Table of Contents
We will use following annotations for CRUD operation.
Method
|
Description
|
Get
|
It is used to read resource
|
Post
|
It is used to create new resource.
It is not idempotent method |
Put
|
It is generally used to update resource.It is idempotent method
|
Delete
|
It is used to delete resource
|
Idempotent means result of multiple successful request will not change state of resource after initial application
For example :
Delete is idempotent method because when you first time use delete, it will delete the resource (initial application) but after that, all other request will have no result because resource is already deleted.
Post is not idempotent method because when you use post to create resource , it will keep creating resource for each new request, so result of multiple successful request will not be same.
Source code:
Here are steps to create a Spring Restful web services with hibernate integration.
Maven dependencies
2)Â We need to add Jackson json utility in the classpath.
1 2 3 4 5 6 7 |
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> |
Now change pom.xml as follows:
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringRestHibernateExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringRestHibernateExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> <!-- Hibernate --> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>${hibernate.version}</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${hibernate.version}</version> </dependency> <!-- Apache Commons DBCP --> <dependency> <groupId>commons-dbcp</groupId> <artifactId>commons-dbcp</artifactId> <version>1.4</version> </dependency> <!-- Spring ORM --> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>${spring.version}</version> </dependency> <!-- AspectJ --> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjrt</artifactId> <version>${org.aspectj-version}</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.6</version> </dependency> </dependencies> <build> <finalName>SpringRestHibernateExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <configuration> <failOnMissingWebXml>false</failOnMissingWebXml> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <security.version>4.0.3.RELEASE</security.version> <jdk.version>1.7</jdk.version> <hibernate.version>4.3.5.Final</hibernate.version> <org.aspectj-version>1.7.4</org.aspectj-version> </properties> </project> |
Please change context:component-scan if you want to use different package for spring to search for controller.Please refer to spring mvc hello world example for more understanding.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
<?xml version="1.0" encoding="UTF-8"?> <beans:beans xmlns="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd"> <annotation-driven /> <resources mapping="/resources/**" location="/resources/" /> <beans:bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close"> <beans:property name="driverClassName" value="com.mysql.jdbc.Driver" /> <beans:property name="url" value="jdbc:mysql://localhost:3306/CountryData" /> <beans:property name="username" value="root" /> <beans:property name="password" value="" /> </beans:bean> <!-- Hibernate 4 SessionFactory Bean definition --> <beans:bean id="hibernate4AnnotatedSessionFactory" class="org.springframework.orm.hibernate4.LocalSessionFactoryBean"> <beans:property name="dataSource" ref="dataSource" /> <beans:property name="annotatedClasses"> <beans:list> <beans:value>org.arpit.java2blog.model.Country</beans:value> </beans:list> </beans:property> <beans:property name="hibernateProperties"> <beans:props> <beans:prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect </beans:prop> <beans:prop key="hibernate.show_sql">true</beans:prop> </beans:props> </beans:property> </beans:bean> <context:component-scan base-package="org.arpit.java2blog" /> <tx:annotation-driven transaction-manager="transactionManager"/> <beans:bean id="transactionManager" class="org.springframework.orm.hibernate4.HibernateTransactionManager"> <beans:property name="sessionFactory" ref="hibernate4AnnotatedSessionFactory" /> </beans:bean> </beans:beans> |
dataSource bean is used to specify java data source. We need to provide driver, URL , Username and Password.
transactionManager bean is used to configure hibernate transaction manager. hibernate4AnnotatedSessionFactory bean is used to configure FactoryBean that creates a Hibernate SessionFactory. This is the common way to set up a shared Hibernate SessionFactory in a Spring application context, so you can use this SessionFactory to inject in Hibernate data access objects.
