Table of Contents
- Introduction to web services
- Web services interview questions
- SOAP web service introduction
- RESTful web service introduction
- Difference between SOAP and REST web services
- SOAP web service example in java using eclipse
- JAX-WS web service eclipse tutorial
- JAX-WS web service deployment on tomcat
- Create RESTful web service in java(JAX-RS) using jerse
- yRESTful web service JAXRS json example using jersey
- RESTful web service
- JAXRS CRUD example using jersey
- AngularJS RESTful web service
- JAXRS CRUD example using $http
- RESTful Web Services (JAX-RS) @QueryParam Example
- Spring Rest simple example
- Spring Rest json example
- Spring Rest xml example
- Spring Rest CRUD example
In previous post, we have created a very simple Spring Restful web services  which returns plain text. In this post, we will see Spring Restful web services which will return json as example. If you want complete integration with hibernate and mysql, you can go through Spring Restful hibernate mysql example.
Here are steps to create a simple Spring Restful web services which will return json.
1) Create a dynamic web project using maven in eclipse.
1 2 3 4 5 6 7 |
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> |
Now change pom.xml as follows:
pom.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.arpit.java2blog</groupId> <artifactId>SpringRestfulWebServicesWithJSONExample</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>SpringRestfulWebServicesWithJSONExample Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-webmvc</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.4.1</version> </dependency> </dependencies> <build> <finalName>SpringRestfulWebServicesWithJSONExample</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${jdk.version}</source> <target>${jdk.version}</target> </configuration> </plugin> </plugins> </build> <properties> <spring.version>4.2.1.RELEASE</spring.version> <jdk.version>1.7</jdk.version> </properties> </project> |
Spring application configuration:
3)Â Change web.xml as below:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<!DOCTYPE web-app PUBLIC "-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN" "http://java.sun.com/dtd/web-app_2_3.dtd" > <web-app> <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>springrest</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springrest</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> |
4)Â create a xml file named springrest-servlet.xml in /WEB-INF/ folder.
Please change context:component-scan if you want to use different package for spring to search for controller.Please refer to spring mvc hello world example for more understanding.
1 2 3 4 5 6 7 8 9 10 11 12 |
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd"> <mvc:annotation-driven/> <context:component-scan base-package="org.arpit.java2blog.controller" /> </beans> |
Create bean class
5) Create a bean name “Country.java” in org.arpit.java2blog.bean.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
package org.arpit.java2blog.bean; public class Country{ int id; String countryName; public Country(int i, String countryName) { super(); this.id = i; this.countryName = countryName; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } } |
Create controller
6)Â Create a controller named “CountryController.java”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
package org.arpit.java2blog.controller; import java.util.ArrayList; import java.util.List; import org.arpit.java2blog.bean.Country; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RestController; @RestController public class CountryController { @RequestMapping(value = "/countries", method = RequestMethod.GET,headers="Accept=application/json") public List getCountries() { List listOfCountries = new ArrayList(); listOfCountries=createCountryList(); return listOfCountries; } @RequestMapping(value = "/country/{id}", method = RequestMethod.GET,headers="Accept=application/json") public Country getCountryById(@PathVariable int id) { List listOfCountries = new ArrayList(); listOfCountries=createCountryList(); for (Country country: listOfCountries) { if(country.getId()==id) return country; } return null; } // Utiliy method to create country list. public List createCountryList() { Country indiaCountry=new Country(1, "India"); Country chinaCountry=new Country(4, "China"); Country nepalCountry=new Country(3, "Nepal"); Country bhutanCountry=new Country(2, "Bhutan"); List listOfCountries = new ArrayList(); listOfCountries.add(indiaCountry); listOfCountries.add(chinaCountry); listOfCountries.add(nepalCountry); listOfCountries.add(bhutanCountry); return listOfCountries; } } |
@PathVariable:Â Used to inject values from the URL into a method parameter.This way you inject id in getCountryById method.
