Table of Contents
If you want to practice data structure and algorithm programs, you can go through 100+ java coding interview questions.
In this post, we will see how to find lowest common ancestor(LCA) of two nodes in binary tree. Lets understand with example.
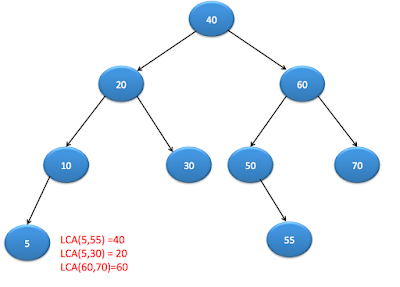
As you can see here, LCA is nothing but lowest common parent of two nodes.
Recursive Algorithm (For nodes A and B):
- If node is null, return it
- If we find A or B, return it.
- Traverse left subtree and right subtree
- Â If we get both left and right for any node as not null, it will be lowest common ancestor of two given nodes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
public static TreeNode lowestCommonAncestor(TreeNode root, TreeNode a, TreeNode b) { if(root == null) return null; if(root.data == a.data || root.data == b.data ) return root; TreeNode left=lowestCommonAncestor(root.left,a,b); TreeNode right=lowestCommonAncestor(root.right,a,b); // If we get left and right not null , it is lca for a and b if(left!=null && right!=null) return root; if(left== null) return right; else return left; } |
Complete java program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
package org.arpit.java2blog.binarytree; public class BinaryTreeLCA { public static class TreeNode { int data; TreeNode left; TreeNode right; TreeNode(int data) { this.data=data; } } public static TreeNode lowestCommonAncestor(TreeNode root, TreeNode a, TreeNode b) { if(root == null) return null; if(root.data == a.data || root.data == b.data ) return root; TreeNode left=lowestCommonAncestor(root.left,a,b); TreeNode right=lowestCommonAncestor(root.right,a,b); // If we get left and right not null , it is lca for a and b if(left!=null && right!=null) return root; if(left== null) return right; else return left; } public static void main(String[] args) { // Creating a binary tree TreeNode rootNode=createBinaryTree(); System.out.println("Lowest common ancestor for node 5 and 30:"); TreeNode node5=new TreeNode(5); TreeNode node30=new TreeNode(30); System.out.println(lowestCommonAncestor(rootNode,node5,node30).data); } public static TreeNode createBinaryTree() { TreeNode rootNode =new TreeNode(40); TreeNode node20=new TreeNode(20); TreeNode node10=new TreeNode(10); TreeNode node30=new TreeNode(30); TreeNode node60=new TreeNode(60); TreeNode node50=new TreeNode(50); TreeNode node70=new TreeNode(70); TreeNode node5=new TreeNode(5); TreeNode node45=new TreeNode(45); TreeNode node55=new TreeNode(55); rootNode.left=node20; rootNode.right=node60; node20.left=node10; node20.right=node30; node60.left=node50; node60.right=node70; node10.left=node5; node50.right=node55; return rootNode; } } |
1 2 3 4 |
Lowest common ancestor for node 5 and 30: 20 |
Java Binary tree tutorial:
- Binary tree in java
- Binary tree preorder traversal
- Binary tree postorder traversal
- Binary tree inorder traversal
- Binary tree level order traversal
- Binary tree spiral order traversal
- Binary tree reverse level order traversal
- Binary tree boundary traversal
- Print leaf nodes of binary tree
- Count leaf nodes in binary tree
- get maximum element in binary tree
- Print all paths from root to leaf in binary tree
- Print vertical sum of binary tree in java
- Get level of node in binary tree in java
- Lowest common ancestor(LCA) in binary tree in java
Please go through java interview programs for more such programs.
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.