Hibernate is most popular Object relational Mapping (ORM) framework and if you have used hibernate and has good experience on it, then you may face good hibernate interview questions. I am sharing some important hibernate interview questions here.
Table of Contents
- 1. What is ORM?
- 2. What is Hibernate?
- 3. What are advantages of Hibernate?
- 4. Explain architecture of Hibernate?
- 5. What are some core interfaces of hibernate?
- 6. Explain brief about Session interface used in hibernate?
- 7. Are session objects thread safe?
- 8. Explain brief about SessionFactory object used in hibernate?
- 9. What is Configuration class in hibernate?
- 10. Explain brief about Criteria API in Hibernate?
- 11. Explain brief about Query interface in Hibernate?
- 12. What are differences between openSession and getCurrentSession in hibernate?
- 13. What are differences between get and load methods in hibernate?
- 14. Can you declare Entity class as final in hibernate?
- 15. Differentiate between first level cache and second level cache?
- 16. What are states of object in hibernate?
- 17. Does entity class in hibernate require no arg constructor?
- 18. What is HQL?
- 19. What are differences between save and saveOrUpdate method of session object?
- 20. What are two types of Collections in hibernate?
- 21. What are differences between sorted and ordered collection in hibernate?
- 22. What is lazy loading in hibernate?
- 23. What is LazyInitializationException in Hibernate? Why do you get it?
- 24. What are different collection types available in hibernate?
- 25. How can you log sql queries executed by Hibernate?
- 26. Can you execute native sql in hibernate?
- 27. What are inheritance mapping strategies are available in Hibernate?
- 28. How to make an class immutable in Hibernate?
- 29. What is autamatic dirty checking in Hibernate?
- 30. What do you understand by Hibernate tuning?
- 31. What types of joins can you use in Hibernate?
- 32. What is dialect in Hibernate?
- 33. Can you share some of the databases supported by Hibernate?
- 34. What is Named queries in Hibernate?
- 35. What is Query cache in Hibernate?
- 36. What are benefits of Criteria API in Hibernate?
- 37. What is cascading in Hiberante and can you list types of cascading?
- 38. How to integrate log4j with Hibernate?
- 39. What is hibernate configuration file?
- 40. Can you list down important annotations used for Hibernate mapping?
- 41. What are the design patterns used in Hibernate?
- 42. Given a Customer class, you need to persist customer data in the database with customer ID as primary key. Please list down the changes you need to make?
1. What is ORM?
ORM
stands for Object Relational mapping. It is programming paradigm which is used to persist java objects  to database tables.
2. What is Hibernate?
Hibernate
is pure ORM tool which is used to save old java objects to database tables. The main goal of hibernate to avoid old JDBC code
and focus more on business logic. You need to write less code with it.
3. What are advantages of Hibernate?
Advantages of Hibernate are:
- Lazy Loading
- Caching
- You do not need to maintain JDBC code , Hibernate takes care of it.
- You need to write less code
- It provides high level object oriented API
4. Explain architecture of Hibernate?
Following is detail architecture of hibernate with core classes.
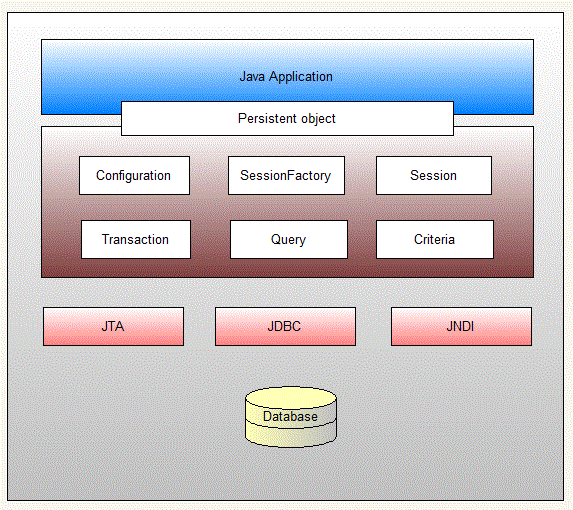
Hibernate is like a bridge between java code and relation database and provide object oriented API to deal with JDBC tasks.
5. What are some core interfaces of hibernate?
- Session
- SessionFactory
- Configuration
- Transaction
- Query and Criteria interface.
