Table of Contents
In this post, we will see about AngularJS inbuilt filter examples.
AngularJS tutorial:
We need to display output data in some other formats many times . For example: You need to display currency with the number. AngularJS provides many inbuilt filter for this purpose.
AngularJS filters are used to format data in views. “|” symbol is used to apply filter.
AngularJS filter can be applied in two ways
AngularJS filters are used to format data in views. “|” symbol is used to apply filter.
AngularJS filter can be applied in two ways
- On AngularJS directives
- On AngularJS expressions
Lets see some of inbuilt filters here.
Filter on expressions
uppercase
This filter is used to change format of text to uppercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.java2blogMsg = "Hello from Java2blog"; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> {{java2blogMsg|uppercase}} !!! </div> |
Output: HELLO FROM JAVA2BLOG !!!
lowercase
This filter is used to change format of text to lowercase.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.java2blogMsg = "Hello from Java2blog"; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> {{java2blogMsg|lowercase}} !!! </div> |
Output:hello from java2blog !!!
currency
This filter is used to format number into currency.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.cost= 500; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> Cost is {{cost|currency}} </div> |
Output:Cost is $500.00
limitTo
limitTo is used to display limited number of charcters
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.java2blogMsg = "Hello from Java2blog"; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> {{java2blogMsg | limitTo:5}} !!! </div> |
Output:
Hello !!!
date
Date is used to format dates in specific formats
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.date = (new Date()).getTime() ; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> Today 's date : {{date | date:'dd/MM/yyyy'}} </div> |
Output:Today ‘s date : 02/04/2016
Filter on directives
orderby
This filter is use to order by column in the table.
Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.countries= [ {"name": "India" , "capital" : "delhi"}, {"name": "England" , "capital" : "London"}, {"name": "France" , "capital" : "Paris"}, {"name": "Japan" , "capital" : "Tokyo"} ]; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> <table border="1"> <thead> <tr> <th>Name</th> <th>Captial</th> </tr> </thead> <tr ng-repeat="con in countries | orderBy:'name'"> <td>{{ con.name }}</td> <td>{{ con.capital }}</td> </tr> </table> </div> |
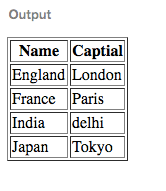
Filter:
It is used to filter data from array or list
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
<meta charset="UTF-8"> <title>Angular js</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0/angular.min.js"></script> <script> var app = angular.module('myApp', []); app.controller('java2blogContoller', function($scope) { $scope.countries= [ {"name": "India" , "capital" : "delhi"}, {"name": "England" , "capital" : "London"}, {"name": "France" , "capital" : "Paris"}, {"name": "Japan" , "capital" : "Tokyo"} ]; }); </script> <div ng-app="myApp" ng-controller="java2blogContoller"> Filter : <input type="text" ng-model="country.name"> <table border="1"> <thead> <tr> <th>Name</th> <th>Captial</th> </tr> </thead> <tr ng-repeat="con in countries | filter: country"> <td>{{ con.name }}</td> <td>{{ con.capital }}</td> </tr> </table> </div> |
AngularJS filter example
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.