💡 Outline
You can copy DataFrame in Pandas usingcopy()
method ofDataFrame
. By default, it will create deep copy of DataFrame.
123456789101112131415161718 import pandas as pddf = pd.DataFrame([['Jay','M',21],['Jennifer','F',17],['Preity','F',19],['Neil','M',17]],By only using the assignment operator <code>=</code>, we are not creating a copy of a DataFrame. It will only create a view of the original and act as another name to access the DataFrame like a pointer.See the following example.<pre code = "python">import pandas as pddf = pd.DataFrame([['Jay','M',21],['Jennifer','F',17],['Preity','F',19],['Neil','M',17]],columns = ['Name','Gender','Age'])df1 = dfdf1.iloc[0,0] = 'Mark'print(df)Output:
1234567 Name Gender Age0 Mark M 211 Jennifer F 172 Preity F 193 Neil M 17As visible in the output, the changes we made to the
df1
DataFrame are reflected in the originaldf
DataFrame. To create copies of a DataFrame, we can use thecopy()
function provided by thepandas
module.We have two different copies available in Python. A shallow copy and a deep copy.
A
shallow copy
creates a reference to the original object. Any changes made to this copy are affected in the original object as well.However, a
deep copy
recursively adds all the data of the original object to a new object. This way any changes made in the deep copy are not reflected in the original copy.## Using copy() method to deep copy
DataFrame
in Pandas
By default, thecopy
function creates a deep copy. It has adeep
parameter, which is set to True by default.For example,
12345678910 import pandas as pddf = pd.DataFrame([['Jay','M',21],['Jennifer','F',17],['Preity','F',19],['Neil','M',17]],columns = ['Name','Gender','Age'])df1 = df.copy()df1.iloc[0,0] = 'Mark'print(df)print(df1)Output:
123456789101112 Name Gender Age0 Jay M 211 Jennifer F 172 Preity F 193 Neil M 17Name Gender Age0 Mark M 211 Jennifer F 172 Preity F 193 Neil M 17In the above example, a deep copy of the original
DataFrame
was created. Changes made to this copy are not reflected in the original.## Using copy() method to shallow copy DataFrame in Pandas
If we set thedeep
parameter as false, a shallow copy is created.See the following code.
12345678910 import pandas as pddf = pd.DataFrame([['Jay','M',21],['Jennifer','F',17],['Preity','F',19],['Neil','M',17]],columns = ['Name','Gender','Age'])df1 = df.copy(deep = False)df1.iloc[0,0] = 'Mark'print(df)print(df1)Output:
123456789101112 Name Gender Age0 Mark M 211 Jennifer F 172 Preity F 193 Neil M 17Name Gender Age0 Mark M 211 Jennifer F 172 Preity F 193 Neil M 17We can also use the earlier discussed assignment operator
=
to create shallow copies of DataFrames.That’s all about how to copy DataFrame in Pandas.
Was this post helpful?
Let us know if this post was helpful. Feedbacks are monitored on daily basis. Please do provide feedback as that\'s the only way to improve.
Copy DataFrame in Pandas
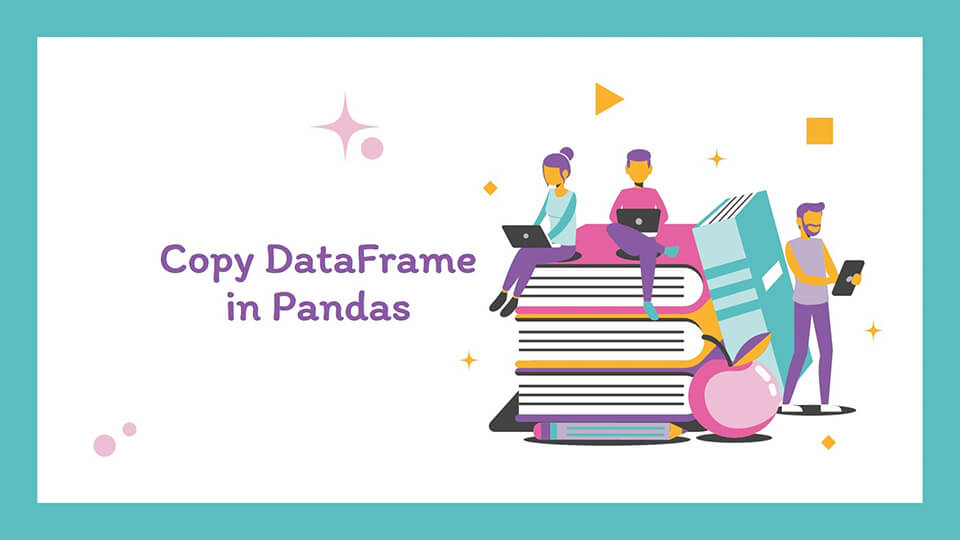