6. Explain brief about Session interface used in hibernate?
Session
interface is primarily used by hibernate application. Session is light weight,short lived objects which are inexpensive to create and destroy. It allows you to create query objects to retrieve persistent objects.It wraps JDBC connection Factory for Transaction.It holds a mandatory (first-level) cache of persistent objects, used when navigating the object graph or looking up objects by identifier .
7. Are session objects thread safe?
Session
objects are not thread safe and need to be used in single thread. Every thread should have their own session object and close it once it is done with the work.
8. Explain brief about SessionFactory object used in hibernate?
SessionFactory is heavy weight object and it should be created one per database. SessionFactory object is shared by multiple sessions.
9. What is Configuration class in hibernate?
Configuration class is used to load required hibernate configuration. It is used to bootstrap hibernate and it is used to locate to hibernate mapping file.
10. Explain brief about Criteria API in Hibernate?
Criteria API is a API for retrieving entities by composing Criterion objects also referred as Criterion query.
Criteria API is elegant way for building dynamic queries on the persistence database.
Let’s understand with the help of example.
You have Employee class with two attributes i.e. name and age.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 |
package org.arpit.java2blog; /* * This is our model class and it corresponds to Employee table in database */ @Entity @Table(name="EMPLOYEE") public class Employee { @Id @Column(name="id") @GeneratedValue(strategy=GenerationType.IDENTITY) int id; @Column(name="name") String name; @Column(name="age") int age; public Employee(String name) { this.name = name; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } |
You want to get list of employees whose name start with A and age is greater than 30.
You can write query as below.
1 2 3 4 5 6 |
Criteria criteria = session.createCriteria(Employee.class); criteria.add(Restrictions.like("name","A%"); criteria.add(Restrictions.gt("age",30); List<Employee> employeeList = criteria.list(); |
11. Explain brief about Query interface in Hibernate?
Query interface is object oriented representation of Hibernate Query. You can get query object bu calling Session.createQuery()
method.
Here is simple example to execute Native query using Query APIs.
1 2 3 4 5 6 7 8 9 10 |
SQLQuery query = session.createSQLQuery("select name, age from Employee"); List<Object[]> rows = query.list(); for(Object[] row : rows){ Employee e = new Employee(); e.setName(row[0].toString()); e.setAge(Integer.parseInt(row[1].toString())); System.out.println(e); } |
12. What are differences between openSession and getCurrentSession in hibernate?
Parameter
|
openSession
|
getCurrentSession
|
---|---|---|
Session object
|
It always create new Session object
|
It creates a new Session if not exists , else use same session which is in current hibernate context
|
Flush and close
|
You need to explicitly flush and close session objects
|
You do not need to flush and close session objects, it will be automatically taken care by Hibernate internally
|
Performance
|
In single threaded environment , It is slower than getCurrentSession
|
In single threaded environment , It is faster than getOpenSession
|
Configuration
|
You do not need to configure any property to call this method |
You need to configure additional property “hibernate.current_session_context_class” to call getCurrentSession method, otherwise it will throw exceptions.
|
You can also refer difference between opensession and getCurrentSession in hibernate.
13. What are differences between get and load methods in hibernate?
This is one of the most asked hibernate interview questions.
Parameter
|
get
|
load
|
---|---|---|
Database retrieval
|
It always hits the database
|
It does not hit database
|
If null
|
If it does not get the object with id, it returns null
|
If it does get the object with id, it throws ObjectNotFoundException
|
Proxy
|
It returns real object
|
It returns proxy object
|
Use
|
If you are not sure if object with id exists or not, you can use get |
If you are sure about existence of object, you can use load
|
14. Can you declare Entity class as final in hibernate?
Yes, you can declare entity class as final but it is not considered as a good practice because hibernate uses proxy pattern for lazy initialisation, If you declare it as final then hibernate won’t be able to create sub class and won’t be able to use proxy pattern, so it will limit performance and improvement options.
15. Differentiate between first level cache and second level cache?
This is one of most important hibernate interview questions.
