Table of Contents
In this post , we will see how to set style in excel using apache poi.
You can put cell style with below code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// create font XSSFFont font= workbook.createFont(); font.setFontHeightInPoints((short)10); font.setFontName("Arial"); font.setColor(IndexedColors.WHITE.getIndex()); font.setBold(true); font.setItalic(false); // Create cell style CellStyle style=workbook.createCellStyle();; style.setFillPattern(CellStyle.SOLID_FOREGROUND); style.setAlignment(CellStyle.ALIGN_CENTER); // Setting font to style style.setFont(font); // Setting cell style cell.setCellStyle(style); |
Java Program :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 |
package org.arpit.java2blog; import java.io.File; import java.io.FileOutputStream; import java.io.IOException; import java.util.ArrayList; import org.apache.poi.ss.usermodel.Cell; import org.apache.poi.ss.usermodel.CellStyle; import org.apache.poi.ss.usermodel.IndexedColors; import org.apache.poi.ss.usermodel.Row; import org.apache.poi.xssf.usermodel.XSSFFont; import org.apache.poi.xssf.usermodel.XSSFSheet; import org.apache.poi.xssf.usermodel.XSSFWorkbook; public class WriteExcelMain { public static void main(String[] args) throws IOException { writeFileUsingPOI(); } public static void writeFileUsingPOI() throws IOException { //create blank workbook XSSFWorkbook workbook = new XSSFWorkbook(); //Create a blank sheet XSSFSheet sheet = workbook.createSheet("Country"); ArrayList<Object[]> data=new ArrayList<Object[]>(); data.add(new String[]{"Country","Capital","Population"}); data.add(new Object[]{"India","Delhi",10000}); data.add(new Object[]{"France","Paris",40000}); data.add(new Object[]{"Germany","Berlin",20000}); data.add(new Object[]{"England","London",30000}); //Iterate over data and write to sheet int rownum = 0; for (Object[] countries : data) { Row row = sheet.createRow(rownum++); int cellnum = 0; for (Object obj : countries) { Cell cell = row.createCell(cellnum++); if(obj instanceof String) cell.setCellValue((String)obj); else if(obj instanceof Double) cell.setCellValue((Double)obj); else if(obj instanceof Integer) cell.setCellValue((Integer)obj); // Setting style only for header if(rownum==1) { CellStyle style=null; // Creating a font XSSFFont font= workbook.createFont(); font.setFontHeightInPoints((short)10); font.setFontName("Arial"); font.setColor(IndexedColors.WHITE.getIndex()); font.setBold(true); font.setItalic(false); style=workbook.createCellStyle();; style.setFillPattern(CellStyle.SOLID_FOREGROUND); style.setAlignment(CellStyle.ALIGN_CENTER); // Setting font to style style.setFont(font); // Setting cell style cell.setCellStyle(style); } } } Row rowGap = sheet.createRow(rownum++); Row row = sheet.createRow(rownum++); Cell cellTotal = row.createCell(0); cellTotal.setCellValue("Total Population"); // Setting cell formula and cell type Cell cell = row.createCell(2); cell.setCellFormula("SUM(C2:C5)"); cell.setCellType(Cell.CELL_TYPE_FORMULA); try { //Write the workbook to the file system FileOutputStream out = new FileOutputStream(new File("CountriesDetails.xlsx")); workbook.write(out); out.close(); System.out.println("CountriesDetails.xlsx has been created successfully"); } catch (Exception e) { e.printStackTrace(); } finally { workbook.close(); } } } |
1 2 3 |
CountriesDetails.xlsx has been created successfully |
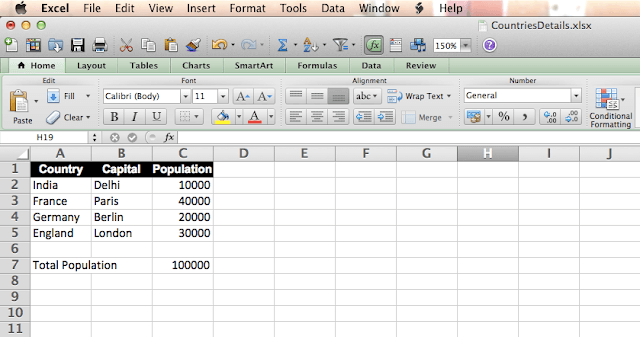
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.