Table of Contents
This is 1st part of java Serialization tutorial.
Java Serialization Tutorial:
- Serialization in java
- Java Serialization interview questions and answers
- serialversionuid in java
- serializationexternalizable in java
- Transient keyword in java
- Difference between Serializable and Externalizable in Java
Java provides mechanism called serialization to persists java objects in a form of ordered or sequence of bytes that includes the object’s data as well as information about the object’s type and the types of data stored in the object.
So if we need to serialize any object then it can be read and deserialize it using object’s type and other information so we can retrieve original object.
Classes ObjectInputStream and ObjectOutputStream are high-level streams that contain the methods for serializing and deserializing an object.
ObjectOutputStream has many method for serializing object but commonly used method is
1 2 3 4 5 6 |
   private void writeObject(ObjectOutputStream os) throws IOException    {          } |
Similarly ObjectInputStream has
1 2 3 4 5 6 |
   private void readObject(ObjectInputStream is) throws IOException, ClassNotFoundException    {          } |
Need of Serialization in java?
Serialization is usually used when there is a need to send your data over network or to store in files. By data, I mean objects and not text.
Now the problem is your network infrastructure and your Hard disk are hardware components that understand bits and bytes but not Java objects.
Serialization is the translation of Java object’s values/states to bytes to send it over network or to save it.On other hand,Deserialization is conversion of byte code to corresponding java objects.
Concept of serialVersionUID :
For Serialization in java:
steps are :

Lets take an example:
Create Employee.java in src->org.arpit.javapostsforlearning
1.Employee.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
package org.arpit.javapostsforlearning; import java.io.Serializable; public class Employee implements Serializable{Â private static final long serialVersionUID = 1L;Â Â Â Â Â int employeeId; Â Â Â String employeeName; Â Â Â String department; Â Â Â Â Â Â public int getEmployeeId() { Â Â Â Â Â Â return employeeId; Â Â Â } Â Â Â public void setEmployeeId(int employeeId) { Â Â Â Â Â Â this.employeeId = employeeId; Â Â Â } Â Â Â public String getEmployeeName() { Â Â Â Â Â Â return employeeName; Â Â Â } Â Â Â public void setEmployeeName(String employeeName) { Â Â Â Â Â Â this.employeeName = employeeName; Â Â Â } Â Â Â public String getDepartment() { Â Â Â Â Â Â return department; Â Â Â } Â Â Â public void setDepartment(String department) { Â Â Â Â Â Â this.department = department; Â Â Â } } |
Marker interface in Java is interfaces with no field or methods or in simple word empty interface in java is called marker interface
Create SerializeMain.java in src->org.arpit.javapostsforlearning
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
package org.arpit.javapostsforlearning; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectOutputStream; Â public class SerializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { Employee emp = new Employee(); emp.setEmployeeId(101); emp.setEmployeeName("Arpit"); emp.setDepartment("CS"); try { FileOutputStream fileOut = new FileOutputStream("employee.ser"); ObjectOutputStream outStream = new ObjectOutputStream(fileOut); outStream.writeObject(emp); outStream.close(); fileOut.close(); }catch(IOException i) { i.printStackTrace(); } } } |
For Deserialization:
Steps are:

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
package org.arpit.javapostsforlearning; import java.io.IOException; import java.io.ObjectInputStream; public class DeserializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { Employee emp = null; try { FileInputStream fileIn =new FileInputStream("employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); emp = (Employee) in.readObject(); in.close(); fileIn.close(); }catch(IOException i) { i.printStackTrace(); return; }catch(ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getEmployeeName()); System.out.println("Department: " + emp.getDepartment()); } } |
4.Run it:
1 2 3 4 5 6 |
Deserialized Employee... Emp id: 101 Name: Arpit Department: CS |
So we have serialized an employee object and then deserialized it. It seems very simple but it can be very complex when reference object, inheritance come into the picture. So we will see different cases one by one and how we can apply serialization in different scenarios.
Case 1-What if an object has a reference to other objects
We have seen a very simple case of serialization, now what if it also a reference to other objects.
How will it serialize then? will the reference object will also get serialized?.
Yes,You don’t have to explicitly serialize reference objects. When you serialize any object and if it contains any other object reference then Java serialization serializes that object’s entire object graph.
