Table of Contents
In this post, we will see how to read object from file in java. In last post, we have already seen how to write object to a file and created employee.ser , now in this post, we are going to read same file and retrieve back Employee object.
Java Serialization Tutorial:
- Serialization in javaJava
- Serialization interview questions and answers
- serialversionuid in java serialization
- externalizable in java
- Transient keyword in java
- Difference between Serializable and Externalizable in Java
Steos for reading object from file are:
Reading object from file using ObjectInputStream may be referred as Deserialization.
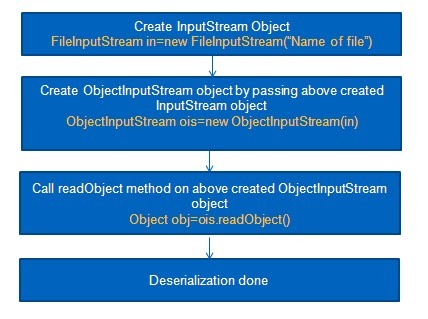
Lets take an example:
Create Employee.java same as last example in src->org.arpit.java2blog
Create DeserializeMain.java in src->org.arpit.java2blog
Create Employee.java same as last example in src->org.arpit.java2blog
1.Employee.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
package org.arpit.java2blog; import java.io.Serializable; public class Employee implements Serializable{Â private static final long serialVersionUID = 1L;Â Â Â Â Â int employeeId; Â Â Â String employeeName; Â Â Â String department; Â Â Â Â Â Â public int getEmployeeId() { Â Â Â Â Â Â return employeeId; Â Â Â } Â Â Â public void setEmployeeId(int employeeId) { Â Â Â Â Â Â this.employeeId = employeeId; Â Â Â } Â Â Â public String getEmployeeName() { Â Â Â Â Â Â return employeeName; Â Â Â } Â Â Â public void setEmployeeName(String employeeName) { Â Â Â Â Â Â this.employeeName = employeeName; Â Â Â } Â Â Â public String getDepartment() { Â Â Â Â Â Â return department; Â Â Â } Â Â Â public void setDepartment(String department) { Â Â Â Â Â Â this.department = department; Â Â Â } } |
1 2 3 |
2.DeserializeMain.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
package org.arpit.java2blog; import java.io.IOException; import java.io.ObjectInputStream; public class DeserializeMain { /** * @author Arpit Mandliya */ public static void main(String[] args) { Employee emp = null; try { FileInputStream fileIn =new FileInputStream("employee.ser"); ObjectInputStream in = new ObjectInputStream(fileIn); emp = (Employee) in.readObject(); in.close(); fileIn.close(); }catch(IOException i) { i.printStackTrace(); return; }catch(ClassNotFoundException c) { System.out.println("Employee class not found"); c.printStackTrace(); return; } System.out.println("Deserialized Employee..."); System.out.println("Emp id: " + emp.getEmployeeId()); System.out.println("Name: " + emp.getEmployeeName()); System.out.println("Department: " + emp.getDepartment()); } } |
3.Run it:
When you run above DeserializeMain.java and you will get following output:
1 2 3 4 5 6 |
Deserialized Employee... Emp id: 101 Name: Arpit Department: CS |
These are some simple steps to read object from file. If you want to go through some complex scenario you can go through Serialization in java
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.