In this post, we will some important interview questions specifically on Java 8. Java has changed a lot over years and Java 8 has introduced many new features which you need to know when you are preparing for Java interview.
Here is a list of most asked Java 8 interview questions.
Table of Contents
- 1) What are new features which got introduced in Java 8?
- 2) What are main advantages of using Java 8?
- 3) What is lambda expression?
- 4) Can you explain the syntax of Lambda expression?
- 5) What are functional interfaces?
- 6) How lambda expression and functional interfaces are related?
- 7) Can you create your own functional interface?
- 8) What is method reference in java 8?
- 9) What is Optional? Why and how can you use it?
- 10) What are defaults methods?
- 11) What is the difference between Predicate and Function?
- 12) Are you aware of Date and Time API introduced in Java 8? What the issues with Old Date and time API?
- 13) Can you provide some APIs of Java 8 Date and TIme?
- 14) How will you get current date and time using Java 8 Date and TIme API?
- 15) Do we have PermGen in Java 8? Are you aware of MetaSpace?
- 16) Given a list of employees, you need to filter all the employee whose age is greater than 20 and print the employee names.(Java 8 APIs only)
- 17) Given the list of employees, count number of employees with age 25?
- 18) Given the list of employees, find the employee with name “Mary”.
- 19)Given a list of employee, find maximum age of employee?
- 20) Given a list of employees, sort all the employee on the basis of age? Use java 8 APIs only
- 21) Join the all employee names with “,” using java 8?
- 22) Given the list of employee, group them by employee name?
- 23) Difference between Intermediate and terminal operations in Stream?
- 24) Given the list of numbers, remove the duplicate elements from the list.
- 25) Difference between Stream’s findFirst() and findAny()?
- 26) Given a list of numbers, square them and filter the numbers which are greater 10000 and then find average of them.( Java 8 APIs only)
- 27) What is use of Optional in Java 8?
- 28) What is predicate function interface?
- 29) What is consumer function interface?
- 30) What is supplier function interface?
1) What are new features which got introduced in Java 8?
There are lots of new features which were added in Java 8. Here is the list of important features:
- Lambda Expression
- Stream API
- Default methods in the interface
- Functional Interface
- Optional
- Method reference
- Date API
- Nashorn, JavaScript Engine
2) What are main advantages of using Java 8?
- More compact code
- Less boiler plate code
- More readable and reusable code
- More testable code
- Parallel operations
3) What is lambda expression?
Lambda expression is an anonymous function that has a set of parameters, a lambda (->) symbol, and a function body. Essentially, it is a function without a name.
Structure of Lambda Expressions
1 2 3 4 |
(Argument List) ->{expression;} or (Argument List) ->{statements;} |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
public class ThreadSample { public static void main(String[] args) { // old way new Thread(new Runnable() { @Override public void run() { System.out.println("Thread is started"); } }).start(); // using lambda Expression new Thread(()->System.out.println("Thread is started")).start(); } } |
You can refer to lambda expression in java for more details.
4) Can you explain the syntax of Lambda expression?
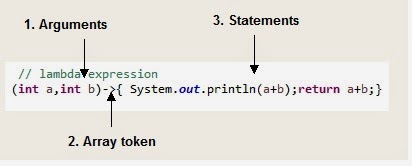
1 2 3 4 5 |
()->{System.out.println("Hello")}; //Without argument, will print hello (int a)->{System.out.println(a)} //; One argument, will print value of a (int a,int b)-> {a+b};//two argument, will return sum of these two integers |
1 2 3 |
(a,b)->{a+b};//two argument, will return sum of these two numbers |
1 2 3 |
(int a,b)->{a+b};//Compilation error |
1 2 3 |
a->{System.out.println(a)}; // Will print value of number a |
3. Body
- The body can contain either an expression or statements.
- If the body contains only one statement, curly braces are not needed, and the return type of the anonymous function matches that of the body expression.
- If there are multiple statements, they must be enclosed in curly braces, and the return type of the anonymous function corresponds to the value returned from the code block, or void if nothing is returned.
5) What are functional interfaces?
Functional interfaces are those interfaces that can have only one abstract method. They can include static methods, default methods, or even override methods from the Object class.
There are many functional interfaces already present in java such as Comparable, Runnable.
Since Runnable has only one method, it is considered a functional interface.
You can read more about the functional interface.
Lambda expressions can be applied only to the abstract method of a functional interface.
For example
Runnable has only one abstract method named run()
, so it can be used as follows:
1 2 3 4 5 6 |
// Using lambda expression Thread t1=new Thread( ()->System.out.println("In Run method") ); |
Here, we are using the Thread constructor that takes a Runnable as a parameter. As you can see, we did not specify any function name here. Since Runnable has only one abstract method, Java will implicitly create an anonymous Runnable and execute the run method.