For example Lets say, Employee now has reference to address object and Address can have reference to some other object(e.g.Home) then when you serialize Employee object all other reference objects such as address and home will be automatically serialized. Let’s create Address class and add object of Address as a reference to the above employee class.
Employee.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
package org.arpit.javapostsforlearning; import java.io.Serializable; public class Employee implements Serializable{Â private static final long serialVersionUID = 1L; Â int employeeId; String employeeName; String department; Address address; public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getEmployeeName() { return employeeName; } public void setEmployeeName(String employeeName) { this.employeeName = employeeName; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } } |
Create Address.java in org.arpit.javapostsforlearning
Address.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
package org.arpit.javapostsforlearning; public class Address { int homeNo; String street; String city; Â public Address(int homeNo, String street, String city) { super(); this.homeNo = homeNo; this.street = street; this.city = city; } Â public int getHomeNo() { return homeNo; } public void setHomeNo(int homeNo) { this.homeNo = homeNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package org.arpit.javapostsforlearning; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class SerializeDeserializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { Employee emp = new Employee(); emp.setEmployeeId(101); emp.setEmployeeName("Arpit"); emp.setDepartment("CS"); Address address=new Address(88,"MG road","Pune"); emp.setAddress(address); //Serialize try { FileOutputStream fileOut = new FileOutputStream("employee.ser"); ObjectOutputStream outStream = new ObjectOutputStream(fileOut); outStream.writeObject(emp); outStream.close(); fileOut.close(); }catch(IOException i) { i.printStackTrace(); } //Deserialize emp = null; try { FileInputStream fileIn =new FileInputStream("employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); emp = (Employee) in.readObject(); in.close(); fileIn.close(); }catch(IOException i) { i.printStackTrace(); return; }catch(ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getEmployeeName()); System.out.println("Department: " + emp.getDepartment()); address=emp.getAddress(); System.out.println("City :"+address.getCity()); } } |
1 2 3 4 5 6 7 8 9 |
java.io.NotSerializableException: org.arpit.javapostsforlearning.Address    at java.io.ObjectOutputStream.writeObject0(Unknown Source)    at java.io.ObjectOutputStream.defaultWriteFields(Unknown Source)    at java.io.ObjectOutputStream.writeSerialData(Unknown Source)    at java.io.ObjectOutputStream.writeOrdinaryObject(Unknown Source)    at java.io.ObjectOutputStream.writeObject0(Unknown Source)    at java.io.ObjectOutputStream.writeObject(Unknown Source) |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
import java.io.Serializable; public class Address implements Serializable{ private static final long serialVersionUID = 1L; int homeNo; String street; String city; public Address(int homeNo, String street, String city) { super(); this.homeNo = homeNo; this.street = street; this.city = city; } Â public int getHomeNo() { return homeNo; } public void setHomeNo(int homeNo) { this.homeNo = homeNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } } |
1 2 3 4 5 6 7 |
Deserialized Employee... Emp id: 101 Name: Arpit Department: CS City :Pune |
If you don’t have access to address class then how will you implement serializable interface in Address class.Is there any alternative to that? yes there is,You can create another class which extends address and make it serialzable but It can fails in many cases:
- What if class is declared as final
- What if class have reference to other non serializable object.
So then how will you serialize Employee object? so solution is you can make it transient.If you don’t want to serialize any field then make it transient.
1 2 3 |
transient Address address |
Case 3:What if you still want to save state of reference object(e.g above address object):
If you make address transient then during deserialization it will return null. But what if you still want to have the same state as when you have serialized address object.Java serialization provides a mechanism such that if you have private methods with a particular signature then they will get called during serialization and deserialization so if we provide writeObject and readObject method of employee class and they will be called during serialization and deserialization of Employee object.
Employee.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
package org.arpit.javapostsforlearning; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; public class Employee implements Serializable{Â Â private static final long serialVersionUID = 1L; int employeeId; String employeeName; String department; transient Address address; public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getEmployeeName() { return employeeName; } public void setEmployeeName(String employeeName) { this.employeeName = employeeName; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } public Address getAddress() { return address; } public void setAddress(Address address) { this.address = address; } private void writeObject(ObjectOutputStream os) throws IOException, ClassNotFoundException { try { os.defaultWriteObject(); os.writeInt(address.getHomeNo()); os.writeObject(address.getStreet()); os.writeObject(address.getCity()); } catch (Exception e) { e.printStackTrace(); } } private void readObject(ObjectInputStream is) throws IOException, ClassNotFoundException { try { is.defaultReadObject(); int homeNo=is.readInt(); String street=(String) is.readObject(); String city=(String) is.readObject(); address=new Address(homeNo,street,city); } catch (Exception e) { e.printStackTrace(); } } } |
One thing should be kept in mind that ObjectInputStream should read data in same sequence in which we have written data to ObjectOutputStream.
