In this post, we will see how to left pad Integer with zeroes in java.
There are multiple ways to left pad Integer with zeroes in java.
Table of Contents
Using String’s format() method
You can use String
‘s format() method to add leading zeroes to Integer in java. This is simplest and elegant solution to left pad Integer with zeroes in java.
Let’s say you want to add 3 leading zeroes to Integer of lenght 2, so you need to provide following format:
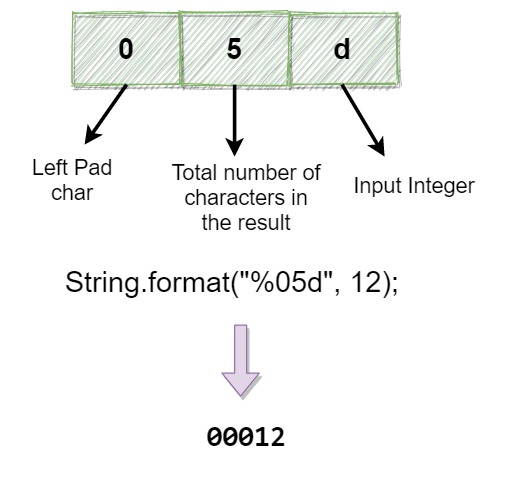
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 |
package org.arpit.java2blog; public class PadzeroestringFormat { public static void main(String[] args) { int i = 12; System.out.printf("%05d",i); } } |
Output:
Using DecimalFormat
You can also use DecimalFormat‘s format() method to left pad Integer with zeroes. You need to pass format with all 0 of total length to do this.
For example:
1 2 3 4 5 6 7 8 9 10 11 |
int i = 12; DecimalFormat df = new DecimalFormat("00000"); String padLeftStr = df.format(i); System.out.println(padLeftStr); // Prints 00012 DecimalFormat df = new DecimalFormat("000"); String padLeftStr = df.format(i); System.out.println(padLeftStr); // Print 0012 |
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
package org.arpit.java2blog.entry; import java.text.DecimalFormat; public class PadZeroDecimalFormat { public static void main(String[] args) { int i = 12; DecimalFormat df = new DecimalFormat("00000"); String padLeftStr = df.format(i); System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
Using NumberFormat
You can use NumberFormat’s format()
method with setMaximumIntegerDigits()
and setMinimumIntegerDigits()
to add leading zeroes to Integer in java.
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
package org.arpit.java2blog.entry; import java.text.NumberFormat; public class PadZeroNumberFormat { public static void main(String[] args) { int i=12; NumberFormat numberFormat = NumberFormat.getInstance(); numberFormat.setMaximumIntegerDigits(5); numberFormat.setMinimumIntegerDigits(5); numberFormat.setGroupingUsed(false); String padLeftStr = numberFormat.format(i); System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
Using apache common lang3
You can use Apache common’s StringUtils
class to pad integer with leading zeroes.
Here is the dependency which you need to add for Apache common lang3 in pom.xml
.
1 2 3 4 5 6 7 |
<dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.9</version> </dependency> |
Here, we are using StringUtils.leftPad()
method. Here are the overloaded version of leftPad.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
/** * @param str - the String to pad out, may be null * @param size - Final length of the string * @param padChar - character or string which you want to use for left pad * * @return left pad string or original string if padding is not required. */ public static String leftPad(final String str, final int size) {...} public static String leftPad(final String str, final int size, String padStr) {...} public static String leftPad(final String str, final int size, final char padChar) { ... } |
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
package org.arpit.java2blog.entry; import org.apache.commons.lang3.StringUtils; public class PadzeroestringUtils { public static void main(String[] args) { int i = 12; String padLeftStr = StringUtils.leftPad(""+i,5,'0'); System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
Using Guava library
You can also use Guava’s Strings
class to left pad String.
Here is the dependency which you need to add for guava in pom.xml
.
1 2 3 4 5 6 7 |
<dependency> <groupId>com.google.guava</groupId> <artifactId>guava</artifactId> <version>29.0-jre</version> </dependency> |
Here, we are using Strings.padStart()
method. The overloaded version of padStart()
are:
1 2 3 4 5 6 7 8 9 10 11 |
/** * @param string - the String to pad out, it may be null * @param minLength - Final length of the string * @param padChar - character or string which you want to use for left pad * * @return left pad string or original string if padding is not required. */ public static String padEnd(String string, int minLength, char padChar){...} |
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
package org.arpit.java2blog; import com.google.common.base.Strings; public class PadZeroGauva { public static void main(String[] args) { int i = 12; String padLeftStr = Strings.padStart("" + i, 5, '0'); System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
Using System.out.printf
If you just want to print left pad Integer with zeroes in java, you can use System.out.printf()
.
Here is an example:
1 2 3 4 5 6 7 8 9 |
package org.arpit.java2blog.entry; public class PadLeftPrintf { public static void main(String[] args) { } } |
Output:
Using StringBuilder
You can write your own solution using for loop and StringBuffer
rather than using any library method.
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
package org.arpit.java2blog.entry; public class PadzeroestringBuilder { public static void main(String[] args) { int i=12; String str = ""+i; StringBuilder sb = new StringBuilder(); int size=5; for (int leftzeroes=size-str.length(); leftzeroes>0; leftzeroes--) { sb.append('0'); } sb.append(str); String padLeftStr = sb.toString(); System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
Using String’s substring() method
You can use for loop and substring()
method to left pad integer with zeroes in java.
First create a String of total length of all 0s and then use substring() to extract required left pad zeroes and concatenate original String to it.
Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
package org.arpit.java2blog.entry; public class PadLeftSubstring { public static void main(String[] args) { int inputNo=12; String str = ""+inputNo; String padZero=""; int size=5; for (int i = 0; i < 5; i++) { padZero += "0"; } String padLeftStr = padZero.substring(str.length()) + str; System.out.println("Formatted Integer with 3 leading zeroes: "+padLeftStr); } } |
Output:
That’s all about how to left pad integer with zeroes in java.