In this post, we will see about Indexerror: list Index Out of Range
in Python.
While working with list, you can access its elements by its index. You will get this error when you are trying to access an index that does not exist.
Let’s see with the help of example.
1 2 3 4 5 |
countryList=["India","China","Nepal","Bhutan"] print(countryList[3]) print(countryList[4]) |
Output:
1 2 3 4 5 6 7 8 9 10 11 12 |
Bhutan --------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-6-c6cdb4c86747> in <module> 1 countryList=["India","China","Nepal","Bhutan"] 2 print(countryList[3]) ----> 3 print(countryList[4]) IndexError: list index out of range </module></ipython-input-6-c6cdb4c86747> |
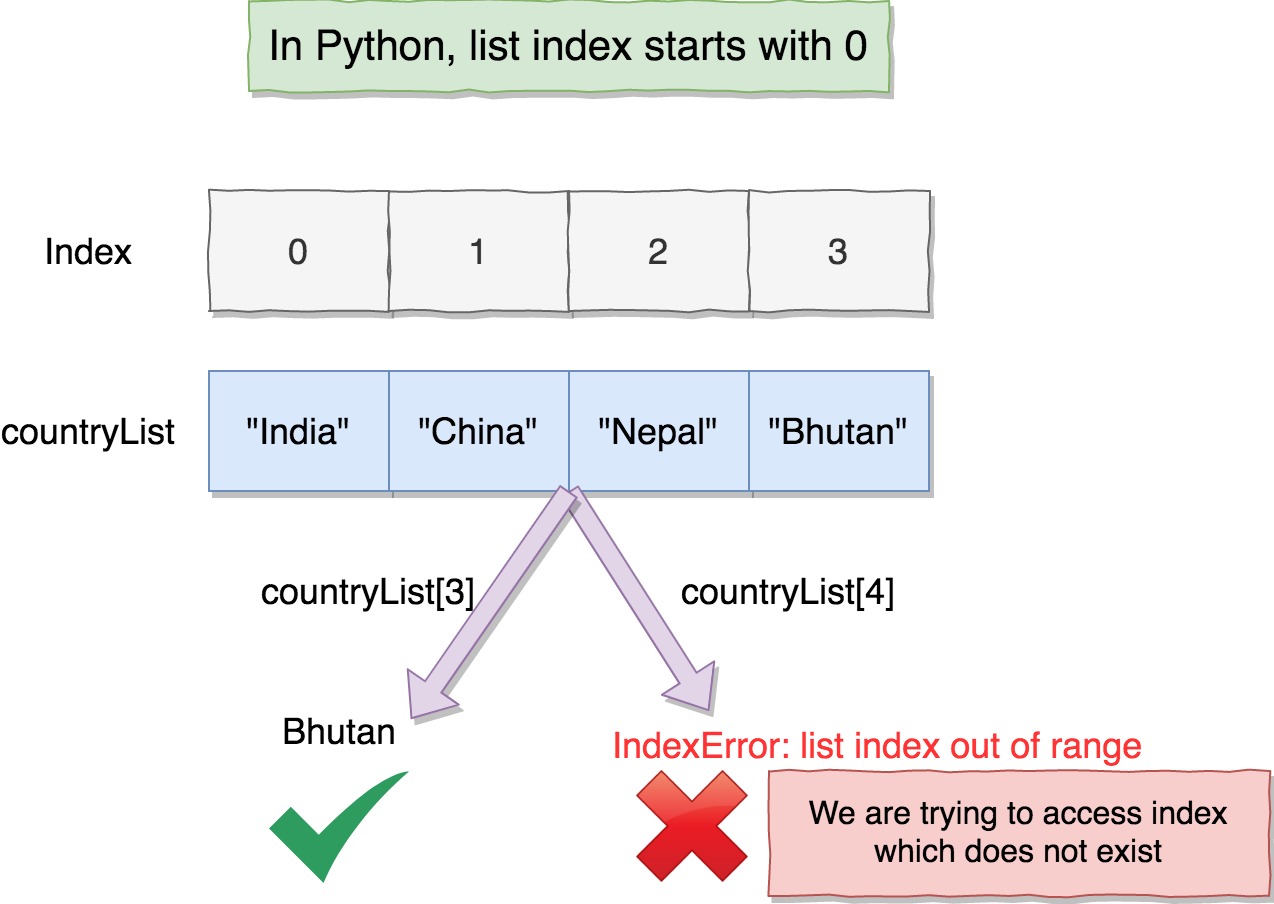
You will generally get this error while working with while
or for
loop in Python.
Let’s understand few common scenarios.
While loop
When you use while loop, you might get Indexerror: list Index Out of Range
.
Let’s understand with help of example:
1 2 3 4 5 6 7 8 |
colorList = ["Orange","Blue","Red","Pink"] print("colorList length",len(colorList)) i=0 while i <= len(colorList): print("Index:",i,"Element:", colorList[i]) i+=1 |
When you run above program, you will get below output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
4 Index: 0 Element: Orange Index: 1 Element: Blue Index: 2 Element: Red Index: 3 Element: Pink --------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-9-c07c56d30f4e> in <module> 3 i=0 4 while i <= len(colorList): ----> 5 print("Index:",i,"Element:", colorList[i]) 6 i+=1 7 IndexError: list index out of range </module></ipython-input-9-c07c56d30f4e> |
Can you guess why did we get IndexError: list index out of range
?
As we have already printed all the elements of the list and we are trying to access colorList[4]
, we are getting indexError
.
Hint: We have issue in below line.
while i <= len(colorList):
We should be iterating from 0 to 3, rather than 0 to 4. In python, list index starts from 0, so we need to change while i <= len(colorList):
to while i < len(colorList):
1 2 3 4 5 6 7 8 |
colorList = ["Orange","Blue","Red","Pink"] print("colorList length",len(colorList)) i=0 while i < len(colorList): print("Index:",i,"Element:", colorList[i]) i+=1 |
When you run above program, you will get below output:
Index: 0 Element: Orange
Index: 1 Element: Blue
Index: 2 Element: Red
Index: 3 Element: Pink
Similarly, you can get this issue in for
loop as well.
Recommended way for iterating with index
If you want to iterate with index over loop, you should use enumerate() function.
Here is the example for the same.
1 2 3 4 5 |
colorList = ["Orange","Blue","Red","Pink"] for idx, color in enumerate(colorList): print("Index:",idx,"Element:", color) |
Index: 1 Element: Blue
Index: 2 Element: Red
Index: 3 Element: Pink
As you can see, we don’t have to deal with len(colorList)
here. In this case, you won’t get Indexerror: list Index Out of Range
Accessing element as index
Another reason for this error may be when you are trying to access elements as index.
Let’s see with the help of example.
1 2 3 4 5 |
listInt = [7,1,5,4,3] for i in listInt: print(listInt[i]) |
Output:
1 2 3 4 5 6 7 8 9 10 11 |
--------------------------------------------------------------------------- IndexError Traceback (most recent call last) <ipython-input-12-3f8a39f792a8> in <module> 1 listInt = [7,1,5,4,3] 2 for i in listInt: ----> 3 print(listInt[i]) IndexError: list index out of range </module></ipython-input-12-3f8a39f792a8> |
As you can see, i
actually refers to element and we are trying to access as index.That’s why we are getting this error.
To resolve this issue, you just need to print i
rather than listInt[i]
That’s all about Indexerror: list Index Out of Range in Python.