Table of Contents
If you want to practice data structure and algorithm programs, you can go through data structure and algorithm interview questions.
This is one of popular interview question. In this post, we will see how to reverse linked list in pairs.
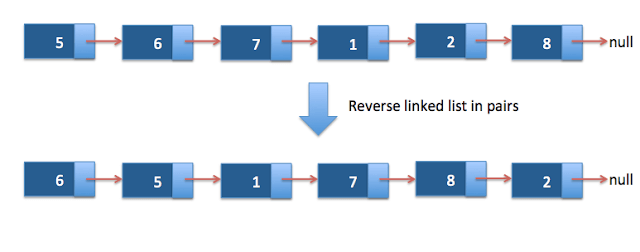
Java Linked List Interview Programs:
- How to reverse a linked list in java
- How to reverse a linked list in pairs in java
- How to find middle element of linked list in java
- How to detect a loop in linked list in java
- Find start node of loop in linkedlist
- How to find nth element from end of linked list
- How to check if linked list is palindrome in java
- Add two numbers represented by linked list in java
- Iterative
- Recursive
Iterative:
Logic for this would be:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
public static Node reverseLinkedListInPairItr(Node head) { Node current=head; Node temp=null; Node newHead =null; while (current != null && current.next != null) { if (temp != null) { // This is important step temp.next.next = current.next; } temp=current.next; current.next=temp.next; temp.next=current; if (newHead == null) newHead = temp; current=current.next; } return newHead; } |
Explanation:
Lets understand with the help of simple example:
Lets say linkedlist is 5-> 6 -> 7 -> 1
If you look closely, below steps are just reversing link to 6->5->7->1 in first iteration (Swapping node 6 and 5) and then advance to next pair (Node 7 and 1)
1 2 3 4 5 6 7 8 9 |
// Node 6 is temp node temp=current.next; // putting link between 5->7 current.next=temp.next; // putting link between 6->5 temp.next=current; // 6 becomes head node here |
You must be wondering why we have put below code:
1 2 3 4 5 6 |
if (temp != null) { // This is important step temp.next.next = current.next; } |
before swapping two nodes , we need to link nodes properly. When we are swapping 7 and 1 , link between 5->7 will be broken and we need to connect node 5 to node 1.
As per above code , temp will be null in first iteration(swapping node 6 and 5) but when current node is 7, temp will be 6, here we are linking nodes as below
6->5->1->7
Java program for this will be :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 |
package org.arpit.java2blog; public class ReverseLinkedListInPair{ private Node head; private static class Node { private int value; private Node next; Node(int value) { this.value = value; } } public void addToTheLast(Node node) { if (head == null) { head = node; } else { Node temp = head; while (temp.next != null) temp = temp.next; temp.next = node; } } public void printList(Node head) { Node temp = head; while (temp != null) { System.out.format("%d ", temp.value); temp = temp.next; } System.out.println(); } // Reverse linked list in pair public static Node reverseLinkedListInPairs(Node head) { Node current=head; Node temp=null; Node newHead =null; while (current != null && current.next != null) { if (temp != null) { // This is important step temp.next.next = current.next; } temp=current.next; current.next=temp.next; temp.next=current; if (newHead == null) newHead = temp; current=current.next; } return newHead; } public static void main(String[] args) { ReverseLinkedListInPair list = new ReverseLinkedListInPair(); // Creating a linked list Node head=new Node(5); list.addToTheLast(head); list.addToTheLast(new Node(6)); list.addToTheLast(new Node(7)); list.addToTheLast(new Node(1)); list.addToTheLast(new Node(2)); list.addToTheLast(new Node(8)); list.printList(head); //Reversing LinkedList in pairs Node result=reverseLinkedListInPairs(head); System.out.println("After reversing in pair"); list.printList(result); } } |
Run above program, you will get following output:
1 2 3 4 5 |
5 6 7 1 2 8 After reversing 6 5 1 7 8 2 |
Recursive:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
public static Node reverseLinkedListInPairs(Node head) { if (head == null || head.next == null) { return head; } // Lets take example of 5->6->7 // Here head node is 5 // Storing 6 in temp node, it will become our new head Node temp=head.next; // Putting link between 5->7 head.next=temp.next; // putting link between 6->5 temp.next=head; // recursively calling the function for node 7 head.next=reverseLinkedListInPairRec(head.next); // returning new head return temp; } |
Output of this program will be same as above program.
Please go through java interview programs for more such programs.