In this tutorial, we will see how to read a video and start a webcam using python open-cv, which exists as cv2 (computer vision) library.
Table of Contents
VideoCapture()
method of cv2
library to read and start live streaming. To use cv2 library, you need to import cv2 library using import statement
.
Now let’s see the syntax and return value of cv2 canny()
method first, then we will move on to the examples.
Syntax
1 2 3 |
cv2.VideoCapture(video_path or device index ) |
Parameters
There is one argument which needs to pass in VideoCapture() class.
video_path: Location
video in your system in string form with their extensions like .mp4, .avi, etc.
OR
device index:
It is just the number to specify the camera. Its possible values ie either 0 or -1.
Return value
Video capture object.
cv2 VideoCapture() method examples
Now Let’s see the Python code :
Example 1: Run a given video file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
# import computer vision library(cv2) in this code import cv2 # main code if __name__ == "__main__" : # mentioning absolute path of the video video_path = "C:\\Users\\user\\Desktop\\test_video.mp4" # creating a video capture object video_object = cv2.VideoCapture(video_path) # handling errors # if any erros is occured during the running of the program # then it handles try : # run a infinite loop while(True) : # read a video frame by frame # read() returns tuple in which 1st item is boolean value # either True or False and 2nd item is frame of the video. # read() returns False when video is ended so # no frame is readed and error will be generated. ret,frame = video_object.read() # show the frame on the newly created image window cv2.imshow('Frames',frame) # this condition is used to run a frames at the interval of 10 mili sec # and if in b/w the frame running , any one want to stop the execution . if cv2.waitKey(10) & 0xFF == ord('q') : # break out of the while loop break except : # if any error occurs then this block of code will run print("Video has ended..") |
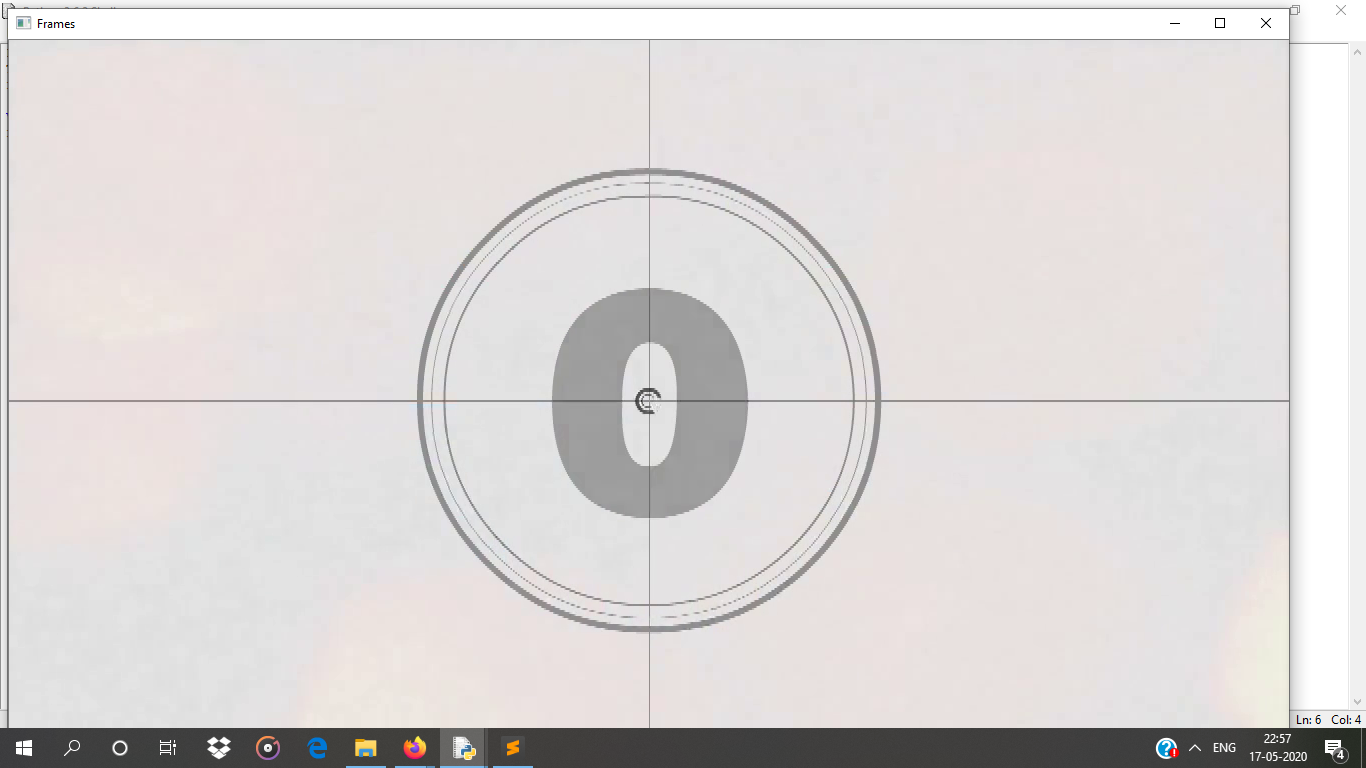
Example 2: Start a webcam.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
# import computer vision library(cv2) in this code import cv2 # main code if __name__ == "__main__" : # creating a video capture object # argument 0 , webcam start video_object = cv2.VideoCapture(0) # handling errors # if any erros is occured during the running of the program # then it handles try : # run a infinite loop while(True) : # read a video frame by frame # read() returns tuple in which 1st item is boolean value # either True or False and 2nd item is frame of the video. # read() returns False when live video is ended so # no frame is readed and error will be generated. ret,frame = video_object.read() # show the frame on the newly created image window cv2.imshow('Frames',frame) # this condition is used to run a frames at the interval of 10 mili sec # and if in b/w the frame running , any one want to stop the execution . if cv2.waitKey(10) & 0xFF == ord('q') : # break out of the while loop break except : # if error occur then this block of code is run print("Video has ended..") |
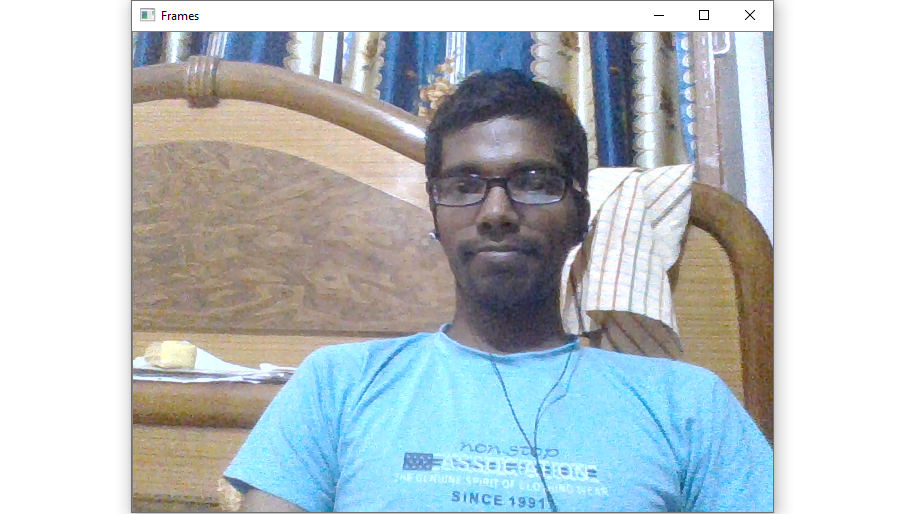
That’s all about Python cv2 VideoCapture() method,
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.