Parameter
|
First level Cache
|
Second level Cache
|
---|---|---|
Association
|
It is associated at Session level. |
It is associated at SessionFactory level and is generally exists one per application.
|
Default
|
It is enabled by default |
It is not enabled by default, you need to enable it explicitly
|
16. What are states of object in hibernate?
17. Does entity class in hibernate require no arg constructor?
Yes, Entity class in hibernate requires no arg constructor because Hibernate use reflection to create instance of entity class and it mandates no arg constructor in Entity class.
18. What is HQL?
HQL stands for Hibernate query language. It is very simple, efficient and object oriented query languages which simplifies complex SQL queries. Instead of table, you use object to write queries.
19. What are differences between save and saveOrUpdate method of session object?
save():
 stores object in database. It generates identifier for tQhe object and returns it. If object already exists in database, it will throw an error.saveOrUpdate():
SaveOrUpdate method save the object if identifies does not exist. If it exists , it calls update method.20. What are two types of Collections in hibernate?
- Sorted Collection
- Ordered Collection
21. What are differences between sorted and ordered collection in hibernate?
Parameter
|
Sorted Collection
|
Ordered Collection
|
---|---|---|
Sorting
|
Sorted collection uses java’s sorting API to sort the collection. |
Ordered Collections uses order by clause while retrieval of objects
|
Default
|
It is enabled by default |
It is not enabled by default, you need to enable it explicitly
|
22. What is lazy loading in hibernate?
23. What is LazyInitializationException in Hibernate? Why do you get it?
24. What are different collection types available in hibernate?
There are 5 collection types available in Hibernate for one to many relationship mappings.
- Bag
- Set
- List
- Map
- Array
25. How can you log sql queries executed by Hibernate?
You can set hibernate.show_sql
to true
for logging sql queries in Hibernate configuration file.
1 2 3 |
<property name="hibernate.show_sql">true</property> |
26. Can you execute native sql in hibernate?
Yes, you can use execute native sql with the help of SQLQuery
object in Hibernate.
Here is the example to fetch list of employees from database and create corrresponding Employee objects.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Crate session object SessionFactory sf = HibernateUtil.getSessionFactory(); Session session = sf.getCurrentSession(); // Get list of employees with help of SQLQuery. Transaction tx = session.beginTransaction(); SQLQuery sqlQuery = session.createSQLQuery("select id, name, age from Employee"); List<Object[]> rows = sqlQuery.list(); for(Object[] row : rows){ Employee employee = new Employee(); employee.setId(Long.parseLong(row[0].toString())); employee.setName(row[1].toString()); employee.setAge(Integer.parseInt(row[2].toString())); System.out.println(employee); } |
27. What are inheritance mapping strategies are available in Hibernate?
There are three strategies supported by Hibernate. You can use xml files or JPA annotation to implement them.
- [Table per Hierarchy](https://java2blog.com/hibernate-inheritancetable-per-class/ “Table per Hierarchy”)
- [Table per concrete class](https://java2blog.com/hibernate-inheritancetable-per-concrete/ “Table per concrete class”)
- [Table per subclass](https://java2blog.com/hibernate-inheritancetable-per-subclass/ “Table per subclass”)
28. How to make an class immutable in Hibernate?
You can mark class as mutable=false
and the class will become immutable.
29. What is autamatic dirty checking in Hibernate?
if object is modified in the transaction, then its state will be updated automatically when you committ the transaction.
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
SessionFactory factory = cfg.buildSessionFactory(); Session session = factory.openSession(); Transaction tx=session.beginTransaction(); Employee e1 = (Employee) session.get(Employee.class, Integer.valueOf(10001)); e1.setAge(32); tx.commit(); session.close(); |
Here, we are updating age of employe after getting employee instance and this is updated automatically when we have committed the transaction.
30. What do you understand by Hibernate tuning?
Hibernate tuning is process of optimizing performance of Hibernate application.
Some of the performance tuning strategies are:
- Data Caching
- Session management
- SQL optimization
31. What types of joins can you use in Hibernate?
There are multiple ways to use join in Hibernate.
- Using relationships such as one-to-one, one-to-many or many-to-many
- Using joins in native SQL query
- Using joins in HQL
32. What is dialect in Hibernate?
Dialect specifies type of database used in Hibernate, so that Hibernate can generate type of SQL statements.