We are not providing any view information in springrest-servlet.xml as we do in Spring MVC. If we need to directly get resource from controller, we need to return @ResponseBody as per Spring 3 but with Spring 4, we can use @RestController for that.
In spring 4.0, we can use @RestController which is combination of @Controller + @ResponseBody.
1 2 3 |
@RestController =Â @Controller + @ResponseBody |
6)Â It ‘s time to do maven build.
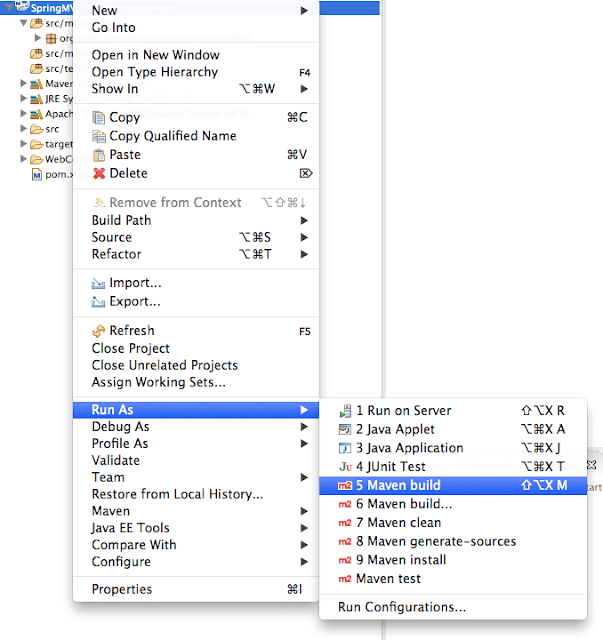
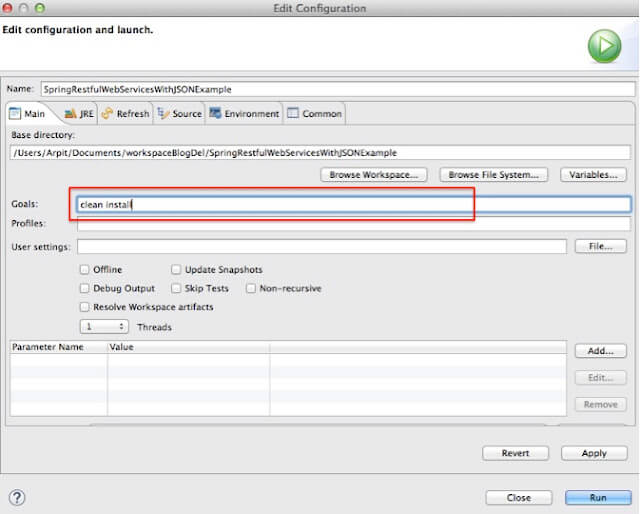
Run the application
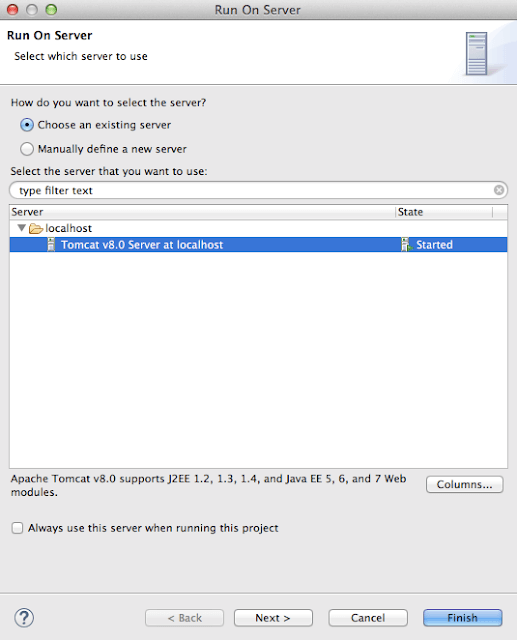
When you run the application, you might get this kind of warning
1 2 3 4 |
Mar 26, 2016 1:45:51 AM org.springframework.web.servlet.PageNotFound noHandlerFound WARNING: No mapping found for HTTP request with URI [/SpringRestfulWebServicesWithJSONExample/] in DispatcherServlet with name 'SpringRestfulWebServicesWithJSONExample' |
Please ignore above warning. When you start application, you have below URL if you have not provided start page:
http://localhost:8080/SpringRestfulWebServicesWithJSONExample/Â
As we have used DispatcherServlet in web.xml, this request goes to spring DispatcherServlet and it did not find corresponding mapping in controller , hence you get that warning.