Create bean class
4)Â Create a bean name “Country.java” in org.arpit.java2blog.bean.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 |
package org.arpit.java2blog.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; /* * This is our model class and it corresponds to Country table in database */ @Entity @Table(name="COUNTRY") public class Country{ @Id @Column(name="id") @GeneratedValue(strategy=GenerationType.IDENTITY) int id; @Column(name="countryName") String countryName; @Column(name="population") long population; public Country() { super(); } public Country(int i, String countryName,long population) { super(); this.id = i; this.countryName = countryName; this.population=population; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } public long getPopulation() { return population; } public void setPopulation(long population) { this.population = population; } } |
@Entity is used for making a persistent pojo class.For this java class,you will have corresponding table in database. @Column is used to map annotated attribute to corresponding column in table. So Create Country table in mysql database with following code:
1 2 3 4 5 6 7 8 9 |
CREATE TABLE COUNTRY ( id int PRIMARY KEY NOT NULL AUTO_INCREMENT, countryName varchar(100) NOT NULL, population int NOT NULL ) ; |
Create Controller
5) Create a controller named “CountryController.java” in package org.arpit.java2blog.controller
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
package org.arpit.java2blog.controller; import java.util.List; import org.arpit.java2blog.model.Country; import org.arpit.java2blog.service.CountryService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; @RestController public class CountryController { @Autowired CountryService countryService; @RequestMapping(value = "/getAllCountries", method = RequestMethod.GET, headers = "Accept=application/json") public List getCountries() { List listOfCountries = countryService.getAllCountries(); return listOfCountries; } @RequestMapping(value = "/getCountry/{id}", method = RequestMethod.GET, headers = "Accept=application/json") public Country getCountryById(@PathVariable int id) { return countryService.getCountry(id); } @RequestMapping(value = "/addCountry", method = RequestMethod.POST, headers = "Accept=application/json") public void addCountry(@RequestBody Country country) { countryService.addCountry(country); } @RequestMapping(value = "/updateCountry", method = RequestMethod.PUT, headers = "Accept=application/json") public void updateCountry(@RequestBody Country country) { countryService.updateCountry(country); } @RequestMapping(value = "/deleteCountry/{id}", method = RequestMethod.DELETE, headers = "Accept=application/json") public void deleteCountry(@PathVariable("id") int id) { countryService.deleteCountry(id); } } |
Create DAO class
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
package org.arpit.java2blog.dao; import java.util.List; import org.arpit.java2blog.model.Country; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Repository; @Repository public class CountryDAO { @Autowired private SessionFactory sessionFactory; public void setSessionFactory(SessionFactory sf) { this.sessionFactory = sf; } public List getAllCountries() { Session session = this.sessionFactory.getCurrentSession(); List countryList = session.createQuery("from Country").list(); return countryList; } public Country getCountry(int id) { Session session = this.sessionFactory.getCurrentSession(); Country country = (Country) session.load(Country.class, new Integer(id)); return country; } public Country addCountry(Country country) { Session session = this.sessionFactory.getCurrentSession(); session.persist(country); return country; } public void updateCountry(Country country) { Session session = this.sessionFactory.getCurrentSession(); session.update(country); } public void deleteCountry(int id) { Session session = this.sessionFactory.getCurrentSession(); Country p = (Country) session.load(Country.class, new Integer(id)); if (null != p) { session.delete(p); } } } |
@Repository is specialised component annotation which is used to create bean at DAO layer. We have use Autowired annotation to inject hibernate SessionFactory into CountryDAO class. We have already configured hibernate SessionFactory object in Spring-Servlet.xml file.
Create Service class
It is service level class. It will call DAO layer class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
package org.arpit.java2blog.service; import java.util.List; import org.arpit.java2blog.dao.CountryDAO; import org.arpit.java2blog.model.Country; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; @Service("countryService") public class CountryService { @Autowired CountryDAO countryDao; @Transactional public List getAllCountries() { return countryDao.getAllCountries(); } @Transactional public Country getCountry(int id) { return countryDao.getCountry(id); } @Transactional public void addCountry(Country country) { countryDao.addCountry(country); } @Transactional public void updateCountry(Country country) { countryDao.updateCountry(country); } @Transactional public void deleteCountry(int id) { countryDao.deleteCountry(id); } } |
7)Â It ‘s time to do maven build.
