Table of Contents
In this tutorial, we will see about Dropwizard validation example.
As discussed in the previous dropwizard tutorial, hibernate validator is packaged with Dropwizard, so whatever validations you can put with hibernate validator, you can do same with Dropwizard as well.
Resource endpoint validation
You can validate almost anything at resource end point.
Let’s take a quick example.
1 2 3 4 5 6 7 |
@Get public String getCountryByName(@QueryParam("name") @NotEmpty String countryName) { // endpoint implementation } |
Constraining entities
You can put various validations at entity level. I will extend same example which I have provided in dropwizard tutorial.
Let’s annotate book class to apply the following constraints.
- Title must not be null or “Dummy”
- Number of pages in book should be greater than 10 and less than 1000.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package org.arpit.java2blog.model; import javax.validation.constraints.Max; import javax.validation.constraints.Min; import org.hibernate.validator.constraints.NotEmpty; import com.fasterxml.jackson.annotation.JsonIgnore; import io.dropwizard.validation.ValidationMethod; public class Book{ int id; @NotEmpty String title; @Min(10) @Max(1000) long pages; public Book() { } public Book(int id, String title, long pages) { super(); this.id = id; this.title = title; this.pages = pages; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public long getPages() { return pages; } public void setPages(long pages) { this.pages = pages; } @ValidationMethod(message="title must not be dummy") @JsonIgnore public boolean isNotDummy() { return !"Dummy".equalsIgnoreCase(title); } } |
Let’s put @Valid in post and put request in BookController class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
package org.arpit.java2blog.controller; import java.util.List; import javax.validation.Valid; import javax.ws.rs.Consumes; import javax.ws.rs.DELETE; import javax.ws.rs.GET; import javax.ws.rs.POST; import javax.ws.rs.PUT; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; import org.arpit.java2blog.model.Book; import org.arpit.java2blog.service.BookService; @Path("bookService") @Produces(MediaType.APPLICATION_JSON) public class BookController { BookService bookService = new BookService(); @Path("/books") @GET public List<Book> getBooks() { List<Book> listOfBooks = bookService.getAllBooks(); return listOfBooks; } @Path("/book/{id}") @GET public Book getBookById(@PathParam(value = "id") int id) { return bookService.getBook(id); } @Consumes(MediaType.APPLICATION_JSON) @Path("/books") @POST public Book addBook(@Valid Book Book) { return bookService.addBook(Book); } @Path("/books") @PUT @Consumes(MediaType.APPLICATION_JSON) public Book updateBook(@Valid Book Book) { return bookService.updateBook(Book); } @Produces(MediaType.APPLICATION_JSON) @Path("/book/{id}") @DELETE public void deleteBook(@PathParam(value = "id")int id) { bookService.deleteBook(id); } } |
Let’s start DropwizardApplication.java again and test out application using postman.
Post method is used to create new resource. Here we are adding new Book “Effective Javascript” to book list, so you can see we have used new book json in post body.
URL:Â “localhost:8080/bookService/books”.
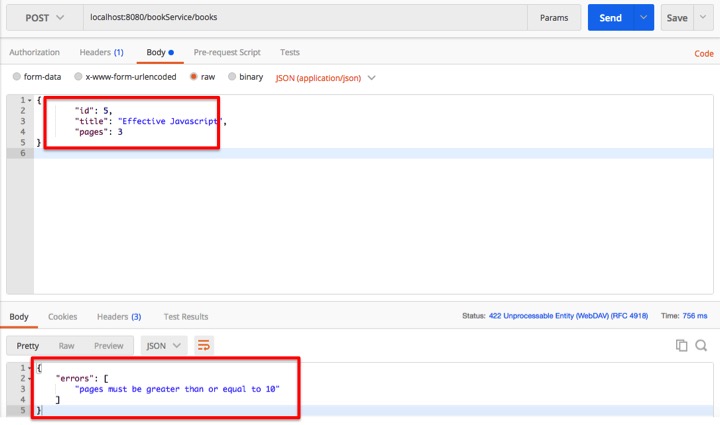
As you can see, we have put number of pages as 3 which is lesser than 10, so we are getting error here.
Another post request:
URL:Â “localhost:8080/bookService/books”.
As we have provided title as “Dummy” in our post request body,isNotDummy will return false and our entity will not be a valid one.
Source code
That’s all about Dropwizard validation example.