9)Â Test your REST service under: “http://localhost:8080/SpringRestfulWebServicesWithJSONExample/countries”.
You will get following output:

“http://localhost:8080/SpringRestfulWebServicesWithJSONExample/country/2”.
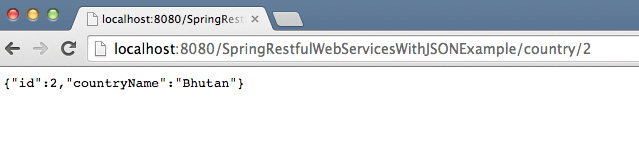
That’s all about Spring Restful web services json example.
Best and simple way of implementing Restful webservice. Very useful
Thanks
Hello, I have one problem with this example…
lis 11, 2015 3:09:30 PM org.springframework.web.servlet.PageNotFound noHandlerFound
WARNING: No mapping found for HTTP request with URI [/SpringRestfulWebServicesWithJSONExample/countries] in DispatcherServlet with name 'springrest'
I think you might have copied over the package value of base-package=”org.arpit.java2blog.controller” /> in springrest-servlet.xml. You need to put in your own package name instead… I fell victim to this error as well and it took me a few too many minutes to find the issue…
you have said , to put the code in class path,, where is this class path
Are you using maven for running above project? If yes, then maven will automatically fetch required library and will put it in the build path.
Let me know if you need more help on this. Thanks for asking
hi i am getting this error now:-
Feb 02, 2016 2:11:24 PM org.springframework.web.servlet.PageNotFound noHandlerFound
WARNING: No mapping found for HTTP request with URI [/mavenWebApp/] in DispatcherServlet with name 'springrest'
my app name is mavenWebAppp
please check springrest-servlet.xml in /WEB-INF/ folder once
you need to change context:component-scan if you want to use different package for spring to search for controller.
let me know if you need more help on it.
works fine, thank you!
can you tell how to produce xml format using a similar method to this json way?
Works fine, thank you so much !!
Works Fine, But how to send json response back (http POST) not discussed.
Please go through below link for post operation : https://www.java2blog.com/2016/04/spring-restful-w…
If not working fine, “No mapping found for HTTP request with URI ” remember, RUN THE MAVEN UPDATE BITCH!
I was with this problem and thus resolved, rs. THANKS alot
Dear Author,
Impressed your article has worked as one shot operation.
Just downloaded, Built with Maven, updated Maven, Run on Tomcat Server.
Perfect example for Rest WebService.
Thank you so much.
Regards,
Ameen Mohammad
ONE problem I encountered was Junit Dependency was not getting resolve so application was not working. may be the version provided in the example is deprecated now. I removed that dependency and it worked.
one more thing I did wrong was not changes the import package name for bean class in controller class. I used as is given here ” org.arpit.java2blog.bean.Country” which was wrong according to my folder structure. I know this was silly mistake but even the eclipse was not showing any error.
BTW thanks arpit . good article.
yah good
Dear Author,
I impressed your code, but i am getting small error.
org.springframework.beans.factory.BeanDefinitionStoreException: IOException parsing XML document from ServletContext resource.
any help highly appreciated
Regards
Great Thanks
You don’t know how much you do beneficence on me to provide this solution, you know I have been searching this answer for 5 days. thanks a lot