Table of Contents
In this post, we will see how to detect if enter key is pressed or not in jQuery.
If you want to catch keypress event in whole web page, you can simply use:
To capture enter keypress, we will bind dom element with keypress event and if ascii value is 13, then enter key is pressed.
So basically code will look something like this.
1 2 3 4 5 6 7 8 |
$('#textBox').keypress(function(event){ var keycode = (event.keyCode ? event.keyCode : event.which); if(keycode == '13'){ alert('You pressed a "enter" key in textbox'); } }); |
1 2 3 4 5 6 7 8 |
$('#document').keypress(function(event){ var keycode = (event.keyCode ? event.keyCode : event.which); if(keycode == '13'){ alert('You pressed a "enter" key in textbox'); } }); |
JQuery keypress enter example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<script src="http://code.jquery.com/jquery-2.2.3.min.js"></script> <h1>Detect if Enter key is pressed</h1> <label>TextBox : </label> <input id="textbox" type="text" size="40"> <script type="text/javascript"> $('#textbox').keypress(function(event){ var keycode = (event.keyCode ? event.keyCode : event.which); if(keycode == '13'){ alert('You pressed a "enter" key in textbox'); } }); </script> |
Output:
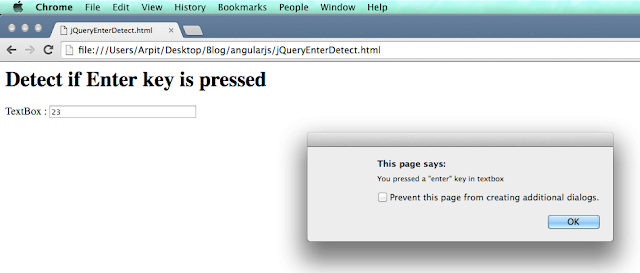
Live demo: jQuery keypress enter example
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.