Table of Contents
Introduction
So, you sit down, grab a cup of coffee and start programming in Python. Then out of nowhere, this stupid python error shows up: ValueError: math domain error
. 😞
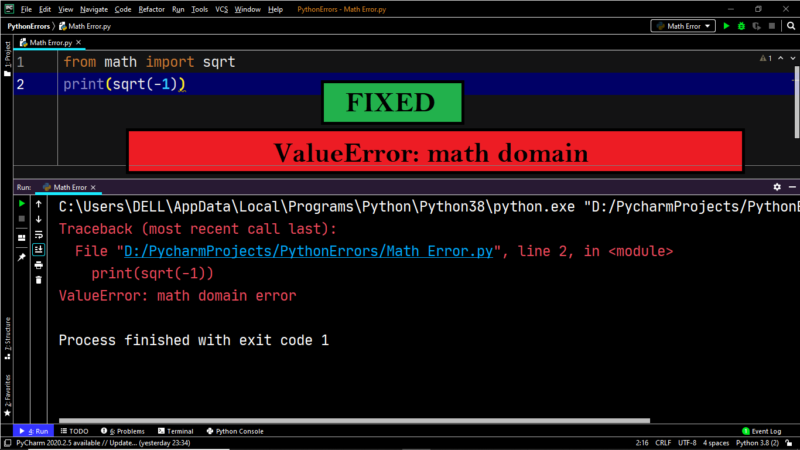
Sometimes it may seem annoying, but once you take time to understand what Math domain error
actually is, you will solve the problem without any hassle.
To fix this error, you must understand – what is meant by the domain of a function?
Let’s use an example to understand “the domain of a function.”
Given equation: y= √(x+4)
- y = dependent variable
- x = independent variable
The domain of the function above is x≥−4
. Here x can’t be less than −4 because other values won’t yield a real output.
❖ Thus, the domain of a function is a set of all possible values of the independent variable (‘x’) that yield a real/valid output for the dependent variable (‘y’).
⚠️What Is a Math Domain Error in Python?
If you have done something that is mathematically undefined (not possible mathematically), then Python throws ValueError: math domain error
.
➥ Fixing “ValueError: math domain error”-sqrt
Example:
1 2 3 4 |
from math import * print(sqrt(-5)) |
Output:
1 2 3 4 5 6 |
Traceback (most recent call last): File "D:/PycharmProjects/PythonErrors/Math Error.py", line 2, in <module> print(sqrt(-5)) ValueError: math domain error |
Explanation:
Calculating the square root of a negative number is outside the scope of Python, and it throws a ValueError
.
Now, let’s dive into the solutions to fix our problem!
💡 Solution 1: Using “cmath” Module
When you calculate the square root of a negative number in mathematics, you get an imaginary number. The module that allows Python to compute the square root of negative numbers and generate imaginary numbers as output is known as cmath
.
Solution:
1 2 3 4 |
from cmath import sqrt print(sqrt(-5)) |
Output:
💡 Solution 2: Use Exception Handling
If you want to eliminate the error and you are not bothered about imaginary outputs, then you can use try-except
blocks. Thus, whenever Python comes across the ValueError: math domain error
it is handled by the except block.
Solution:
1 2 3 4 5 6 7 8 |
from math import * x = int(input('Enter an integer: ')) try: print(sqrt(x)) except ValueError: print("Cannot Compute Negative Square roots!") |
Output:
Enter an integer: -5
Cannot Compute Negative Square roots!
Let us have a look at some other scenarios that lead to the occurrence of the math domain error
and the procedure to avoid this error.
➥ “ValueError: math domain error” Examples
✰ Scenario 1: Math Domain Error While Using pow()
Cause of Error: If you try to calculate a negative base value raised to a fractional power, it will lead to the occurrence of ValueError: math domain error
.
Example:
1 2 3 4 5 |
import math e = -1.7 print(math.pow(-3, e)) |
Output:
Traceback (most recent call last):
File “D:/PycharmProjects/PythonErrors/Math Error.py”, line 3, in
print(math.pow(-3, e))
ValueError: math domain error
Solution: Use the cmath
module to solve this problem.
- Note:
- Xy = ey ln x
Using the above property, the error can be avoided as follows:
1 2 3 4 5 |
from cmath import exp,log e = -1.7 print(exp(e * log(-3))) |
Output:
(0.0908055832509843+0.12498316306449488j)
✰ Scenario 2: Python Math Domain Error While Using log()
Consider the following example if you are working on Python 2.x:
1 2 3 4 |
import math print(2/3*math.log(2/3,2)) |
Output:
Traceback (most recent call last):
File “main.py”, line 2, in
print(2/3*math.log(2/3,2))
ValueError: math domain error
Explanation: In Python 2.x, 2/3 evaluates to 0 since division floors by default. Therefore you’re attempting a log 0, hence the error. Python 3, on the other hand, does floating-point division by default.
Solution:
To Avoid the error try this instead:
from __future__ import division
, which gives you Python 3 division behaviour in Python 2.7.
1 2 3 4 5 6 7 |
from __future__ import division import math print(2/3*math.log(2/3,2)) # Output: -0.389975000481 |
✰ Scenario 3: Math Domain Error While Using asin()
Example:
1 2 3 4 5 6 7 |
import math k = 5 print("asin(",k,") is = ", math.asin(k)) |
Output:
Traceback (most recent call last):
File “D:/PycharmProjects/PythonErrors/rough.py”, line 4, in
print(“asin(“,k,”) is = “, math.asin(k))
ValueError: math domain error
Explanation: math.asin()
method only accepts numbers between the range of -1 to 1. If you provide a number beyond of this range, it returns a ValueError – “ValueError: math domain error
“, and if you provide anything else other than a number, it returns error TypeError – “TypeError: a float is required
“.
Solution: You can avoid this error by passing a valid input number to the function that lies within the range of -1 and 1.
1 2 3 4 5 6 7 8 9 |
import math k = 0.25 print("asin(",k,") is = ", math.asin(k)) #OUTPUT: asin( 0.25 ) is = 0.25268025514207865 |
📖 Exercise: Fixing Math Domain Error While Using Acos()
Note: When you pass a value to math.acos()
which does not lie within the range of -1 and 1, it raises a math domain error
.
Fix the following code:
1 2 3 4 |
import math print(math.acos(10)) |
Conclusion
I hope this article helped you. Please subscribe and stay tuned for more exciting articles in the future. Happy learning! 📚