Table of Contents
This is 8 of 16 parts of tutorial series
Tutorial Content: Spring tutorial for beginners
- Introduction to spring framework
- Spring interview questions
- Dependency injection(ioc) in spring
- Spring XML based configuration example
- Spring java based configuaration
- Dependency injection via setter method in spring
- Dependency injection via constructor in spring
- Spring Bean scopes with examples
- Initializing collections in spring
- Beans Autowiring in spring
- Inheritance in Spring
- Spring ApplicationContext
- Spring lifetime callbacks
- BeanPostProcessors in Spring
- Annotation based Configuration in spring
- Spring AOP tutorial
In Spring, bean scope is used to decide which type of bean instance should be return from Spring container back to the caller.
There are 5 types of bean scopes supported in spring
- singleton – Scopes a single bean definition to a single object instance per Spring IoC container.
- prototype – Return a new bean instance each time when requested
- request – Return a single bean instance per HTTP request.
- session – Return a single bean instance per HTTP session.
- globalSession – Return a single bean instance per global HTTP session.
In many cases,spring’s core scopes i.e.singleton and prototype are used.By default scope of beans is singleton.
Here we will see singleton and prototype scopes in more details.
Singleton bean scope
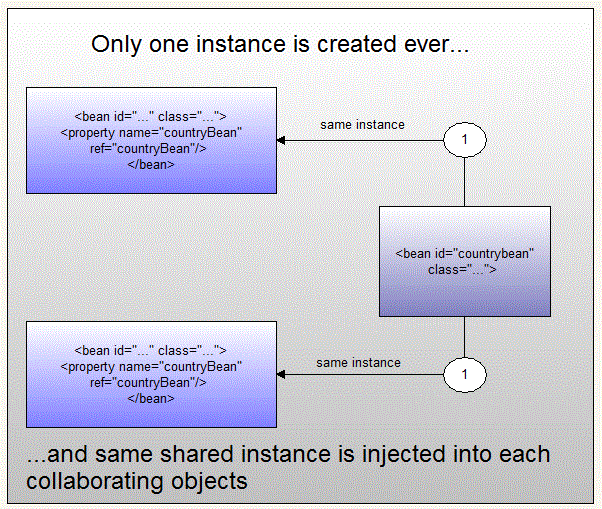
Example:
For configuring spring in your eclipse ide please refer hello world example
1.Country.java:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
package org.arpit.javapostsforlearning; public class Country { String countryName; public String getCountryName() { return countryName; } public void setCountryName(String countryName) { this.countryName = countryName; } } |
2.ApplicationContext.xml
1 2 3 4 5 6 7 8 9 |
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="country" class="org.arpit.javapostsforlearning.Country"> </bean> </beans> |
3.ScopesInSpringMain.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
package org.arpit.javapostsforlearning; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class ScopesInSpringMain{ public static void main(String[] args) { ApplicationContext appContext = new ClassPathXmlApplicationContext("ApplicationContext.xml"); Country countryObj1 = (Country) appContext.getBean("country"); countryObj1.setCountryName("India"); System.out.println("Country Name:"+countryObj1.getCountryName()); //getBean called second time Country countryObj2 = (Country) appContext.getBean("country"); System.out.println("Country Name:"+countryObj2.getCountryName()); } } |
4.Run it
1 2 3 4 |
Country Name:India Country Name:India |
When We firstly called getBean and retrieved country object and set countryName to “india” and when second time we called getBean method it did nothing but returned same object with countryName as “india”.
Prototype bean scope
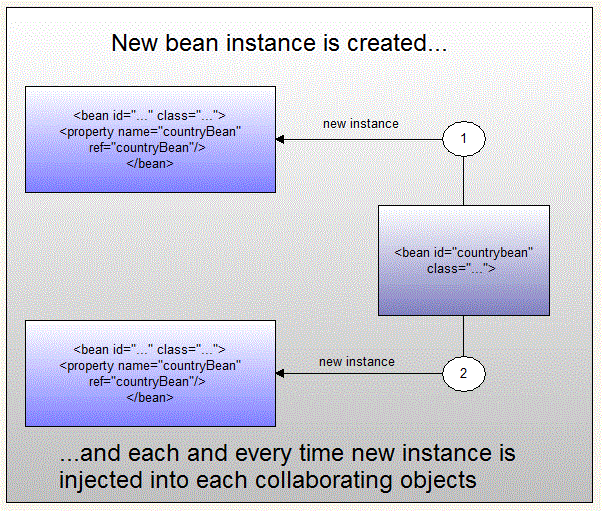
Now we will make change in above xml configuration file.We will add scope attribute in tag and set it to “prototype” and then run it again
ApplicationContext.xml:
1 2 3 4 5 6 7 8 9 10 |
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="country" class="org.arpit.javapostsforlearning.Country" scope="prototype"> </bean> </beans> |
Run it again:
When you will run above application,you will get following as output.
1 2 3 4 |
Country Name:India Country Name:null |
That’s all about Spring bean scope.
In next post,we will see initializing collections in spring.