It will be as good as below code:
1 2 3 4 5 6 7 8 |
Thread t1=new Thread(new Runnable() { @Override public void run() { System.out.println("In Run method"); } }); |
7) Can you create your own functional interface?
Yes, you can create your own functional interface. Although Java can implicitly identify a functional interface, you can also annotate it with @FunctionalInterface to explicitly indicate its purpose.
Example:
Create interface named Printable
as below:
1 2 3 4 5 6 7 8 9 10 11 12 |
package org.arpit.java2blog; public interface Printable { void print(); default void printColor() { System.out.println("Printing Color copy"); } } |
Create main class named "FunctionalIntefaceMain"
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
package org.arpit.java2blog.constructor; public class FunctionalIntefaceMain { public static void main(String[] args) { FunctionalIntefaceMain pMain=new FunctionalIntefaceMain(); pMain.printForm(() -> System.out.println("Printing form")); } public void printForm(Printable p) { p.print(); } } |
When you run above program, you will get below output:
As you can see, because Printable
has only one abstract method called print(), we were able to invoke it using a lambda expression.
8) What is method reference in java 8?
Method reference is used refer method of functional interface. It is simply a more compact form of a lambda expression. You can replace a lambda expression with a method reference.
Syntax:
class::methodname
9) What is Optional? Why and how can you use it?
Java 8 introduced a new class called Optional
, primarily to avoid NullPointerException in Java. The Optional class encapsulates an optional value that may or may not be present. It acts as a wrapper around an object and can be used to prevent NullPointerExceptions.
Let’s take a simple example:
You have written below function to get first non repeated character in String.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
public static Character getNonRepeatedCharacter(String str) { Map<Character, Integer> countCharacters = new LinkedHashMap<Character, Integer>(); for (int i = 0; i < str.length() - 1; i++) { Character c = str.charAt(i); if (!countCharacters.containsKey(c)) { countCharacters.put(c, 1); } else { countCharacters.put(c, countCharacters.get(c) + 1); } } // As LinkedHashMap maintains insertion order, first character with // count 1 should return first non repeated character for (Entry<Character, Integer> e : countCharacters.entrySet()) { if (e.getValue() == 1) return e.getKey(); } return null; } |
You call above method as below:
1 2 3 4 |
Character c=getNonRepeatedCharacter("SASAS"); System.out.println("Non repeated character is :"+c.toString()); |
Do you see the problem? There is no non-repeating character in getNonRepeatedCharacter("SASAS")
; hence, it will return null. When calling c.toString()
, so it will obviously throw a NullPointerException.
You can use Optional to avoid this NullPointerException. Let’s modify the method to return an Optional
object rather than a String:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
public static Optional<Character> getNonRepeatedCharacterOpt(String str) { Map<Character, Integer> countCharacters = new LinkedHashMap<Character, Integer>(); for (int i = 0; i < str.length(); i++) { Character c = str.charAt(i); if (!countCharacters.containsKey(c)) { countCharacters.put(c, 1); } else { countCharacters.put(c, countCharacters.get(c) + 1); } } // As LinkedHashMap maintains insertion order, first character with // count 1 should return first non repeated character for (Entry<Character, Integer> e : countCharacters.entrySet()) { if (e.getValue() == 1) return Optional.of(e.getKey()); } return Optional.ofNullable(null); } |
When above method returned Optional, you are already aware that it can return null value too.
You can call Optional’s isPresent method to check if there is any value wrapped in Optional.
1 2 3 4 5 6 7 8 9 |
Optional<Character> opCh=getNonRepeatedCharacterOpt("SASAS"); if(opCh.isPresent()) System.out.println("Non repeated character is :"+opCh.toString()); else { System.out.println("No non repeated character found in String"); } |
If no value is present in Optional, it will simply print "No non-repeating character found in the string."
10) What are defaults methods?
Default methods are those methods in an interface that have a body and use the default
keyword. They were introduced in Java 8, mainly for backward compatibility.
You can refer to default method in java for more details.
11) What is the difference between Predicate and Function?
Both are functional interfaces.Predicate<T> is a single-argument function that returns either true or false. It can be used as the assignment target for a lambda expression or method reference.
Function<T, R>
is a single-argument function that returns an object. Here, T denotes the type of input to the function, and R denotes the type of result. This can also be used as the assignment target for a lambda expression or method reference.
12) Are you aware of Date and Time API introduced in Java 8? What the issues with Old Date and time API?
Issues with old Date and TIme API:
Thread Safety:You might already be aware that java.util.Date
is mutable and not thread-safe. Similarly, java.text.SimpleDateFormat
is also not thread-safe. The new Java 8 date and time APIs are thread-safe.
Performance: Java 8 ‘s new APIs are better in performance than old Java APIs.
