Table of Contents
@ModelAttribute is one of most important Spring MVC annotation.
Spring MVC tutorial:
- Spring MVC hello world example
- Spring MVC Hibernate MySQL example
- Spring MVC interceptor example
- Spring MVC angularjs example
- Spring MVC @RequestMapping example
- Spring Component,Service, Repository and Controller example
- Spring MVC @ModelAttribute annotation example
- Spring MVC @RestController annotation example
- Spring MultiActionController Example
- Spring MVC ModelMapSpring MVC file upload example
- Spring restful web service example
- Spring restful web service json example
- Spring Restful web services CRUD example
- Spring security hello world example
- Spring security custom login form example
@ModelAttribute is used to bind request parameters with model object. Â Lets say you have 10 request parameters , you can wrap them to model object and pass it in the to handler method.
- Object is already present in session or ModelMap object.
- Annotate another method with @ModelAttribute annotation and create the model object. This method will get called before actual handler call.
Example:
Source code:
Here are steps to create a Spring MVC ModelAttribute example.
Maven dependencies
2)Â We need to add Jackson json utility in the classpath.
1 2 3 4 5 6 7 |
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> |
Now change pom.xml as follows:
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringRestfulWebServicesWithJSONExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringRestfulWebServicesWithJSONExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> </dependencies> <build> <finalName>SpringRestfulWebServicesWithJSONExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <jdk.version>1.7</jdk.version> </properties> </project> |
Spring application configuration:
3)Â Change web.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" version="3.0"> <display-name>Archetype Created Web Application</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>springrest</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springrest</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> |
4)Â create a xml file named springrest-servlet.xml in /WEB-INF/ folder.
Please change context:component-scan if you want to use different package for spring to search for controller.Please refer to spring mvc hello world example for more understanding.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> <mvc:annotation-driven/> <context:component-scan base-package="org.arpit.java2blog.controller" /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix"> <value>/WEB-INF/</value> </property> <property name="suffix"> <value>.jsp</value> </property> </bean> <mvc:default-servlet-handler/> </beans> |
Create bean class
5)Â Create a bean name “Country.java” in org.arpit.java2blog.bean.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
package org.arpit.java2blog.bean; public class Country{ String countryName; long population; public Country() { super(); } public Country(String countryName,long population) { super(); this.countryName = countryName; this.population=population; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } public long getPopulation() { return population; } public void setPopulation(long population) { this.population = population; } } |
Create Controller
6) Create a controller named “CountryController.java” in package org.arpit.java2blog.controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
package org.arpit.java2blog.controller; import org.arpit.java2blog.bean.Country; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; @Controller public class CountryController { @ModelAttribute public Country getCountry(@RequestParam String countryName, @RequestParam long population) { Country country=new Country(); country.setCountryName(countryName); country.setPopulation(population); return country; } @RequestMapping(value = "/addCountry", method = RequestMethod.POST) public String addCountry(@ModelAttribute Country country,ModelMap model) { model.addAttribute("countryName", country.getCountryName()); model.addAttribute("population", country.getPopulation()); return "countryDetails"; } } |
1 2 3 |
Create view |
Modify index.jsp as below
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
<head> <style> .blue-button{ background: #25A6E1; filter: progid: DXImageTransform.Microsoft.gradient( startColorstr='#25A6E1',endColorstr='#188BC0',GradientType=0); padding:3px 5px; color:#fff; font-family:'Helvetica Neue',sans-serif; font-size:12px; border-radius:2px; -moz-border-radius:2px; -webkit-border-radius:4px; border:1px solid #1A87B9 } table { font-family: "Helvetica Neue", Helvetica, sans-serif; width: 50%; } th { background: SteelBlue; color: white; } td,th{ border: 1px solid gray; width: 25%; text-align: left; padding: 5px 10px; } </style> </head> <body> <form action="addCountry" method="post"> <table> <tr> <th colspan="2">Add Country</th> </tr> <tr> <td>Country</td> <td><input type="text" name=countryName></td> </tr> <tr> <td>Population</td> <td><input type="text" name=population> </td></tr> <tr> <td colspan="2"><input type="submit" class="blue-button" /></td> </tr> </table> </form> </body> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Country Details</title> </head> <body> Added country : <h3>Country Name: {countryName}</h3> <h3>Population : ${population}</h3> </body> </html> |
7)Â It ‘s time to do maven build.
