Table of Contents
Web service Tutorial Content:
- Introduction to web services
- Web services interview questions
- SOAP web service introduction
- RESTful web service introduction
- Difference between SOAP and REST web services
- SOAP web service example in java using eclipse
- JAX-WS web service eclipse tutorial
- JAX-WS web service deployment on tomcat
- Create RESTful web service in java(JAX-RS) using jersey
- RESTful web service JAXRS json example using jersey
- RESTful web service JAXRS CRUD example using jersey
- AngularJS RESTful web service JAXRS CRUD example using $http
- RESTful Web Services (JAX-RS) @QueryParam Example
- Spring Rest simple example
- Spring Rest json example
- Spring Rest xml example
- Spring Rest CRUD example
In previous post, we have created a very simple Spring Restful web services  which returns json. In this post, we will see Spring Restful web services which will return xml as example.
Here are steps to create a simple Spring Restful web services which will return xml.
1) Create a dynamic web project using maven in eclipse.
pom.xml will be as follows:
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringRestfulWebServicesWithJSONExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringRestfulWebServicesWithJSONExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> </dependencies> <build> <finalName>SpringRestfulWebServicesWithJSONExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <jdk.version>1.7</jdk.version> </properties> </project> |
Spring application configuration:
3)Â Change web.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>springrest</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springrest</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> |
4)Â create a xml file named springrest-servlet.xml in /WEB-INF/ folder.
Please change context:component-scan if you want to use different package for spring to search for controller.Please refer to spring mvc hello world example for more understanding.
1 2 3 4 5 6 7 8 9 10 11 12 |
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> <mvc:annotation-driven/> <context:component-scan base-package="org.arpit.java2blog.controller" /> </beans> |
Create bean class
5)Â Create a bean name “Country.java” in org.arpit.java2blog.bean.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
package org.arpit.java2blog.bean; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement(name="country") public class Country{ private int id; private String countryName; public Country() { } public Country(int i, String countryName) { super(); this.id = i; this.countryName = countryName; } @XmlElement public int getId() { return id; } public void setId(int id) { this.id = id; } @XmlElement public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } } |
We need to annotate bean class with @XmlRootElement and @XmlElement to support for xml. As you can see we have annotated Country class with
JAXB annotation but if you want to have support for list, we can not edit ArrayList class, so we can create another class called CountryList  and we can annotate with JAXB annotation in that class to support xml output.
CountryList.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
package org.arpit.java2blog.bean; import java.util.List; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement(name = "country-list") public class CountryList { ListlistOfCountries; public CountryList() { super(); } public CountryList(ListlistOfCountries) { this.listOfCountries=listOfCountries; } public ListgetListOfCountries() { return listOfCountries; } @XmlElement(name = "country") public void setListOfCountries(ListlistOfCountries) { this.listOfCountries = listOfCountries; } } |
Create controller
6)Â Create a controller named “CountryController.java”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
package org.arpit.java2blog.controller; import java.util.ArrayList; import java.util.List; import org.arpit.java2blog.bean.Country; import org.arpit.java2blog.bean.CountryList; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; @RestController public class CountryController { @RequestMapping(value = "/countries", method = RequestMethod.GET,headers="Accept=application/xml") public CountryList getCountries() { CountryList countryList=createCountryList(); return countryList; } @RequestMapping(value = "/country/{id}", method = RequestMethod.GET) public Country getCountryById(@PathVariable int id) { ListlistOfCountries = new ArrayList(); CountryList countryList=createCountryList(); listOfCountries=countryList.getListOfCountries(); for (Country country: listOfCountries) { if(country.getId()==id) return country; } return null; } /// Utiliy method to create country list. public CountryList createCountryList() { Country indiaCountry=new Country(1, "India"); Country chinaCountry=new Country(4, "China"); Country nepalCountry=new Country(3, "Nepal"); Country bhutanCountry=new Country(2, "Bhutan"); ListlistOfCountries = new ArrayList(); listOfCountries.add(indiaCountry); listOfCountries.add(chinaCountry); listOfCountries.add(nepalCountry); listOfCountries.add(bhutanCountry); return new CountryList(listOfCountries); } } |
@PathVariable:Â Used to inject values from the URL into a method parameter.This way you inject id in getCountryById method .
We are not providing any view information in springrest-servlet.xml as we do in Spring MVC. If we need to directly get resource from controller, we need to return @ResponseBody as per Spring 3 but with Spring 4, we can use @RestController for that.
In spring 4.0, we can use @RestController which is combination of @Controller + @ResponseBody.
1 2 3 |
@RestController =Â @Controller + @ResponseBody |
6)Â It ‘s time to do maven build.
