Table of Contents
1 2 3 4 5 6 7 8 |
Public enum Severity (Level_1, Level_2, Level_3 ) |
1 2 3 |
Serverity  level= Severity. Level_1; |
Lets understand it with the help of example:
Create enum as SeverityEnum.java
1 2 3 4 5 6 7 8 9 10 |
package org.arpit.java2blog.corejava; public enum SeverityEnum { LEVEL_1, LEVEL_2, LEVEL_3; } |
1 2 3 4 5 6 7 8 9 10 |
package org.arpit.java2blog.corejava; public class SeverityClass { public static final String LEVEL_1="LEVEL_1"; public static final String LEVEL_2="LEVEL_2"; public static final String LEVEL_3="LEVEL_3"; } |
Create issue.java. It will use above enum and class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
package org.arpit.java2blog.corejava; public class Issue { String issueName; public Issue(String issueName) { super(); this.issueName = issueName; } public String getIssueName() { return issueName; } public void setIssueName(String issueName) { this.issueName = issueName; } public void resolveIssueEnum(SeverityEnum level) { System.out.println("Resolving "+issueName+ " of "+level.name()); } public void resolveIssueClass(String level) { System.out.println("Resolving "+issueName+ " of "+level); } } |
Create EnumMain.java .
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
package org.arpit.java2blog.corejava; public class EnumMain { public static void main(String args[]) { Issue a=new Issue("Server is down"); Issue b=new Issue("UI is not formatted properly"); System.out.println("Resolving issues:"); System.out.println("*****************"); a.resolveIssueClass(SeverityClass.LEVEL_2); // You are able to pass "LEVEL_5" also here, but LEVEL_5 is not defined as Severity at all // So this is considered to be invalid value, but you still can pass it if you use class b.resolveIssueClass("LEVEL_5"); // b.resolveIssueEnum("LEVEL_5"); // Compilation error // You can pass only fixed values with this which is our requirement here // So enum ensures type safety a.resolveIssueEnum(SeverityEnum.LEVEL_2); } } |
When you run above program, you will get following output:
1 2 3 4 5 6 7 |
Resolving issues: ***************** Resolving Server is down of LEVEL_2 Resolving UI is not formatted properly of LEVEL_5 Resolving Server is down of LEVEL_2 |
Lets understand more about Enum.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
package org.arpit.java2blog.corejava; public class Issue { String issueName; public Issue(String issueName) { super(); this.issueName = issueName; } public String getIssueName() { return issueName; } public void setIssueName(String issueName) { this.issueName = issueName; } public static void main(String[] args) { Severity level=Severity.LEVEL_2; System.out.println(level.name()); } } |
We can see structure of enum using a debug point.
put debug point at line 21 and right click on project->debug as->java application. Program will stop execution at line 21 then right click on level then select watch.You will be able to see structure as below.
name() : it returns you enum constant. For above enum level, its value is LEVEL_2.
ordinal() : It returns you position in enum declaration. This method is not generally used by programmers, it is used mostly in enum based data structures such as EnumSet.Constructor:
protected Enum(String name, int ordinal)
This is only constructor present by default in enum. This can not be called by program. This is called internally when you declare the enum.I am summarising important points on enum based on their nature.
Constructor:
- Enum can have constructors and can be overloaded.
- Enum constructor can never be invoked directly, it will be implicitly called when enum is initialized.
- You also can provide value to enum constants but you need to create member variable and constructor for that
Methods:
- You can declare methods in enum.
- You can also declare abstract methods in enum and while declaring constants, Â you need to override it.