For example:
Dialect for mysql database: org.hibernate.dialect.MySQL5Dialect
Dialect for sqlserver database: org.hibernate.dialect.SQLServer2005Dialect
Here are some of the databases supported by Hibernate:
- MySQL
- SQLServer
- PostgreSQL
- FrontBaase
- Oracle
- Sybase SQL Server
34. What is Named queries in Hibernate?
Named queries helps you to group HQL/SQL statements at single location. You can refer it by name in code when you want to use them. It helps you to avoid code mess that can happend because of scattered queriest through the project.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
package org.arpit.java2blog; @NamedQueries( { @NamedQuery( name = "getEmployeeByName", query = "from Employee e where e.name = :name" ) } ) /* * This is our model class and it corresponds to Employee table in database */ @Entity @Table(name="EMPLOYEE") public class Employee { @Id @Column(name="id") @GeneratedValue(strategy=GenerationType.IDENTITY) int id; @Column(name="name") String name; @Column(name="age") int age; public Employee(String name) { this.name = name; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } |
You can execute the query in main class as below:
1 2 3 4 5 6 7 |
TypedQuery query = session.getNamedQuery("getEmployeeByName"); query.setParameter("name","John"); List<Employee> employees=query.getResultList(); System.out.println(employees); |
35. What is Query cache in Hibernate?
If you have queries that run over and over again,with same parameters, then query caching can you performance improvements in the application.
Benifit from caching query results is very limited and dependent on the usage of the application. This is reason Hibernate disables query level cache by default.
To enable, you need to do following:
- Set
hibernate.cache.use_query_cache
in hibernate config file.
123<property name="hibernate.cache.use_query_cache">true</property> - You need to enable query cache for specific queries. Here is an example:
12345678List<Employees> employee = session.createQuery("from Employee e where e.name = :name").setEntity("employee", employee).setMaxResults(15).setCacheable(true).setCacheRegion("employeeReg").list();
36. What are benefits of Criteria API in Hibernate?
Criteria API can be used to fetch entity from database using object oriented apporach.
Here are the advantages of criteria APIs.
- Criteria API is cleanm nice and Object oriented API.
- Criteria API provides Projection that can be used for aggregate functions like sum(), min() etc.
- You can write more flexible and dynamic queries as compared to HQL.
- It has addOrder() method which can be used for ordering the results.
37. What is cascading in Hiberante and can you list types of cascading?
Most of the time, if Entity relationships depend on existence of other entity.
For example:
In case of Employee-Address relationship, if Employee is removed from database, then Address does not make sense of its own. So when you remove Employee from database, then its associated Address should also be removed.
You can use Cascading to achieve this. When you perform an action on entity, same action can be performed on associated entity.
Here are the Cascading types supported by Hibernate:
Cascade Operation
|
Description
|
---|---|
ALL
|
All the operations will be applied to parent entity’s associated entity. All operation includes DETACH, MERGE, PERSIST, REFRESH, REMOVE etc. |
DETACH
|
If parent entitiy is detached from context, then the associated entity will also be detached. |
MERGE
|
If parent entitiy is merged into the context, then the associated entity will also be merged. |
PERSIST
|
If parent entitiy is persisted into the context, then the associated entity will also be persisted. |
REFRESH
|
If parent entitiy is refreshed in the curent persistence context, then the associated entity will also be persisted. |
REMOVE
|
If parent entitiy is removed from the curent persistence context, then the associated entity will also be removed. |
38. How to integrate log4j with Hibernate?
For log4j configuration, you can follow following steps:
- Add log4j dependencies for maven project. If it is not maven project, then add required log4j jars to classpath.
- Create [log4j.xml](https://java2blog.com/log4j-xml-configuration-example/ “log4j.xml”) or
log4j.properties
and put in the classpath. - Use
DOMConfigurator
orPropertyConfigurator
to configure log4j in static block for standalone application. If you have web application, then you can useServletContextListener
to configure it.
39. What is hibernate configuration file?
Hibernate configuration file contains database configurations such as database url, username, password and dialect etc and is used to initialize SessionFactory
. It also contains mapping files and entity class details.
40. Can you list down important annotations used for Hibernate mapping?
Here are some important annotations that can be used for Hibernate mapping.
@Entity
: It is used to define class as entity bean.
@Table
: It is used to define table name in database corrresponding to Entity.
@Id
: It is used to define primary key in the entity bean.
