In this post, we will see how to save DataFrame to a CSV file in Python pandas.
DataFrame.to_csv()
to save DataFrame to CSV file. We will see various options to write DataFrame to csv file.
Syntax of DataFrame.to_csv()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
def to_csv( self, path_or_buf=None, sep=",", na_rep="", float_format=None, columns=None, header=True, index=True, index_label=None, mode="w", encoding=None, compression="infer", quoting=None, quotechar='"', line_terminator=None, chunksize=None, date_format=None, doublequote=True, escapechar=None, decimal=".", ) |
Here,
path_or_buf:
Path where you want to write CSV file including file name. If you do not pass this parameter, then it will return String.
sep:
Field delimter from output file. Default value is ,
na_rep:
Missing data representation.
float_format:
To format float point numbers, you can use this parameter.
columns:
Columns to write to CSV file.
header:
Write out column names.
index:
To avoid index(row no) column, you can passindex=False
index_label
Column label for index column.
We will see meaning of few important attributes with the help of example.
DataFrame sample data
Here is sample data which are going to write to CSV file.
Name | Age | Gender |
---|---|---|
Mary | 36 | Female |
John | 29 | Male |
Martin | 31 | Male |
Convert pandas DataFrame to csv
Here are steps to write DataFrame to CSV file.
- Import pandas module using import statement
123import pandas as pd - Create a DataFrame as per above sample data.
12345678emp_df = pd.DataFrame({'Name': ['Mary','John','Martin'],'Age': [36,29,31],'Gender':['Female','Male','Male']})print(emp_df) -
Decide the path where you want to save file and pass it to to_csv function.
You can specify either a relative path or an absolute path.
Relative path:
123emp_df.to_csv('Employees.csv')
123emp_df.to_csv('/users/apple/Employees.csv')
Here is the complete python program to save CSV file.
1 2 3 4 5 6 7 8 9 10 11 12 |
import pandas as pd emp_df = pd.DataFrame({ 'Name': ['Mary','John','Martin'], 'Age': [36,29,31], 'Gender':['Female','Male','Male'] }) print(emp_df) emp_df.to_csv('/users/apple/Employees.csv') |
When you run the above program, Employees.csv
will be created a specified path.
Here is data for the csv file.
Save CSV file without index column
If you don’t want to have index column, you can simply pass index=False
to to_csv()
function as below:
1 2 3 |
emp_df.to_csv('Employees.csv',index=False) |
When you run the program again, you will get below output:
Save CSV file with Custom columns header
You can change columns header by passing header
list to to_csv()
as below:
1 2 3 |
emp_df.to_csv('Employees.csv',index=False,header = ['Emp_Name','Emp_Age','Emp_Gender']) |
When you run the program again, you will get below output:
In preceding output, you can see that columns header is changed as per the header
list.
Save CSV file with selected columns
To write selected columns to csv, you can pass columns
list to function to_csv()
as below:
1 2 3 |
emp_df.to_csv('Employees.csv', index=False, columns = ['Name','Age']) |
When you run the program again, you will get below output:
In preceding output, you can see that Name
and Age
columns were saved to CSV file.
Save CSV file with custom delimiter
You can also pass custom delimiter with sep
attribute as argument.
Let’s say you want to use ‘|’ as a separator, you can do as below:
1 2 3 |
emp_df.to_csv('Employees.csv', index=False, columns = ['Name','Age']) |
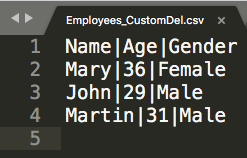
Save CSV file without header
You can also pass header=False
to save CSV file without header.
Let’s say you want to use ‘|’ as a separator, you can do as below:
1 2 3 |
emp_df.to_csv('Employees.csv', index=False, header=False) |
Output:
In preceding output, you can see that CSV file does not contain header.
Append data to CSV file
You can also append data to CSV file using mode='a'
and pass header=False
, otherwise header will be repeated in append mode.
Let’s see with the help of example:
1 2 3 4 5 6 7 8 9 |
emp_app_df = pd.DataFrame({ 'Name': ['Amar'], 'Age': [22], 'Gender':['Male'] }) print(emp_app_df) emp_df.to_csv('Employees.csv', index=False, mode='a',header=False) |
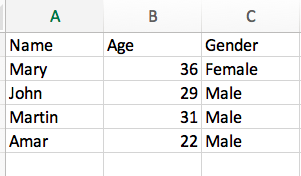
In preceding output, you can see that new row is appended to csv file.
Save CSV file with index_label
If you want to assign name to index column, you can pass index_label
to to_csv()
as below:
1 2 3 |
emp_df.to_csv('Employees.csv',index_label='Serial No.') |
Output:
In preceding output, you can see that index column has heading named Serial no
.
That’s all about Pandas DataFrame to CSV.