Right click on project -> Run as -> Maven build
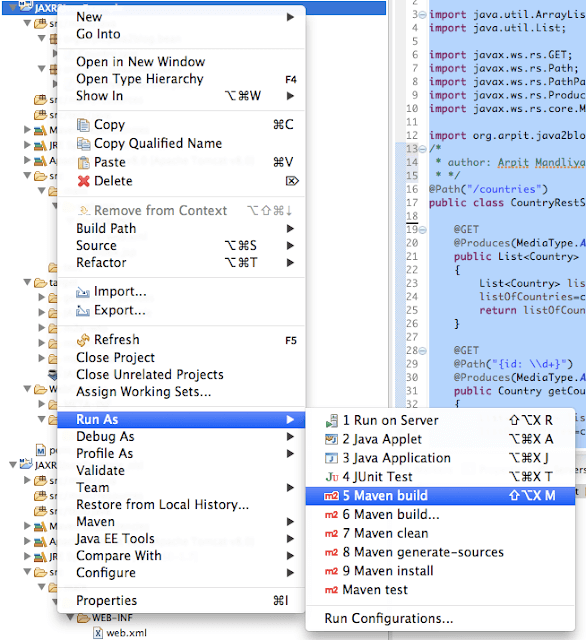
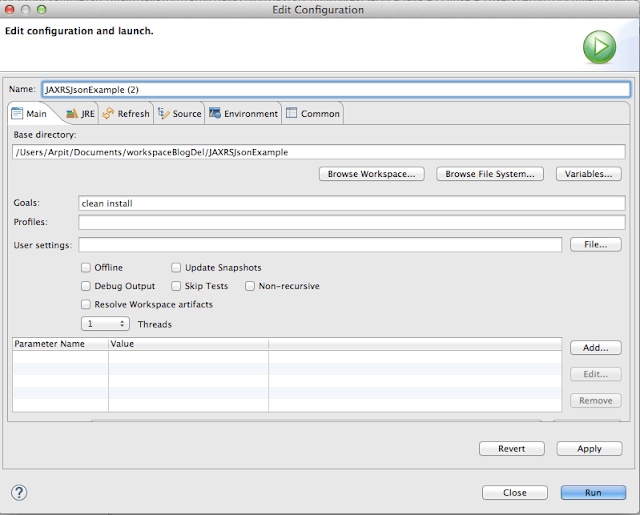
Run the application
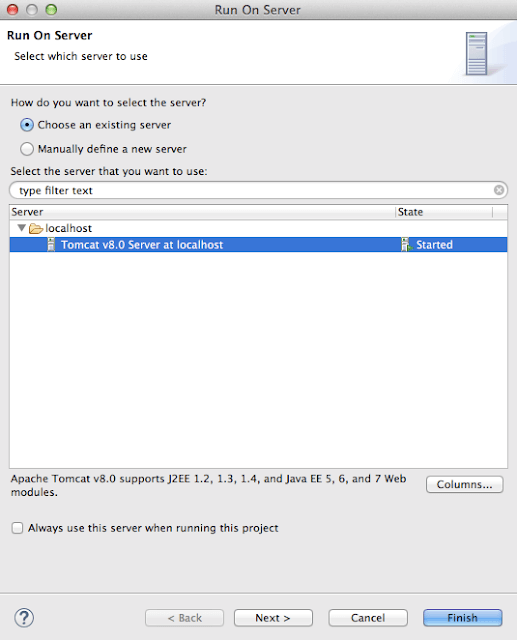
10) You will see screen as below:
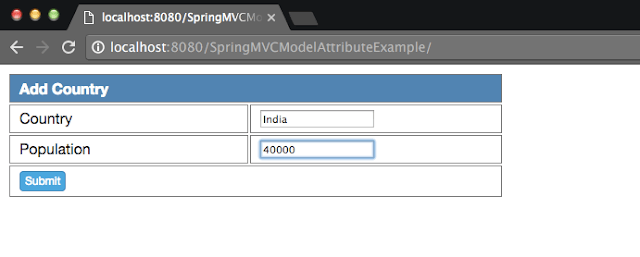
11) When you click on submit button , you will see below screen.
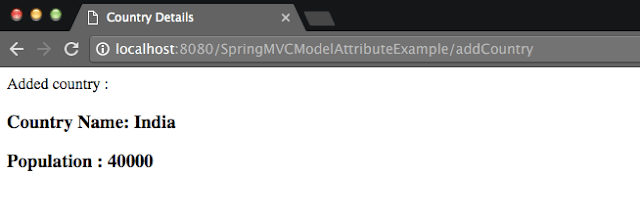