More Readable: Old APIs such Calendar and Date are poorly designed and hard to understand. Java 8 Date and Time APIs are easy to understand and comply with ISO standards.
13) Can you provide some APIs of Java 8 Date and TIme?
LocalDate, LocalTime, and LocalDateTime are the Core API classes for Java 8. As the name suggests, these classes are local to context of observer. It denotes current date and time in context of Observer.
14) How will you get current date and time using Java 8 Date and TIme API?
You can simply use now method of LocalDate to get today’s date.
1 2 3 4 |
LocalDate currentDate = LocalDate.now(); System.out.println(currentDate); |
It will give you output in below format:
You can use now() method of LocalTime to get current time.
1 2 3 4 |
LocalTime currentTime = LocalTime.now(); System.out.println(currentTime); |
It will give you output in below format:
15) Do we have PermGen in Java 8? Are you aware of MetaSpace?
Until Java 7, the JVM used an area called PermGen to store classes. This was removed in Java 8 and replaced by MetaSpace.
Major advantage of MetaSpace over permgen:
PermGen had a fixed maximum size and could not grow dynamically, but MetaSpace can grow dynamically and does not have any size constraints.
Next 7 questions will be based on below class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
package org.arpit.java2blog; public class Employee { private String name; private int age; public Employee(String name, int age) { super(); this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String toString() { return "Employee Name: "+name+" age: "+age; } } |
16) Given a list of employees, you need to filter all the employee whose age is greater than 20 and print the employee names.(Java 8 APIs only)
Answer:
You can simply do it using below statement:
1 2 3 4 5 6 |
List<String> employeeFilteredList = employeeList.stream() .filter(e->e.getAge()>20) .map(Employee::getName) .collect(Collectors.toList()); |
Complete main program for above logic:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
package org.arpit.java2blog; import java.util.ArrayList; import java.util.List; import java.util.stream.Collectors; public class MaximumUsingStreamMain { public static void main(String args[]) { List<Employee> employeeList = createEmployeeList(); List<String> employeeFilteredList = employeeList.stream() .filter(e->e.getAge()>20) .map(Employee::getName) .collect(Collectors.toList()); employeeFilteredList.forEach((name)-> System.out.println(name)); } public static List<Employee> createEmployeeList() { List<Employee> employeeList=new ArrayList<>(); Employee e1=new Employee("John",21); Employee e2=new Employee("Martin",19); Employee e3=new Employee("Mary",31); Employee e4=new Employee("Stephan",18); Employee e5=new Employee("Gary",26); employeeList.add(e1); employeeList.add(e2); employeeList.add(e3); employeeList.add(e4); employeeList.add(e5); return employeeList; } } |
17) Given the list of employees, count number of employees with age 25?
Answer:
You can use a combination of filter()
and count()
to find this.
1 2 3 4 5 6 7 |
List<Employee> employeeList = createEmployeeList(); long count = employeeList.stream() .filter(e->e.getAge()>25) .count(); System.out.println("Number of employees with age 25 are : "+count); |
18) Given the list of employees, find the employee with name “Mary”.
Answer:
The logic is quite simple; modify the main function in the above class as follows:
1 2 3 4 5 6 7 8 |
List<Employee> employeeList = createEmployeeList(); Optional<Employee> e1 = employeeList.stream() .filter(e->e.getName().equalsIgnoreCase("Mary")).findAny(); if(e1.isPresent()) System.out.println(e1.get()); |
19)Given a list of employee, find maximum age of employee?
Answer:
The logic is quite simple; modify the main function in the above class as follows:
1 2 3 4 5 6 7 8 |
List<Employee> employeeList = createEmployeeList(); OptionalInt max = employeeList.stream(). mapToInt(Employee::getAge).max(); if(max.isPresent()) System.out.println("Maximum age of Employee: "+max.getAsInt()); |
20) Given a list of employees, sort all the employee on the basis of age? Use java 8 APIs only
You can simply use the sort()
method of the list to sort the list of employees.
1 2 3 4 5 |
List<Employee> employeeList = createEmployeeList(); employeeList.sort((e1,e2)->e1.getAge()-e2.getAge()); employeeList.forEach(System.out::println); |
21) Join the all employee names with “,” using java 8?
Answer:
1 2 3 4 5 6 7 8 9 |
List<Employee> employeeList = createEmployeeList(); List<String> employeeNames = employeeList .stream() .map(Employee::getName) .collect(Collectors.toList()); String employeeNamesStr = String.join(",", employeeNames); System.out.println("Employees are: "+employeeNamesStr); |
Output:
22) Given the list of employee, group them by employee name?