Right click on project -> Run as -> Maven build
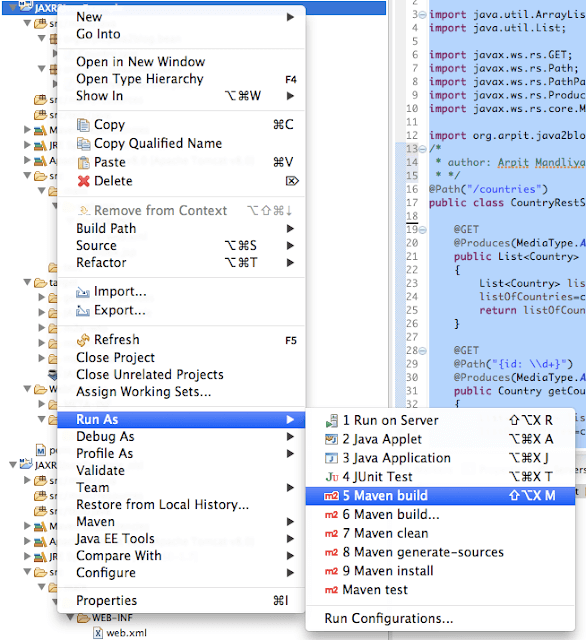
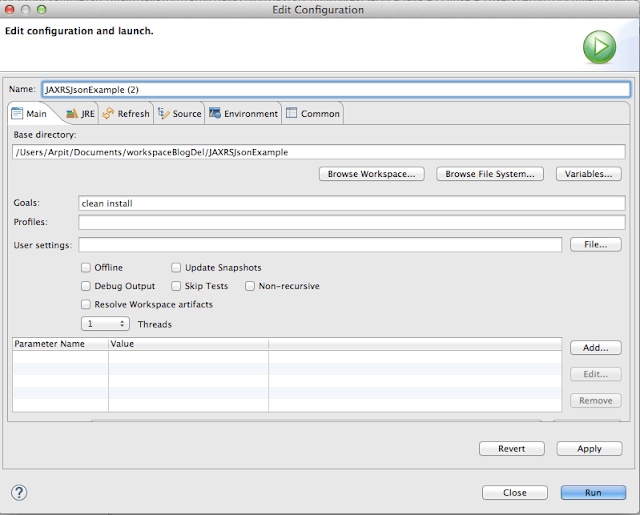
Run the application
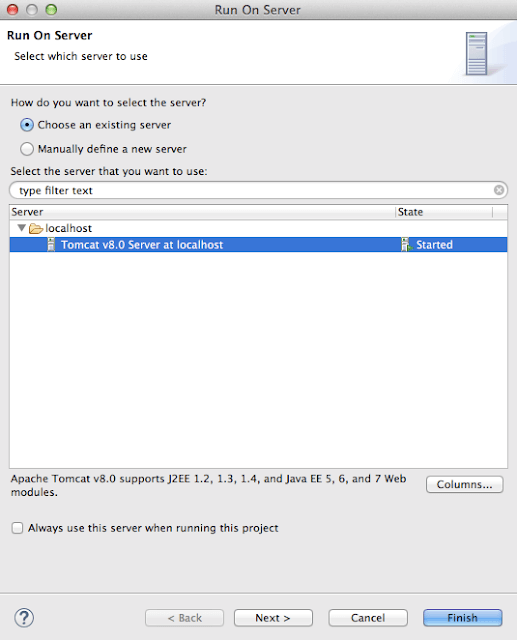
Post method
12)Â Post method is used to create new resource. Here we are adding new Country India to country list, so you can see we have used new country json in post body.
URL:Â “http://localhost:8080/SpringRestHibernateExample/addCountry”.
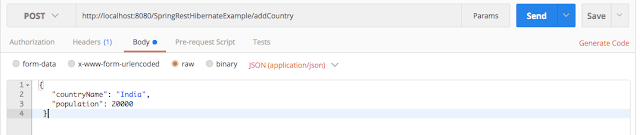
Lets see corresponding entry in Country table in database.

Lets create 3 more countries i.e. china, nepal and USA in similar way.
Put Method
13)Â Put method is used to update resource. Here will update population of nepal using put method.
We will update country json in body of request.
URL :Â “http://localhost:8080/SpringRestHibernateExample/updateCountry”
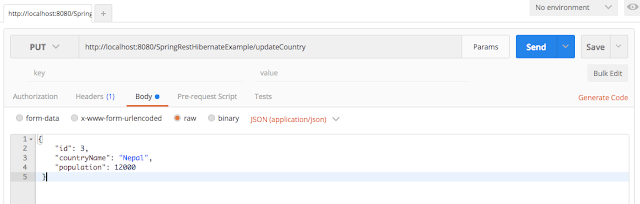
Lets check Nepal’s population in database.
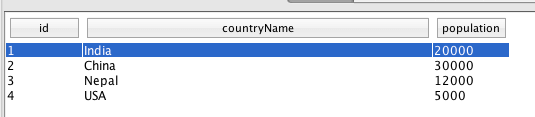
Delete method
14)Â Delete method is used to delete resource.We will pass id of country which needs to be deleted as PathParam. We are going delete id:3 i.e. Nepal to demonstrate delete method.
URL :Â “http://localhost:8080/SpringRestHibernateExample/deleteCountry/3”
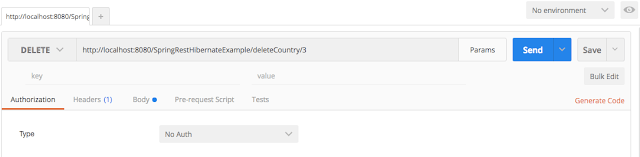
Lets check entry in database now.