Create Address.java in org.arpit.javapostsforlearning
Address.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
package org.arpit.javapostsforlearning; import java.io.Serializable; public class Address { int homeNo; String street; String city; public Address(int homeNo, String street, String city) { super(); this.homeNo = homeNo; this.street = street; this.city = city; } public int getHomeNo() { return homeNo; } public void setHomeNo(int homeNo) { this.homeNo = homeNo; } public String getStreet() { return street; } public void setStreet(String street) { this.street = street; } public String getCity() { return city; } public void setCity(String city) { this.city = city; } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package org.arpit.javapostsforlearning; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class SerializeDeserializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { Employee emp = new Employee(); emp.setEmployeeId(101); emp.setEmployeeName("Arpit"); emp.setDepartment("CS"); Address address=new Address(88,"MG road","Pune"); emp.setAddress(address); //Serialize try { FileOutputStream fileOut = new FileOutputStream("employee.ser"); ObjectOutputStream outStream = new ObjectOutputStream(fileOut); outStream.writeObject(emp); outStream.close(); fileOut.close(); }catch(IOException i) { i.printStackTrace(); } //Deserialize emp = null; try { FileInputStream fileIn =new FileInputStream("employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); emp = (Employee) in.readObject(); in.close(); fileIn.close(); }catch(IOException i) { i.printStackTrace(); return; }catch(ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getEmployeeName()); System.out.println("Department: " + emp.getDepartment()); address=emp.getAddress(); System.out.println("City :"+address.getCity()); } } |
When you run SerializeDeserializeMain.java.You will get following output
1 2 3 4 5 6 7 |
Deserialized Employee... Emp id: 101 Name: Arpit Department: CS City :Pune |
Inheritance in Serialization in java:
Now we will see how inheritance affects serialization.So there can be muliple cases whether super class is serializable or not.If not then how will you handle that and how it works.
Let’s see by example.
We will create Person.java which will be superclass of Employee.
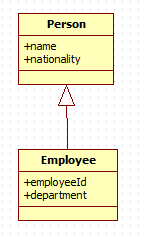
Case 4: What if superclass is Serializable?
If superclass is serialzable then all its subclasses are automatically serializable.
Case 5:What if superclass is not Serializable?
If super class is not serializable then we have to handle it quite differently.
- If superclass is not serializable then it must have no argument constructor.
Person.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
package org.arpit.javapostsforlearning; public class Person { String name="default"; String nationality; public Person() { System.out.println("Person:Constructor"); } public Person(String name, String nationality) { super(); this.name = name; this.nationality = nationality; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getNationality() { return nationality; } public void setNationality(String nationality) { this.nationality = nationality; } } |
Create Employee.java in org.arpit.javapostsforlearning
Employee.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
package org.arpit.javapostsforlearning; import java.io.Serializable; public class Employee extends Person implements Serializable{Â Â private static final long serialVersionUID = 1L; int employeeId; String department; public Employee(int employeeId,String name,String department,String nationality) { super(name,nationality); this.employeeId=employeeId; this.department=department; System.out.println("Employee:Constructor"); } public int getEmployeeId() { return employeeId; } public void setEmployeeId(int employeeId) { this.employeeId = employeeId; } public String getDepartment() { return department; } public void setDepartment(String department) { this.department = department; } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
package org.arpit.javapostsforlearning; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class SerializeDeserializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { //Serialize Employee emp = new Employee(101,"Arpit","CS","Indian"); System.out.println("Before serializing"); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getName()); System.out.println("Department: " + emp.getDepartment()); System.out.println("Nationality: " + emp.getNationality()); System.out.println("************"); System.out.println("Serializing"); try { FileOutputStream fileOut = new FileOutputStream("employee.ser"); ObjectOutputStream outStream = new ObjectOutputStream(fileOut); outStream.writeObject(emp); outStream.close(); fileOut.close(); }catch(IOException i) { i.printStackTrace(); } //Deserialize System.out.println("************"); System.out.println("Deserializing"); emp = null; try { FileInputStream fileIn =new FileInputStream("employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); emp = (Employee) in.readObject(); in.close(); fileIn.close(); }catch(IOException i) { i.printStackTrace(); return; }catch(ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("After serializing"); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getName()); System.out.println("Department: " + emp.getDepartment()); System.out.println("Nationality: " + emp.getNationality()); } } |