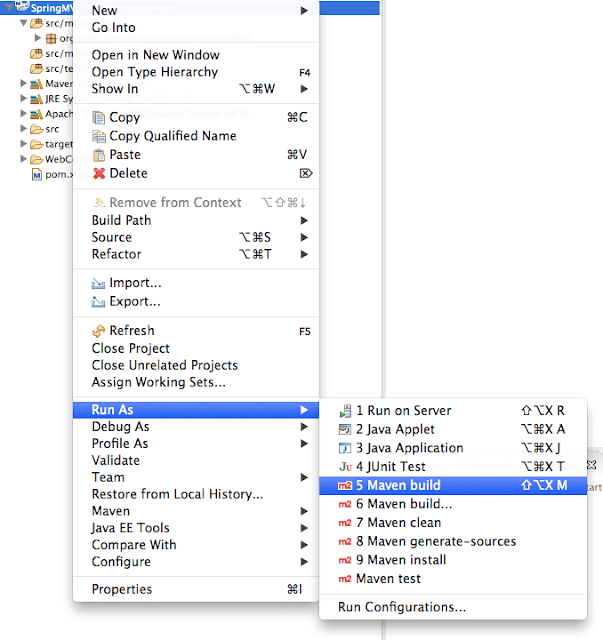
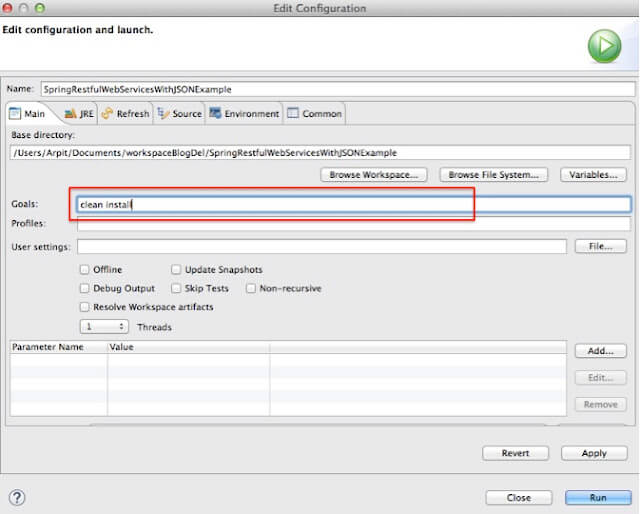
Run the application
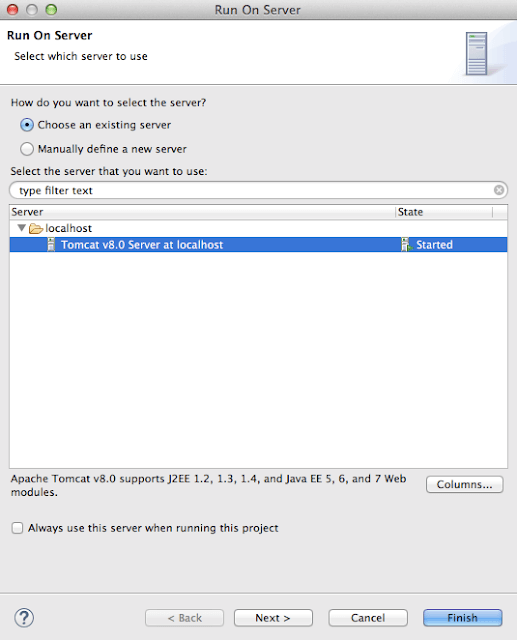
When you run the application, you might get this kind of warning
1 2 3 4 |
Mar 26, 2016 1:45:51 AM org.springframework.web.servlet.PageNotFound noHandlerFound WARNING: No mapping found for HTTP request with URI [/SpringRestfulWebServicesWithXMLExample/] in DispatcherServlet with name 'SpringRestfulWebServicesWithJSONExample' |
Please ignore above warning. When you start application, you have below URL if you have not provided start page:
http://localhost:8080/SpringRestfulWebServicesWithXMLExample/Â
As we have used DispatcherServlet in web.xml, this request goes to spring DispatcherServlet and it did not find corresponding mapping in controller , hence you get that warning.
9)Â Test your REST service under: “http://localhost:8080/SpringRestfulWebServicesWithXMLExample/countries”.
You will get following output:
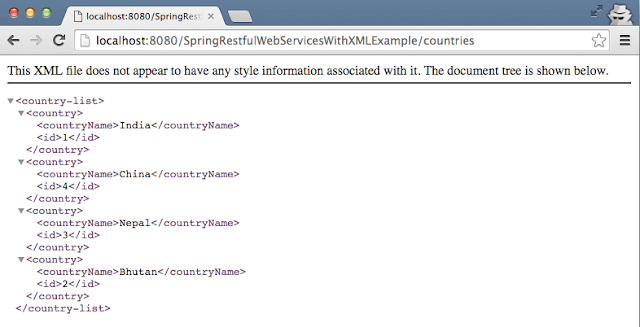
“http://localhost:8080/SpringRestfulWebServicesWithXMLExample/country/2”.
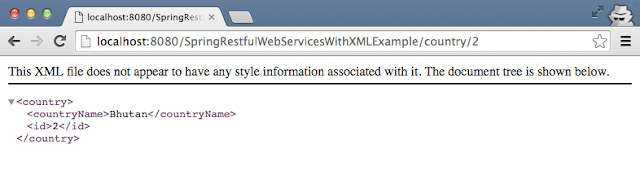
That’s all about Spring Restful web services xml example.