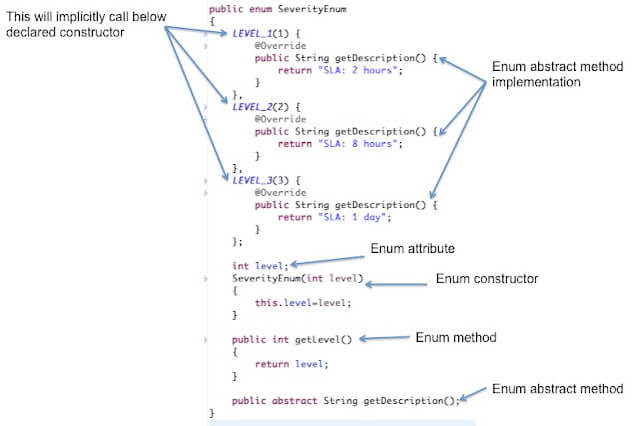
Declaration :
- You can not create instance of enum with new operation as it does not support public constructor and it make sense too as you want fixed values in Enum. If new operator would have been allowed, then you might able to add more values.
1 2 3 4 |
// compilation error Serverity level=new Severity(); |
- Enum constants are implicitly static and final. Â You can not change its value after creation.
- You can not extend any other class to enums as enum implicitly extends Enum class and java does not allow multiple inheritance.
- Enum can be defined inside or outside of a class but can not be defined in method.
- Enum declared outside class can not be declared as static, Â abstract, Â private, Â protected or final.
- Enum can implement an interface.
- Semi colon declared at the end of enum is optional.
Comparison and Switch case:
- You can compare values of enum using ‘==’ Â operator.
- You can use enum in switch case too.
Example:
create Enum class named Severity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
package org.arpit.java2blog.corejava; public enum Severity { LEVEL_1(1) { @Override public String getDescription() { return "SLA: 2 hours"; } }, LEVEL_2(2) { @Override public String getDescription() { return "SLA: 8 hours"; } }, LEVEL_3(3) { @Override public String getDescription() { return "SLA: 1 day"; } }; int level; Severity(int level) { this.level=level; } public int getLevel() { return level; } public abstract String getDescription(); } |
Create issue.java which will used above enum class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
package org.arpit.java2blog.corejava; public class Issue { String issueName; Severity issueLevel; public Issue(String issueName,Severity level) { super(); this.issueName = issueName; this.issueLevel=level; } public String getIssueName() { return issueName; } public void setIssueName(String issueName) { this.issueName = issueName; } public Severity getIssueLevel() { return issueLevel; } public void setIssueLevel(Severity level) { this.issueLevel = level; } public void resolveIssueUsingSwitch() { switch(issueLevel) { case LEVEL_1: System.out.println("Resolving level 1 issue"); break; case LEVEL_2: System.out.println("Resolving level 2 issue"); break; case LEVEL_3: System.out.println("Resolving level 3 issue"); break; } } public void resolveIssueUsingIfElse() { if(issueLevel==Severity.LEVEL_1) { System.out.println("Resolving level 1 issue"); } else if(issueLevel==Severity.LEVEL_2) { System.out.println("Resolving level 2 issue"); } else if(issueLevel==Severity.LEVEL_3) { System.out.println("Resolving level 3 issue"); } } } |
create enumMain.java to run the code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
package org.arpit.java2blog.corejava; public class EnumMain { public static void main(String args[]) { Issue a=new Issue("Server is down",Severity.LEVEL_1); Issue b=new Issue("UI is not formatted properly",Severity.LEVEL_3); System.out.println("*****************"); System.out.println("Resolving issues:"); // Using if else a.resolveIssueUsingIfElse(); // Using switch case b.resolveIssueUsingSwitch(); System.out.println("*****************"); System.out.println("valueOf method example"); // converting string to Enum using valueOf Method Severity level2=Enum.valueOf(Severity.class,"LEVEL_2"); System.out.println(level2.name()+" -"+level2.getDescription()); // values for getting all enum values System.out.println("*****************"); System.out.println("values method example"); for (Severity level:Severity.values()) { System.out.println(level.name()+" - "+level.getDescription()); } } } |
When you run above code, you will get following output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
***************** Resolving issues: Resolving level 1 issue Resolving level 3 issue ***************** valueOf method example LEVEL_2 -SLA: 8 hours ***************** values method example LEVEL_1 - SLA: 2 hours LEVEL_2 - SLA: 8 hours LEVEL_3 - SLA: 1 day |
Please go through  core java interview questions for more interview questions.