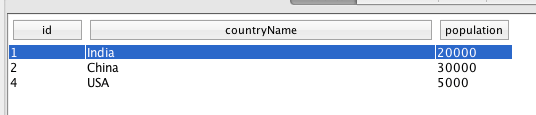
As you can see, we have deleted country with id 3 i.e. Nepal
Project structure:
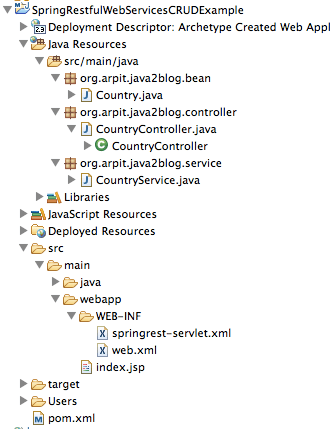
We are done with Spring Restful web services json CRUD example. If you are still facing any issue, please comment.
If you getting 404 error with above steps, you may need to follow below steps:
1)Â If you are getting this warning into your Tomcat startup console log, then it can cause the issue
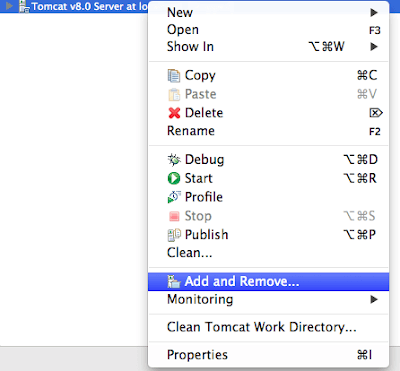
- Go to server view
- Double click on your tomcat server. It will open the server configuration.
- Under server options check ‘Publish module contents to separate XML files’ checkbox.
- Restart your server. This time your page will come without any issues.
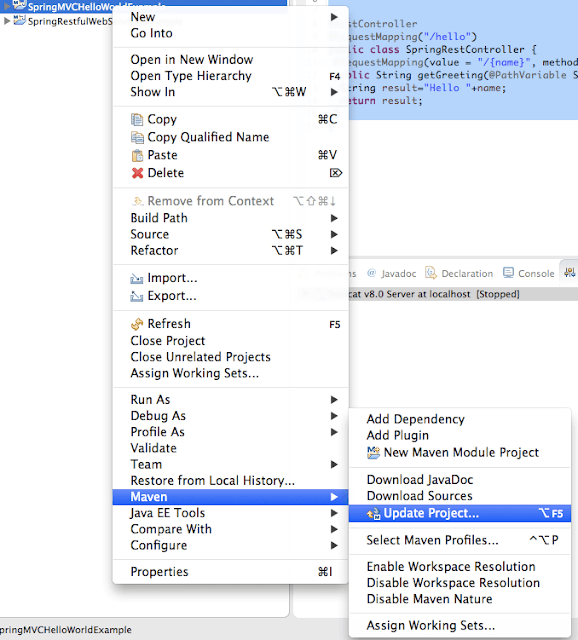
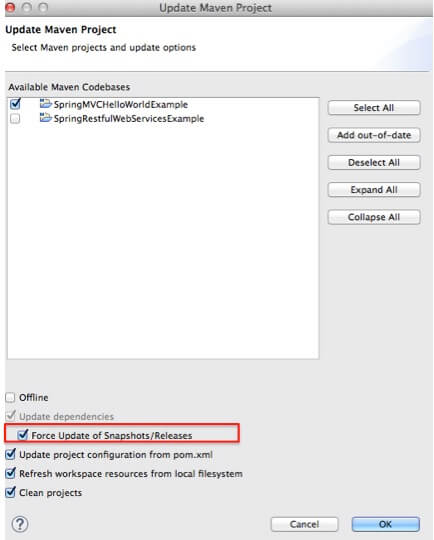
This should solve you issues.
Enti
i have made database in mysql and made hiberate.cfg but how to access the data that are in database using this project
after downloading where should we change
Hi Rohit,
You need to configure mysql and hibernate. I think you have already done it.
You also need to create a table in database as below and run the application. It should work. Let me know if you are still facing any issues.
CREATE TABLE COUNTRY
(
id int PRIMARY KEY NOT NULL AUTO_INCREMENT,
countryName varchar(100) NOT NULL,
population int NOT NULL
)
;
I have implemented the example, I am able to fetch data from database in json format, but other methods like getById, addDetails, Update, delete are not working. Status 500. Please help me to resolve this issue
Thank you