When you run SerializeDeserializeMain.java.You will get following output
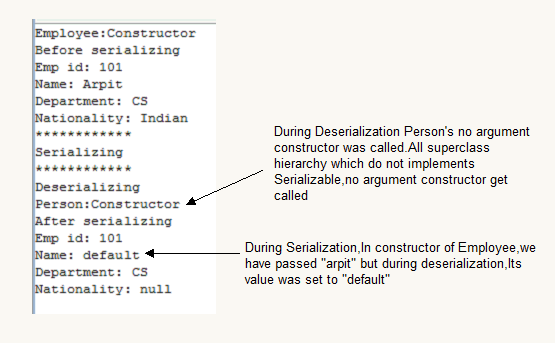
If superclass is not Serializable then all values of the instance variables inherited from super class will be initialized by calling constructor of Non-Serializable Super class during deserialization process. so here name is inherited from person so during deserialization,name is initialized to default.
Case 6-What if superclass is Serializable but you don’t want subclass to be Serializable
If you don’t want subclass to serializable then you need to implement writeObject() and readObject() method and need to throw NotSerializableException from these methods.
Case 7-Can you Serialize static variables?
No, you can’t. As you know static variables are at class level not at object level and you serialize an object so you can’t serialize static variables.
Externalizable interface can be used in place of serializable if you want more control over serialization.You can read more about it at Externalizable in java
Summary:
- Serialization in java is the translation of your Java object’s values/states to bytes to send it over network or save it.On other hand,Deserialization is conversion of byte code to corresponding java objects.
- Good thing about Serialization is entire process is JVM independent, meaning an object can be serialized on one platform and deserialized on an entirely different platform.
- If you want to serialize any class then it must implement Serializable interface which is marker interface.
- Marker interface in Java is interface with no field or methods or in simple word empty interface in java is called marker interface
- serialVersionUID is used to ensure that same object(That was used during Serialization) is loaded during Deserialization.serialVersionUID is used for version control of object.
- When you serialize any object and if it contain any other object reference then Java serialization serialize that object’s entire object graph.
- If you don’t want to serialize any field,then make it transient.
- If superclass is Serializable then its subclasses are automatically Serializable.
- If superclass is not Serializable then all values of the instance variables inherited from super class will be initialized by calling constructor of Non-Serializable Super class during deserialization process.
- If you don’t want subclass to serializable then you need to implement writeObject() and readObject() method and need to throw NotSerializableException from these methods.
- You can’t serialize static variables.
That’s all about Serialization in java.
thank you for this article
please give one article about NORMALIZATION
Thanks Shubhra.I will soon do that 🙂
Don't you think you should provide
serialVersionUID
in the class' in your example. IMHO always have an serialVersionUID in class' who implements Serializable.Hello Harsh,
Yes you are right.I forgot to do so.Thanks for your suggestion.I have updated article
excellent Serialization info provided
btw you can add one section in it about “Externalizable” interface.
awesome but i want more information related to core java
Hi, I am searching how the serialisation works in java.I have got your support.Thanks….
Theosoft
Amazing tutorial! Thanks!
That is what I was looking for
thanks you so much for this great article, best regards from Argentina.
How can we serialize an object have non serialize property which is not accessible by us,because that property is coming from service.i.e. some Enum used by sevice and we are unable to serilize the same though my object implemts serilizable interface.
Please suggest.
very helpful tutorial.
best regards
you explained very clearly.
Hi, am new to JAVA World and I have read many posts and articles regarding the serialization in java, but get understand after reading this post.. Can you please post some more topics related to CORE JAVA (i.e OOPS Concepts, multithreading , Synchronization)…
If so , then it would be very helpful to me as well as to others…
Thank you…
helpfl!! thaks !! i am writing same in exam tomoorrow if asked!!
Great website.
The code snippets are not visible in browsers any more.
Could you please fix that.
Thanks