Some basics about Apache POI:
There are two prefixes which you encounter while reading/writing excel in java
HSSF:Â Used for dealing with files excel 2003 or earlier(.xls). Some of classes with HSSF prefix are HSSFWorkbook , HSSFSheet , HSSFRow and HSSFCell.
XSSF:Â Used for dealing with files excel 2007 or later(.xlsx). Some of classes with XSSF prefix are XSSFWorkbook , XSSFSheet , XSSFRow and XSSFCell.
Here are few classes which you need to aware of.
- Workbook :Â This is high level class for representing excel workbook.
- Sheet :Â This is high level class for representing excel sheet.
- Row :Â This is high level class for representing excel row. It has methods which are related to row.
- Cell:Â This is high level class for representing individual excel cell. It has methods which are related to cell for example : getDataType().
Dependency:
1 2 3 4 5 6 7 8 9 |
org.apache.poi poi 3.13 org.apache.poi poi-ooxml 3.13Â |
- poi-3.13.jar
- commons-codec-1.9.jar
- poi-ooxml-3.13.jar
- poi-ooxml-schemas-3.13.jar
- xmlbeans-2.6.0.jar
- stax-api-1.0.1.jar
write excel file using poi:
- Create a blank workbook
- Create a sheet and pass name of the sheet
- Create row
- Create cells, set its value and add cell to above row
- Repeat 3 and 4 until you have data
XSSFWorkbook workbook = new XSSFWorkbook();
XSSFSheet sheet = workbook.createSheet(“Country”);
Row row = sheet.createRow(rownum++);
Cell cell = row.createCell(cellnum++); if(obj instanceof String) cell.setCellValue((String)obj); else if(obj instanceof Double) cell.setCellValue((Double)obj); else if(obj instanceof Integer) cell.setCellValue((Integer)obj);
Java Program:
We are going to write excel file named  “CountriesDetails.xlsx” Create WriteExcelMain.java as below
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
package org.arpit.java2blog; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.util.ArrayList; import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; public class WriteExcelMain { public static void main(String[] args) throws IOException { writeFileUsingPOI(); } public static void writeFileUsingPOI() throws IOException { //create blank workbook XSSFWorkbook workbook = new XSSFWorkbook(); //Create a blank sheet XSSFSheet sheet = workbook.createSheet("Country"); ArrayList<Object[]> data=new ArrayList<Object[]>(); data.add(new String[]{"Country","Capital","Population"}); data.add(new Object[]{"India","Delhi",10000}); data.add(new Object[]{"France","Paris",40000}); data.add(new Object[]{"Germany","Berlin",20000}); data.add(new Object[]{"England","London",30000}); //Iterate over data and write to sheet int rownum = 0; for (Object[] countries : data) { Row row = sheet.createRow(rownum++); int cellnum = 0; for (Object obj : countries) { Cell cell = row.createCell(cellnum++); if(obj instanceof String) cell.setCellValue((String)obj); else if(obj instanceof Double) cell.setCellValue((Double)obj); else if(obj instanceof Integer) cell.setCellValue((Integer)obj); } } try { //Write the workbook in file system FileOutputStream out = new FileOutputStream(new File("CountriesDetails.xlsx")); workbook.write(out); out.close(); System.out.println("CountriesDetails.xlsx has been created successfully"); } catch (Exception e) { e.printStackTrace(); } finally { workbook.close(); } } } |
When you run above program, you will get following output:
1 2 3 |
CountriesDetails.xlsx has been created successfully |
Lets see content of CountriesDetails.xlsx now.
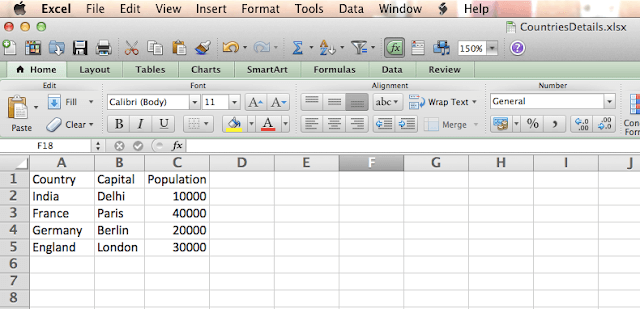
Was this post helpful?
Share this
-
Prev
How to read excel files in java using Apache POI
-
Next
How to set style in excel using Apache POI in java
Good example