |
import Palindrome text = input("Enter the value: ") ans = Palindrome.Palindrome(text) if ans: print("Palindrome!") else: print("Not Palindrome!") |
Output:
1 2 3 4 |
Enter the value: 121 Palindrome! |
Explanation: When we use Palindrome.Palindrome(text)
, it tells Python that we are trying to access the Palindrome method of the Palindrome module; therefore there’s no confusion in this case and the program runs without any error.
☞ Solution to the socket
program mentioned above:
1 2 3 4 5 6 |
import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) print(s) |
Ouput:
1 2 3 |
<socket.socket fd=204, family=AddressFamily.AF_INET, type=SocketKind.SOCK_STREAM, proto=0> |
Let’s have a look at another variation of this kind of TypeError
in Python.
Python TypeError: ‘int’ object is not callable
You will encounter TypeError: 'int' object is not callable
mostly in the following scenarios:
✨ Scenario 1: Declaring a Variable Named ‘int‘
If you declare a variable with the name int
and you also have a variable that accepts the user input with the help of the int()
method, then the Python compiler is unable to distinguish between the inbuilt int()
method and the declared variable int
in the program.
Example:
1 2 3 4 5 6 |
int = 10 a = int(input('enter a value: ')) for i in range(1, int): print(i * 5) |
Output:
1 2 3 4 5 6 7 |
enter a : 5 Traceback (most recent call last): File "D:/PycharmProjects/pythonProject1/example.py", line 2, in a=int(input('enter a : ')) TypeError: 'int' object is not callable |
Solution: Use a different name for the variable
1 2 3 4 5 6 7 |
k = 10 a = int(input('enter a value: ')) for i in range(1, k): print(i * 5) |
Output:
1 2 3 4 5 6 7 8 9 10 11 12 |
enter a value: 5 5 10 15 20 25 30 35 40 45 |
✨ Scenario 2: Declaring a Variable With a Name Of Function That Computes Integer Values
Suppose you have a variable by the name sum
in your program and you are also using the built-in function sum()
within your program then you will come across the TypeError: 'int' object is not callable
since Python won’t be able to distinguish between the two sum
variable and the sum() method.
1 2 3 4 5 6 7 8 9 10 11 |
Amount = [500, 600, 700] Discount = [100, 200, 300] sum = 0 if sum(Amount) < 5000: print(sum(Amount) - 1000) else: sum = sum(Amount) - sum(Discount) print(sum) |
Output:
1 2 3 4 5 6 |
Traceback (most recent call last): File "D:/PycharmProjects/pythonProject1/example.py", line 4, in <module> if sum(Amount) < 5000: TypeError: 'int' object is not callable |
Solution: Use different variable instead of sum
1 2 3 4 5 6 7 8 9 10 11 |
Amount = [500, 600, 700] Discount = [100, 200, 300] value = 0 if sum(Amount) < 5000: print(sum(Amount) - 1000) else: value = sum(Amount) - sum(Discount) print(value) |
Output:
1 2 3 |
800 |
Conclusion
Key take-aways from this article:
- What is TypeError in Python?
- What Does TypeError: module object is not callable Mean?
- Fixing the – TypeError: ‘module’ object is not callable
- Python TypeError: ‘int’ object is not callable