@Column
: It is used to define column properties in database corrresponding to entity bean property.
@OneToOne
, @ManyToOne
, @ManyToMany
: These annotations are used to define relationships between various entities.
@JoinColumn
: It is used to specify a mapped column for joining an entity association.
Here is an example:
We are using two entities Country and Capital with one to one relationship.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 |
package org.arpit.java2blog; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.OneToOne; import javax.persistence.Table; @Entity @Table(name="COUNTRY") public class Country { @Id @Column(name="Country_Name") String countryName ; @OneToOne @JoinColumn(name="Capital_Name") Capital capital; @Column(name="Country_Population") long countryPopulation; public Country() { } public Country(String countryName, long countryPopulation) { this.countryName = countryName; this.countryPopulation = countryPopulation; } public long getCountryPopulation() { return countryPopulation; } public void setCountryPopulation(long countryPopulation) { this.countryPopulation = countryPopulation; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } public Capital getCapital() { return capital; } public void setCapital(Capital capital) { this.capital = capital; } } |
Capital.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
package org.arpit.javapostsforlearning;import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name="CAPITAL") public class Capital { @Id @Column(name="Capital_Name") String capitalName; @Column(name="Capital_Population") long capitalPopulation; public Capital() { } public Capital(String capitalName, long capitalPopulation) { super(); this.capitalName = capitalName; this.capitalPopulation = capitalPopulation; } public String getCapitalName() { return capitalName; } public void setCapitalName(String capitalName) { this.capitalName = capitalName; } public long getCapitalPopulation() { return capitalPopulation; } public void setCapitalPopulation(long capitalPopulation) { this.capitalPopulation = capitalPopulation; } } |
41. What are the design patterns used in Hibernate?
Here are some of the design patterns used in Hibernate:
- Proxy pattern for lazy loading
- Factory pattern in
SessionFactory
- Query object for
Criteria
API - Data Mapper: A pattern in which layer of matters that flows data between object and databases while keeping them independent of each other
Domain Model pattern
: An object model of the domain that incorporates both data and behavior.
42. Given a Customer class, you need to persist customer data in the database with customer ID as primary key. Please list down the changes you need to make?
Here is definition of table CUSTOMER.
1 2 3 4 5 6 7 8 |
CREATE TABLE CUSTOMER ( id int(11) NOT NULL AUTO_INCREMENT, Customer_Name varchar(255) DEFAULT NULL, email varchar(255) DEFAULT NULL, PRIMARY KEY (id) ) |
Here is the definition of Customer class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
package org.arpit.java2blog.model; /* * This is our model class and it corresponds to Customer table in database */ = public class Customer{ int id; String customerName; String email; public Customer() { super(); } public Customer(String customerName,String email) { super(); this.customerName=customerName; this.email=email; } public String getCustomerName() { return customerName; } public void setCustomerName(String customerName) { this.customerName = customerName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public int getId() { return id; } public void setId(int id) { this.id = id; } } |
Here are the changes that needs to be done:
- Annotate the Customer class with
@Entity
to declare it as Hibernate entity - Use
@Table
annotation to map class to table name in databse - Use
@id
and@GeneratedValue
annotation to make id as primary key - Use
@Column
to map attribute to corresponding database column
Here is the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
package org.arpit.java2blog.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; /* * This is our model class and it corresponds to Customer table in database */ @Entity @Table(name="CUSTOMER") public class Customer{ @Id @Column(name="id") @GeneratedValue(strategy=GenerationType.IDENTITY) int id; @Column(name="Customer_Name") String customerName; @Column(name="email") String email; public Customer() { super(); } public Customer(String customerName,String email) { super(); this.customerName=customerName; this.email=email; } public String getCustomerName() { return customerName; } public void setCustomerName(String customerName) { this.customerName = customerName; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } public int getId() { return id; } public void setId(int id) { this.id = id; } } |
That’s all about top 40+ hibernate interview questions with answers.
You may also like:
- web services interview questions
- restful web services interview questions
- Data structure and algorithm Interview Questions
- Spring interview questions
- Core java interview questions
- Java Collections interview questions
- Java String interview questions
- OOPs interview questions in java
- Java Multithreading interview questions
- Exceptional handling interview questions in java
- Java Serialization interview questions in java