Answer:
You can use Collections.groupBy() to group list of employees by employee name.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
package org.arpit.java2blog; import java.util.ArrayList; import java.util.List; import java.util.Map; import java.util.stream.Collectors; public class MaximumUsingStreamMain { public static void main(String args[]) { List<Employee> employeeList = createEmployeeList(); Map<String, List<Employee>> map = employeeList.stream() .collect(Collectors.groupingBy(Employee::getName)); map.forEach((name,employeeListTemp)->System.out.println("Name: "+name+" ==>"+employeeListTemp)); } public static List<Employee> createEmployeeList() { List<Employee> employeeList=new ArrayList<>(); Employee e1=new Employee("John",21); Employee e2=new Employee("Martin",19); Employee e3=new Employee("Mary",31); Employee e4=new Employee("Mary",18); Employee e5=new Employee("John",26); employeeList.add(e1); employeeList.add(e2); employeeList.add(e3); employeeList.add(e4); employeeList.add(e5); return employeeList; } } |
Output:
23) Difference between Intermediate and terminal operations in Stream?
Answer:
Java 8 Streams support both intermediate and terminal operation.
Intermediate operations are lazy in nature and are not executed immediately. Terminal operations, on the other hand, are not lazy and are executed as soon as they are encountered. An intermediate operation is memorized and is called when a terminal operation is invoked.
All intermediate operations return a stream, as they transform one stream into another, while terminal operations do not.
Example of Intermediate operations are:
map(Function)
flatmap(Function)
sorted(Comparator)
distinct()
limit(long n)
skip(long n)
Example of terminal operations are :
toArray
reduce
collect
min
max
count
anyMatch
allMatch
noneMatch
findFirst
findAny
24) Given the list of numbers, remove the duplicate elements from the list.
Answer:
You can simply use a stream and then collect it into a set using the Collections.toSet()
method.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
package org.arpit.java2blog; import java.util.Arrays; import java.util.List; import java.util.Set; import java.util.stream.Collectors; public class RemoveDuplicatesFromListMain { public static void main(String[] args) { Integer[] arr=new Integer[]{1,2,3,4,3,2,4,2}; List<Integer> listWithDuplicates = Arrays.asList(arr); Set<Integer> setWithoutDups = listWithDuplicates.stream().collect(Collectors.toSet()); setWithoutDups.forEach((i)->System.out.print(" "+i)); } } |
You can also use distinct to avoid duplicates, as follows:
1 2 3 4 5 6 |
Integer[] arr=new Integer[]{1,2,3,4,3,2,4,2}; List<Integer> listWithDuplicates = Arrays.asList(arr); List<Integer> listWithoutDups = listWithDuplicates.stream().distinct().collect(Collectors.toList()); listWithoutDups.forEach((i)->System.out.print(" "+i)); |
25) Difference between Stream’s findFirst() and findAny()?
findFirst
will always return the first element from the stream, whereas findAny
is allowed to choose any element from the stream. findFirst
exhibits deterministic behavior, whereas findAny
has nondeterministic behavior.
26) Given a list of numbers, square them and filter the numbers which are greater 10000 and then find average of them.( Java 8 APIs only)
Answer:
You can use the map function to square the numbers and then apply a filter to exclude numbers that are less than 10,000. We will use average as the terminating function in this case.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
package org.arpit.java2blog; import java.util.Arrays; import java.util.List; import java.util.OptionalDouble; public class RemoveDuplicatesFromListMain { public static void main(String[] args) { Integer[] arr=new Integer[]{100,24,13,44,114,200,40,112}; List<Integer> list = Arrays.asList(arr); OptionalDouble average = list.stream() .mapToInt(n->n*n) .filter(n->n>10000) .average(); if(average.isPresent()) System.out.println(average.getAsDouble()); } } |
output:
27) What is use of Optional in Java 8?
Answer:
Java 8’s Optional can be used to avoid NullPointerException. You can read the detailed tutorial for more information.
28) What is predicate function interface?
Answer:
A Predicate
is a single-argument function that returns true or false. It has a test method that returns a boolean. When we use filter in the above example, we are actually passing a Predicate
functional interface to it.
29) What is consumer function interface?
Answer:
A Consumer
is a single-argument functional interface that does not return any value. When we use forEach
in the above example, we are actually passing a Consumer functional interface to it.
30) What is supplier function interface?
Answer:
A Supplier
is a functional interface that does not take any parameters but returns a value using the get()
method.
You may also like other interview questions:
- Top 100+ Java coding interview questions
- Core java interview questions
- Java Collections interview questions
- Java Multithreading interview questions
- Java String interview questions
- OOPs interview questions in java
- Exceptional handling interview questions in java
- Java Serialization interview questions in java
- Method overloading and overriding interview questions
- Spring interview questions
- Hibernate interview questions
- Spring boot interview questions
- web services interview questions
- restful web services interview questions