If you want to practice data structure and algorithm programs, you can go through data structure and algorithm interview questions.
In this post, we will see how to find transpose of matrix in java.
Transpose of matrix?
A matrix which is created by converting all the rows of a given matrix into columns and vice-versa.
Below image shows example of matrix transpose.
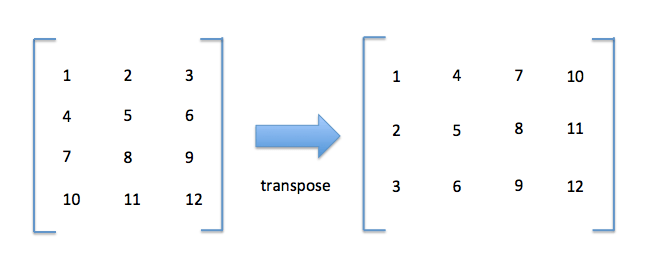
So as you can see we have converted rows to columns and vice versa.
Java program to find transpose of matrix:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
package org.arpit.java2blog; /** @author Arpit Mandliya */ public class TransposeMatrixMain { public static void main(String args[]) { // initialize the variable int[][] a = { { 1, 2, 3 }, { 4, 5, 6 }, { 7, 8, 9 },{10, 11, 12} }; // This will store resultant transpose matrix int[][] transposeMtrx=new int[a[0].length][a.length]; // print the original matrix System.out.println("Original matrix:"); for (int i = 0; i < 4; i++) { for (int j = 0; j < 3; j++) { System.out.print(" "+a[i][j]); } System.out.println(); } // Performing transpose operation for (int i = 0; i < 4; i++) { for (int j = 0; j < 3; j++) { transposeMtrx[j][i] = a[i][j]; } } System.out.println("======================"); System.out.println("Transpose matrix:"); // print transpose matrix for (int i = 0; i < 3; i++) { for (int j = 0; j < 4; j++) { System.out.print(" "+transposeMtrx[i][j]); } System.out.println(); } } } |
1 2 3 4 5 6 7 8 9 10 11 12 |
Original matrix: 1 2 3 4 5 6 7 8 9 10 11 12 ====================== Transpose matrix: 1 4 7 10 2 5 8 11 3 6 9 12 |
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.